I want to have a JFrame, where on the left and the right there is a border that has the color black and a width of withfOfJFrame/10.
Now, my try at it looks like this:
JFrame f = new JFrame();
f.setSize(800, 600);
f.setLayout(new BorderLayout());
JPanel leftBorder = new JPanel();
JPanel rightBorder = new JPanel();
leftBorder.setBackground(Color.black);
rightBorder.setBackground(Color.black);
leftBorder.setSize(f.getWidth()/10, f.getHeight());
rightBorder.setSize(f.getWidth()/10, f.getHeight());
JPanel center = new JPanel();
center.setBackground(Color.red);
f.add(leftBorder, BorderLayout.WEST);
f.add(center, BorderLayout.CENTER);
f.add(rightBorder, BorderLayout.EAST);
f.setVisible(true);
This adds a black border on the left and the right, but that border has a fixed size and doesn't recalculate when resizing the window. The size isn't even 1/10 of 800 (the beginning width of the JFrame).
What am I doing wrong? Or is there even a better way to do this?
You may achieve the desired result with a GridBagLayout
and appropriate weights:
public class Snippet {
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
JFrame f = new JFrame();
f.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
JPanel leftBorder = new JPanel();
JPanel rightBorder = new JPanel();
leftBorder.setBackground(Color.black);
rightBorder.setBackground(Color.black);
JPanel center = new JPanel();
center.setBackground(Color.red);
f.setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.fill = GridBagConstraints.BOTH;
gbc.weighty = 1.0;
gbc.gridy = 0;
gbc.gridwidth = 1;
gbc.gridheight = 1;
gbc.gridx = 0;
gbc.weightx = 0.1;
f.add(leftBorder, gbc);
gbc.gridx = 1;
gbc.weightx = 0.8;
f.add(center, gbc);
gbc.gridx = 2;
gbc.weightx = 0.1;
f.add(rightBorder, gbc);
f.pack();
f.setVisible(true);
}
});
}
}
A ComponentListener
works well for this.
Addendum: The Flank
panel needs to revalidate()
itself explicitly in response to any size change.
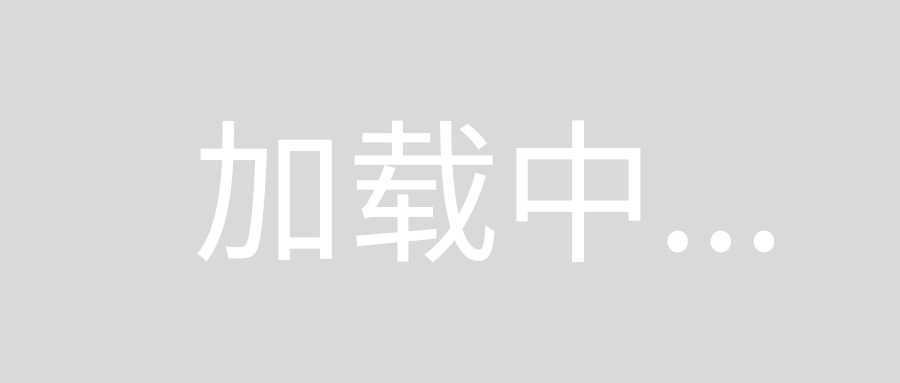
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.event.ComponentAdapter;
import java.awt.event.ComponentEvent;
import javax.swing.JFrame;
import javax.swing.JPanel;
/** @see http://stackoverflow.com/a/14588506/230513 */
public class Test {
private static final int W = 320;
private static final Center center = new Center();
private static class Center extends JPanel {
public Center() {
setBackground(Color.red);
}
@Override
public Dimension getPreferredSize() {
return new Dimension(W, 3 * W / 4);
}
};
private static class Flank extends JPanel {
private Dimension d = new Dimension(W / 10, 0);
public Flank() {
setBackground(Color.black);
Test.center.addComponentListener(new ComponentAdapter() {
@Override
public void componentResized(ComponentEvent e) {
d = new Dimension(e.getComponent().getWidth() / 10, 0);
revalidate();
}
});
}
@Override
public Dimension getPreferredSize() {
return d;
}
}
private void display() {
JFrame f = new JFrame("Test");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.add(new Flank(), BorderLayout.WEST);
f.add(center, BorderLayout.CENTER);
f.add(new Flank(), BorderLayout.EAST);
f.pack();
f.setLocationRelativeTo(null);
f.setVisible(true);
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
new Test().display();
}
});
}
}