I have a rectangular UIView
object in a static table view cell. I have an outlet to the object and I'm trying to selectively round corners but it will not round corners on the right. Here's what it looks like in the storyboard:
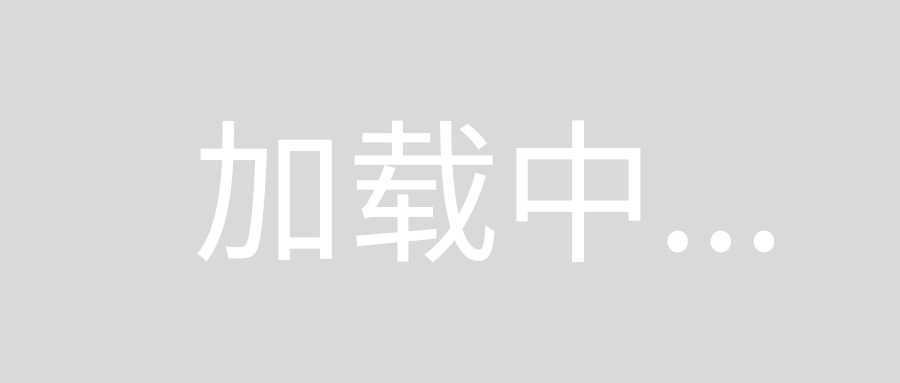
The view I want to configure is the pale yellow one. The red view serves no real purpose other than confirming that the other view is not being clipped.
There are no constraint issues or errors. Here's how it looks on a 4S:
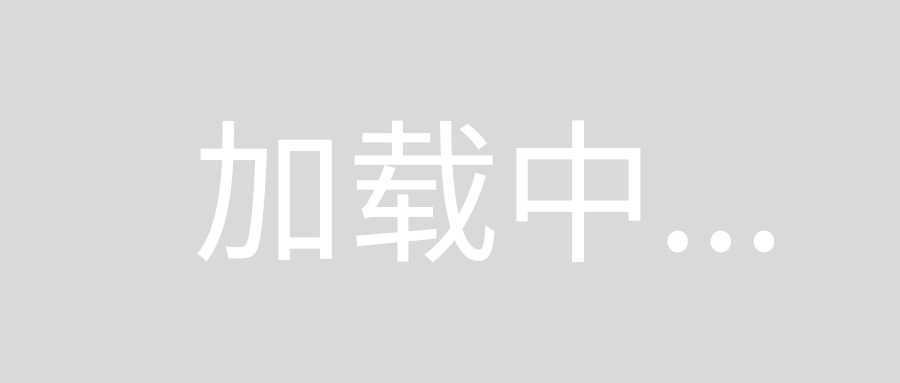
I'm doing the configuration in tableView:willDisplayCell:forRowAtIndexPath:
With this code (myView
is the outlet to the pale yellow view above):
self.myView.layer.cornerRadius = 5.0f;
self.myView.clipsToBounds = YES;
I get this result:
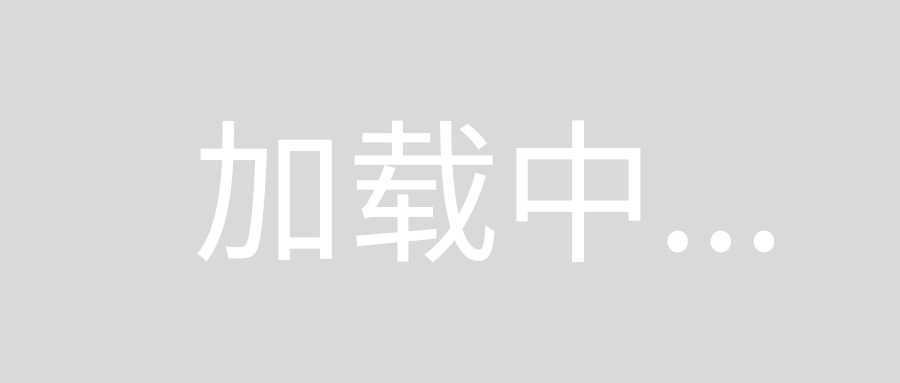
All 4 corners rounded. But I need to selectively round corners, normally both top or both bottom corners. Here is the code for rounding the two bottom corners:
CAShapeLayer *shape = [[CAShapeLayer alloc] init];
shape.path = [UIBezierPath bezierPathWithRoundedRect:CGRectMake(0, 0, self.myView.bounds.size.width, self.myView.bounds.size.height)
byRoundingCorners:UIRectCornerBottomLeft | UIRectCornerBottomRight
cornerRadii:CGSizeMake(5.0f, 5.0f)].CGPath;
self.myView.layer.mask = shape;
self.myView.layer.masksToBounds = YES;
And here is the result:
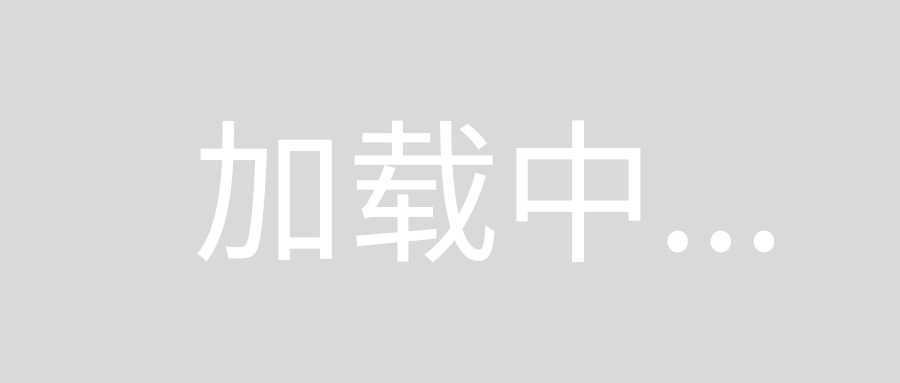
It doesn't work for either of the right-hand corners. It works fine for either of the left-hand corners.
I tested the bezier code with a view object on an ordinary view controller (outside of a table view) and the code works as expected--I can round any corner selectively.
Any ideas why it's not working in a table view cell?
How can I accomplish this without resorting to drawing code?
