可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
In XCode 7.3.x ill changed the background Color for my StatusBar with:
func setStatusBarBackgroundColor(color: UIColor) {
guard let statusBar = UIApplication.sharedApplication().valueForKey("statusBarWindow")?.valueForKey("statusBar") as? UIView else {
return
}
statusBar.backgroundColor = color
}
But it seems that this is not working anymore with Swift 3.0.
Ill tried with:
func setStatusBarBackgroundColor(color: UIColor) {
guard let statusBar = (UIApplication.shared.value(forKey: "statusBarWindow") as AnyObject).value(forKey: "statusBar") as? UIView else {
return
}
statusBar.backgroundColor = color
}
But it gives me:
this class is not key value coding-compliant for the key statusBar.
Any Ideas how to change it with XCode8/Swift 3.0?
回答1:
extension UIApplication {
var statusBarView: UIView? {
if responds(to: Selector("statusBar")) {
return value(forKey: "statusBar") as? UIView
}
return nil
}
}
UIApplication.shared.statusBarView?.backgroundColor = .red
回答2:
"Change" status bar background color:
let statusBarView = UIView(frame: UIApplication.shared.statusBarFrame)
let statusBarColor = UIColor(red: 32/255, green: 149/255, blue: 215/255, alpha: 1.0)
statusBarView.backgroundColor = statusBarColor
view.addSubview(statusBarView)
Change status bar text color:
override var preferredStatusBarStyle: UIStatusBarStyle {
return .lightContent
}
Update: please note that the status bar frame will change when the view is rotated. You could update the created subview frame by:
- Using the autoresizing mask:
statusBarView.autoresizingMask = [.flexibleWidth, .flexibleTopMargin]
- Observing
NSNotification.Name.UIApplicationWillChangeStatusBarOrientation
- Or overriding
viewWillLayoutSubviews()
回答3:
With using Swift 3 and 4 you can use the code snippet on below. It finds the view from UIApplication
using valueForKeyPath
as set it's background color.
guard let statusBarView = UIApplication.shared.value(forKeyPath: "statusBarWindow.statusBar") as? UIView else {
return
}
statusBarView.backgroundColor = UIColor.red
Objective-C
UIView *statusBarView = [UIApplication.sharedApplication valueForKeyPath:@"statusBarWindow.statusBar"];
if (statusBarView != nil)
{
statusBarView.backgroundColor = [UIColor redColor];
}
回答4:
For iOS 11 and Xcode 9 use the following steps.
create an extention to UIApplication class:
extension UIApplication {
var statusBarView: UIView? {
return value(forKey: "statusBar") as? UIView
}
}
In your class or wherever you want to change the Status bar's background color:
UIApplication.shared.statusBarView?.backgroundColor = .red
For light content or dark content of status bar simply go to Info.plist and add the following value row with value NO.
View controller-based status bar appearance
- Now just set the light content or whatever you need in the General Tab of your project's settings.
回答5:
Try this
Goto your app info.plist
- Set View controller-based status bar appearance to NO
- Set Status bar style to UIStatusBarStyleLightContent
Then Goto your app delegate and paste the following code where you set your Windows's RootViewController.
#define SYSTEM_VERSION_GREATER_THAN_OR_EQUAL_TO(v) ([[[UIDevice currentDevice] systemVersion] compare:v options:NSNumericSearch] != NSOrderedAscending)
if (SYSTEM_VERSION_GREATER_THAN_OR_EQUAL_TO(@"7.0"))
{
UIView *view=[[UIView alloc] initWithFrame:CGRectMake(0, 0,[UIScreen mainScreen].bounds.size.width, 20)];
view.backgroundColor=[UIColor blackColor];
[self.window.rootViewController.view addSubview:view];
}
回答6:
For Xcode 9 and iOS 11:
The style of the status bar we will try to achieve is a status bar with white content. Go to the ViewController.swift file and add the following lines of code.
override var preferredStatusBarStyle: UIStatusBarStyle {
return .lightContent
}
Or from project settings option you can change status bar's style:
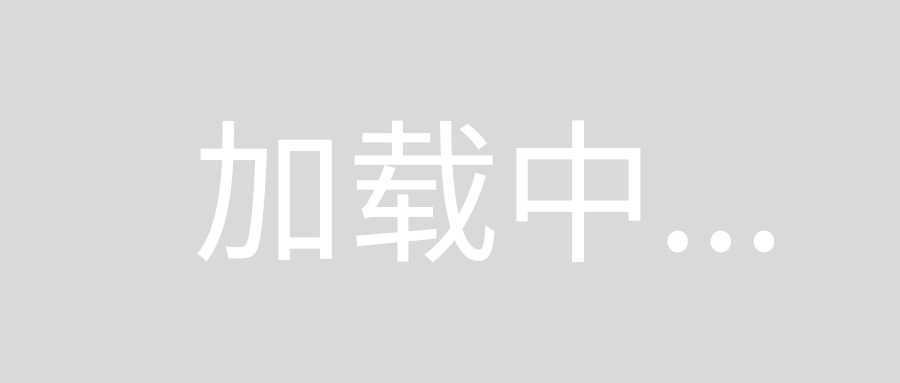
Next, go back to the Storyboard, Select the View Controller and in the Editor menu Select Embed in Navigation Controller. Select the Navigation Bar and in the Attribute Inspector set the Bar Tint color to red. The Storyboard will look like this.
