Dearest Stackoverflowers,
I was wondering if anyone knows how to solve this the best way;
I'm talking to an api which returns a json object like this:
{
"field1": "value1",
"field2": "value2",
"details": {
"nested1": 1,
"nested2": 1
}
In java I have an object (entity) which for example, would have all these fields, but with the details as loose fields, so:
field1, field2, nested1, nested2.
This because It's an android project and I can't just go saving a class with info into my entity since I'm bound to ormlite.
Is there any way to convert the fields flat into my object using GSON? note that I'm using a generic class to convert these right now straight from the API. And I want to store these fields (which contain information as an int). In the same entity.
You can write a custom type adapter to map json value to your pojo.
Define a pojo:
public class DataHolder {
public List<String> fieldList;
public List<Integer> detailList;
}
Write a custom typeAdapter:
public class CustomTypeAdapter extends TypeAdapter<DataHolder> {
public DataHolder read(JsonReader in) throws IOException {
final DataHolder dataHolder = new DataHolder();
in.beginObject();
while (in.hasNext()) {
String name = in.nextName();
if (name.startsWith("field")) {
if (dataHolder.fieldList == null) {
dataHolder.fieldList = new ArrayList<String>();
}
dataHolder.fieldList.add(in.nextString());
} else if (name.equals("details")) {
in.beginObject();
dataHolder.detailList = new ArrayList<Integer>();
} else if (name.startsWith("nested")) {
dataHolder.detailList.add(in.nextInt());
}
}
if(dataHolder.detailList != null) {
in.endObject();
}
in.endObject();
return dataHolder;
}
public void write(JsonWriter writer, DataHolder value) throws IOException {
throw new RuntimeException("CustomTypeAdapter's write method not implemented!");
}
}
Test:
String json = "{\"field1\":\"value1\",\"field2\":\"value2\",\"details\":{\"nested1\":1,\"nested2\":1}}";
GsonBuilder builder = new GsonBuilder();
builder.registerTypeAdapter(DataHolder.class, new CustomTypeAdapter());
Gson gson = builder.create();
DataHolder dataHolder = gson.fromJson(json, DataHolder.class);
Output:
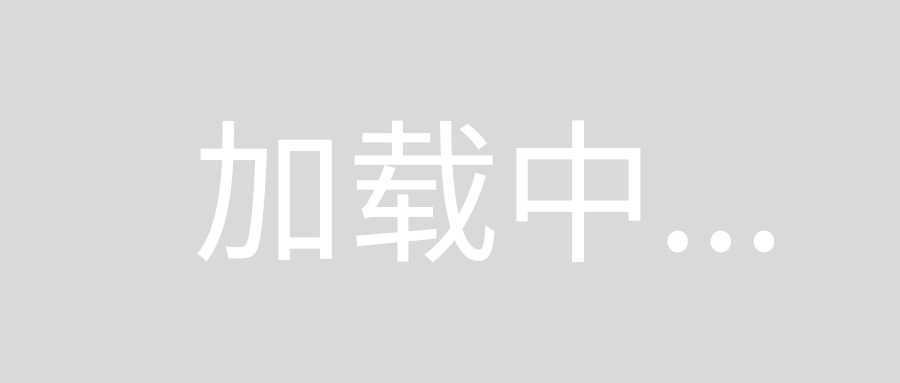
About TypeAdapter:
https://google-gson.googlecode.com/svn/trunk/gson/docs/javadocs/com/google/gson/TypeAdapter.html
http://www.javacreed.com/gson-typeadapter-example/