I have an Angular app which consumes data from a webapi.
When i make a request then i get an typed observable.
now when i do this:
data: Product[];
productService.getByProductId("061000957").subscribe(res => {
console.log(res);
this.data = res;
});
console.log(this.data);
what i see in the console i can see this
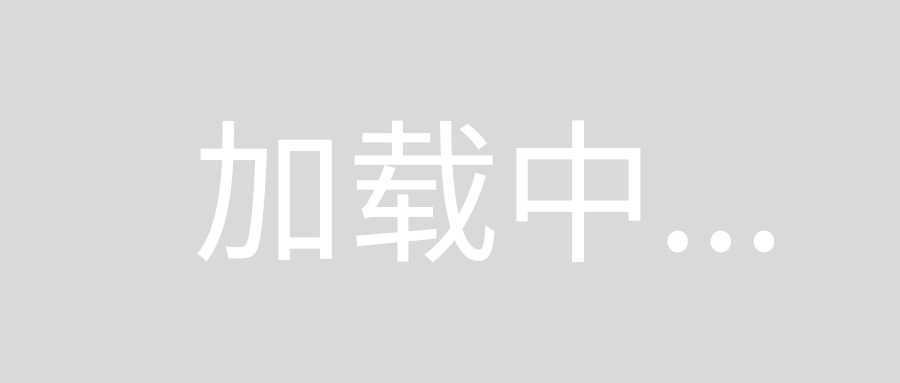
I can clearly see, that the result has the Product i need.
But why cant i save this to my local variable data?
Thanks
Observable is asynchronous just like promise. So console.log(this.data);
is executed before the response is returned . Try .
data: Product[];
productService.getByProductId("061000957").subscribe(res => {
console.log(res);
this.data = res;
console.log(this.data);
});
Now you print your data after it has been set.
You are working with async programming you cannot pause the execution of the code and your subscription will be resolved in future but you cannot predict when. console.log()
outside the subscribe is executed before your subscription is resolved
what you can do is You can store the value in a class property inside subscribe callback .Refer this for better understanding.
data: Product[];
productService.getByProductId("061000957").subscribe(res => {
console.log(res);
this.data = res;
console.log(this.data);
});
You should save the data in the HTTP Observable subscription:
productService.getByProductId("061000957").subscribe(res => {
this.data = res;
console.log(this.data) //you can see
})
You can do it something like this , finally here will execute after getProductId is complete
productService.getByProductId("061000957")
.finally(() => console.log(this.data))
.subscribe(res => {
console.log(res);
this.data = res;
});
I use do and finally when I try to debug rxjs observable