I've been trying to solve this issue for quite some time and cant figure it out. I have the current set up:
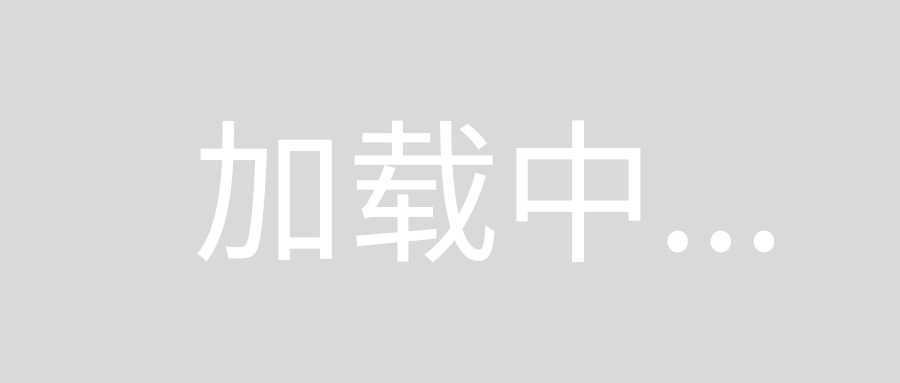
In each view controller I hide the navigation bar like so:
self.navigationController?.setNavigationBarHidden(true, animated: true)
The issue is I loose the swipe gesture on the view controllers that the navigation bar is hidden. I need to have the animation enabled and cannot use:
self.navigationController?.navigationBar.isHidden = true
self.navigationController?.isNavigationBarHidden = true
Any help would be awesome as I'm sure many people has ran into this issue. Thanks!
Here is the answer: Just subclass your NavigationController and do the following.
import UIKit
class YourUINavigationController: UINavigationController {
override func viewDidLoad() {
super.viewDidLoad()
interactivePopGestureRecognizer?.delegate = self
}
}
extension VaultUINavigationController: UIGestureRecognizerDelegate {
func gestureRecognizerShouldBegin(_ gestureRecognizer: UIGestureRecognizer) -> Bool {
return viewControllers.count > 1
}
}
You can handle swipe gesture by doing the following, and its will help you to avoid freezing the app.
- (void)navigationController:(UINavigationController *)navigationController
didShowViewController:(UIViewController *)viewController
animated:(BOOL)animate
{
if ([self.navigationController respondsToSelector:@selector(interactivePopGestureRecognizer)])
{
if (self.navigationController.viewControllers.count > 1)
{
self.navigationController.interactivePopGestureRecognizer.enabled = YES;
}
else
{
self.navigationController.interactivePopGestureRecognizer.enabled = NO;
}
}
}