I think it happens because of your view's frame and therefore bounds get changed after you make a layer mask.
As documentation for tableView:willDisplayCell:forRowAtIndexPath: states: After the delegate returns, the table view sets only the alpha and frame properties, and then only when animating rows as they slide in or out.
The best tactics would be subclassing UITableViewCell and perform layer adjustments in layoutSubviews.
Give a try with this snippet:
CAShapeLayer *shape = [CAShapeLayer layer];
// Create the path (with only the top-left corner rounded)
UIBezierPath *maskPath = [UIBezierPath bezierPathWithRoundedRect:self.myView.bounds.bounds
byRoundingCorners:byRoundingCorners:UIRectCornerBottomLeft|UIRectCornerBottomRight
cornerRadii:CGSizeMake(5.0f, 5.0f)];
shape.frame = self.myView.bounds;
shape.path = maskPath.CGPath;
// Set the newly created shape layer as the mask for the image view's layer
self.myView.layer.mask = shape;
Layer masks will not render when used in combination with the CALayer renderInContext method. If you need to use this try rounding corners with the following: Just two rounded corners?.
Add [self.myView layoutIfNeeded];
Example:
[self.myView layoutIfNeeded];
CAShapeLayer *shape = [[CAShapeLayer alloc] init];
shape.path = [UIBezierPath bezierPathWithRoundedRect:CGRectMake(0, 0, self.myView.bounds.size.width, self.myView.bounds.size.height)
byRoundingCorners:UIRectCornerBottomLeft | UIRectCornerBottomRight
cornerRadii:CGSizeMake(5.0f, 5.0f)].CGPath;
self.myView.layer.mask = shape;
self.myView.layer.masksToBounds = YES;
Try adding this
shape.path = [UIBezierPath bezierPathWithRoundedRect:self.myView.bounds
byRoundingCorners:UIRectCornerBottomRight
cornerRadii:CGSizeMake(5.0f, 5.0f)].CGPath;
and this
shape.frame = self.myView.bounds; //Right before setting your self.mView.layer.mask = shape;