I have developed a simple windows Application(MDI) in C# which exports the data from SQL to Excel.
I am using ClosedXML to achieve this successfully.
When the process is executed, I want to show a picturebox containing a animated GIF image.
I am a beginner and don't know how to achieve this, the picturebox appears after the process is completed.
I saw lot of posts which says to use backgroundworker or threading which I have never used and finding it hard to implement.
Can I have an step by step example with explanation.
The two functions which I have created which I call before and after I execute the code.
private void Loading_On()
{
Cursor.Current = Cursors.WaitCursor;
pictureBox2.Visible = true;
groupBox1.Enabled = false;
groupBox5.Enabled = false;
groupBox6.Enabled = false;
Cursor.Current = Cursors.Arrow;
}
private void Loading_Off()
{
Cursor.Current = Cursors.Arrow;
pictureBox2.Visible = false;
groupBox1.Enabled = true;
groupBox5.Enabled = true;
groupBox6.Enabled = true;
Cursor.Current = Cursors.WaitCursor;
}
It is not that hard to add a BackgroundWorker
- Open your form in the designer
- Open the Toolbox (ctrl+alt+X)
- Open the category Components
- Drag the Backgroundworker on your From
You will end-up with something like this:
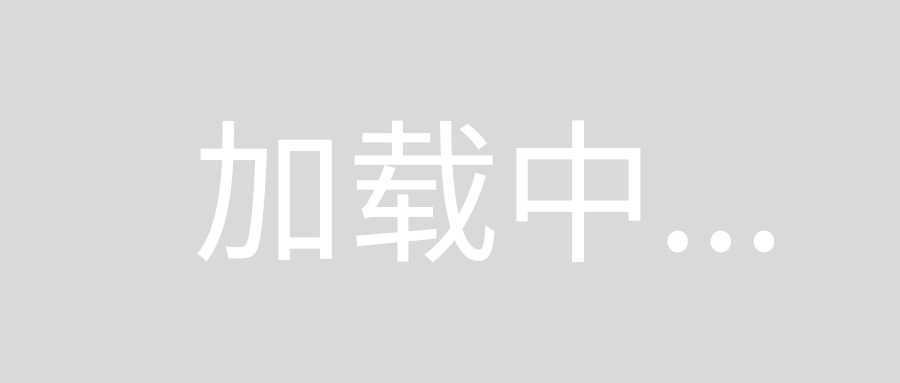
You can now switch to the event view on the Properties tab and add the events for DoWork and RunWorkerCompleted
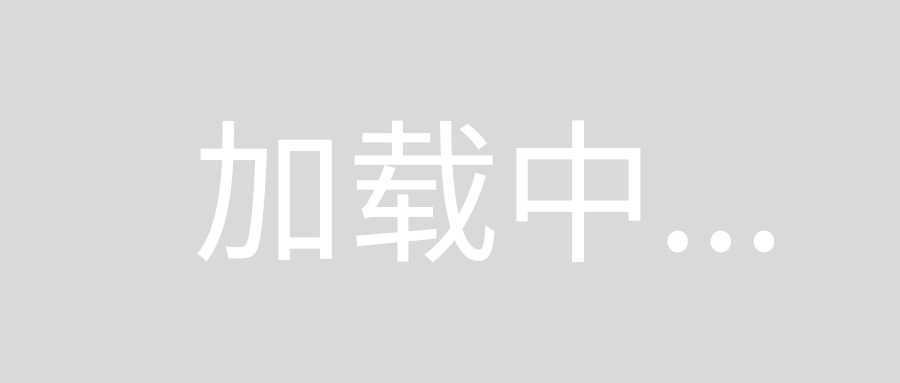
The following code goes in these events, notice how DoWork
use the DowWorkEventArgs
Argument property to retrieve the value that is supplied in RunWorkerAsync
.
private void backgroundWorker1_DoWork(object sender, DoWorkEventArgs e)
{
// start doing what ever needs to be done
// get the argument from the EventArgs
string comboboxValue = (string) e.Argument; // if Argument isn't string, this breaks
// remember that this is NOT on the UI thread
// do a lot of work here that takes forever
System.Threading.Thread.Sleep(10000);
// afer this the completed event is fired
}
private void backgroundWorker1_RunWorkerCompleted(object sender, RunWorkerCompletedEventArgs e)
{
// this runs on the UI thread
Loading_Off();
}
Now you only need to start the background job, for example from a button click event to call RunWorkerAsync
private void button1_Click(object sender, EventArgs e)
{
Loading_On();
backgroundWorker1.RunWorkerAsync(comboBox1.SelectedItem); // pass a string here
}
Done! You have successfully added a backgroundworker to your form.
The best way to achieve that is running the animation in a async task, but accordingly some limitations is it possible to do that on windows forms using a Thread Sleep.
eg: In your constructor,
public partial class MainMenu : Form
{
private SplashScreen splash = new SplashScreen();
public MainMenu ()
{
InitializeComponent();
Task.Factory.StartNew(() => {
splash.ShowDialog();
});
Thread.Sleep(2000);
}
It is very important to put the Thread Sleep after have started a new one, don't forget that every action you did on this thread you need ot invoke, for example
void CloseSplash(EventArgs e)
{
Invoke(new MethodInvoker(() =>
{
splash.Close();
}));
}
Now your gif should work!