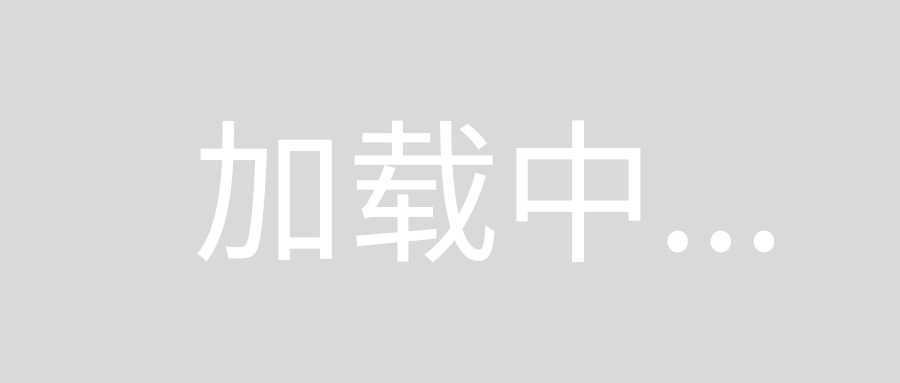
Build and Run the project, The content of the status bar is dark again, which is the default. The reason for this is, iOS asked for the style of the status bar of the navigation controller instead of the contained view controller.
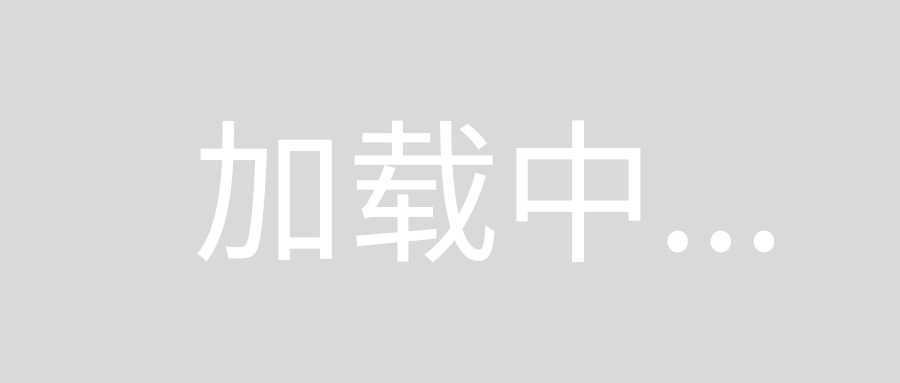
To change the style of all navigation controller inside the app, change the following method in the AppDelegate.swift file.
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
// Override point for customization after application launch.
UINavigationBar.appearance().barStyle = .blackOpaque
return true
}
Build and Run the Project again, this time the content of the status bar changed to white.
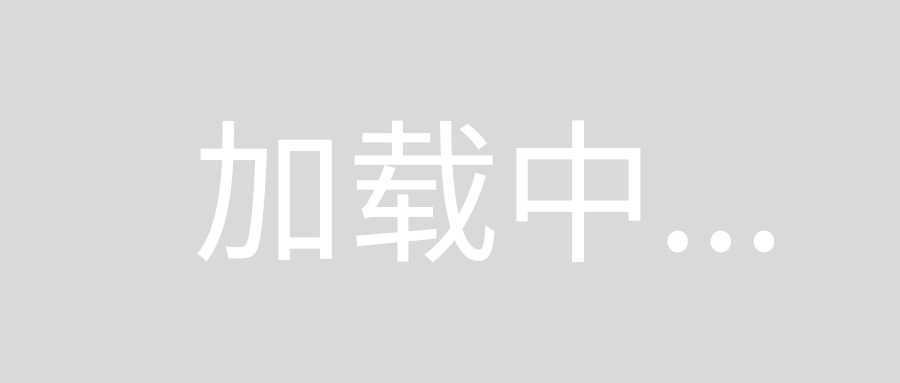
回答7:
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any] ?) -> Bool {
// Override point for customization after application launch.
UINavigationBar.appearance().barStyle = .blackOpaque
return true
}
This works for me, as my navigation barTintColor was black and unable to see the status bar.
When set above code it didFinishLaunch status bar appears in white.
回答8:
You can set background color for status bar during application launch or during viewDidLoad of your view controller.
extension UIApplication {
var statusBarView: UIView? {
return value(forKey: "statusBar") as? UIView
}
}
// Set upon application launch, if you've application based status bar
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
UIApplication.shared.statusBarView?.backgroundColor = UIColor.red
return true
}
}
or
// Set it from your view controller if you've view controller based statusbar
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
UIApplication.shared.statusBarView?.backgroundColor = UIColor.red
}
}
Here is result:
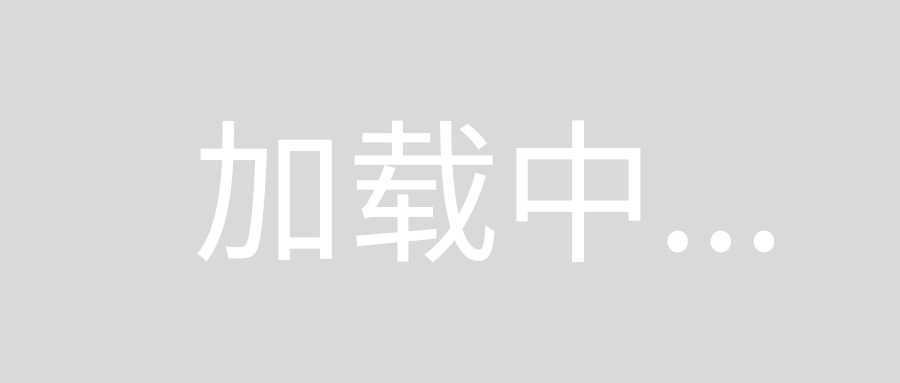