I have this page admin_inquiry.php(page1) which has a dynamic table that shows rows of records.I want to get the values from 2 columns, ContactNo, and message. The contactno column contains a link, which goes to admin_sms.php(page2) and displays the contact no in a textfield.
page1:
<td><a href="admin_sms.php?ContactNo=<?php echo $row_ContactUs['ContactNo']; ?>">Send SMS</a></td>
page2:
<input name='number' type='text' id="number" value="<?php if(isset($_GET['ContactNo'])){echo $_GET['ContactNo'];}else{echo "";}?>">
I also want to get the content of column message from the 1st page and show it in a textarea in page 2. But it should show the message the belongs to particular id or something. I heard of sessions but I don't quite get it yet. Could you show me how?
UPDATE
I tried this in the admin_inquiry.php
$_SESSION['message'] = $row_ContactUs['message'];
admin_sms.php
$_SESSION['message'];
<textarea name="frmMsg" id="frmMsg" cols="45" rows="5"><?php echo $_SESSION['message'];?></textarea>
The problem is, it's showing the same message. Not the corresponding message that belongs to a certain id or something.Help me please.
If you are still having problems solving this, in your table include a html form
UPDATE including pagination
first solution, create a file and call it contact.php
<?php
$db = new PDO('mysql:host=localhost; dbname=data','root','');
$contacts = $db->query('SELECT * FROM contactdetails');
//creating pagination
$nav_counter = basename($_SERVER['SCRIPT_FILENAME']); //getting name of the current page
$current_page = $nav_counter;
$nav_counter = rtrim($nav_counter, ".php"); //getting the name of the current page and removing the extension
//creating pagination pages
$next_page = $nav_counter + 1;
$prev_page = $nav_counter - 1;
//getting row count, we are going to use it to limit our query and to create pagination
$row_count = $contacts->rowCount();
//number of records to show per page
$num_records = 5;
//getting the last page
$last_page = ($row_count / $num_records) - 1;
if(!is_int($last_page)){
$last_page = (int)$last_page + 1;
}
//displaying records
$start = 0;
$limit = $num_records; //number of records to show
if ($current_page !== 'admin_inquiry.php'){
$start = ($limit * $nav_counter);
}
//getting number of rows left in the table
$rows_left = $db->query("SELECT * FROM contactdetails ORDER BY ID limit $start,$limit");
$num_rows = $rows_left->rowCount();
//if records left in the table is less than the number of records to show $num_records
if ($num_rows < $num_records) {
$limit = $num_rows; //limit is equal to the number of records left in the table
}
//getting number of records from the table
$contacts = $db->query("SELECT * FROM contactdetails ORDER BY ID limit $start,$limit");
//displaying pagination and creating pages if they don't exists
$pages = array();
for ($counter = 1; $counter <= $last_page; $counter++) {
$pages[] = $counter;
}
//storing pages in array and creating a page if it doesn't exist
foreach ($pages as $num) {
$page = $num.'.php';
if(file_exists($page)== false && $num <= $last_page){
copy('admin_inquiry.php', $page);
}
}
?>
create a pagination.php file
<p class="pagenav"><a href="<?php if($current_page == '1.php'){ echo 'admin_inquiry.php';}else{echo $prev_page.'.php';} ?>" <?php if($current_page == 'admin_inquiry.php'){echo 'class="hide"';} ?>><</a> <a href="<?php echo $next_page.'.php'; ?>" <?php if($next_page > $last_page){echo 'class="hide"';} ?>>></a></p>
create a css file admin.css
table {
width: 100%;
margin: 0 auto;
}
table, td, th {
border: 1px solid black;
}
th {
height: 50px;
}
#div{
width: 40%;
margin: 0 auto;
}
.pagenav a{
display: inline;
width: 100px;
padding: 10px;
height: 100px;
border-radius: 50px;
font-size: 20px;
color: #fff;
line-height: 50px;
text-align: center;
text-decoration: none;
background-color: #0186ba;
}
.pagenav a:hover{
background-color: #fff;
border: 1px solid #000;
color: #000;
}
.hide {
display: none !important;
}
p{
text-align: center;
}
admin_iquiry.php file
<?php
require_once('contact.php');
?>
<!DOCTYPE html>
<html>
<head>
<title></title>
<link rel="stylesheet" type="text/css" href="admin.css">
</head>
<body>
<div id="div">
<table>
<tr>
<th>ID</th>
<th>Contact No.</th>
<th>Message</th>
</tr>
<?php foreach ($contacts as $value) : ?>
<tr><td><?php echo $value['ID']; ?></td><td><?php echo $value['ContactNO']; ?></td><td><?php echo $value['Message'] ?></td><td><form action="admin_sms.php" method="post"><input type="hidden" name="ContactNo" value="<?php echo $value['ContactNO']; ?>"><input type="hidden" name="Message" value="<?php echo $value['Message']; ?>"><input type="Submit" value="Send SMS" name="send_sms" /></form></td></tr>
<?php endforeach; ?>
</table>
<?php require_once('pagination.php'); ?>
</div>
</body>
</html>
admin_sms.php
<?php
if(isset($_POST['send_sms'])){
$contactNo = $_POST['ContactNo'];
$message = $_POST['Message'];
}
?>
html code
<div style="margin:0 auto; width:50%">
<p>Message for: <?php echo $contactNo; ?></p>
<textarea><?php echo "$message"; ?></textarea>
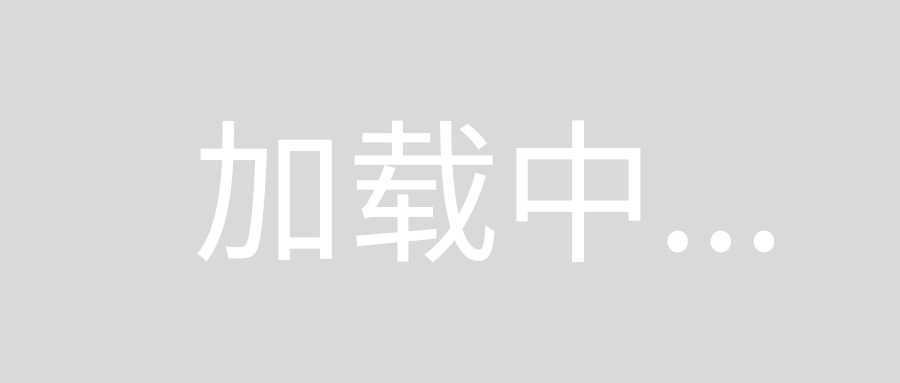
Second solution using $_GET
<?php
require_once('contact.php');
?>
<!DOCTYPE html>
<html>
<head>
<title></title>
<link rel="stylesheet" type="text/css" href="admin.css">
</head>
<body>
<div id="div">
<table>
<tr>
<th>ID</th>
<th>Contact No.</th>
<th>Message</th>
</tr>
foreach ($contacts as $value) : ?>
<tr><td><?php echo $value['ID']; ?></td>
<td><?php echo $value['ContactNO']; ?></td>
<td><?php echo $value['Message'] ?></td>
//use id of the contact no and in the next page use that id to query data related to that id
<td><a href="admin_sms.php?id=<?php echo $value['ID']; ?>">Send Sms</a></td></tr>
<?php endforeach; ?>
</table>
<?php require_once('pagination.php'); ?>
</div>
</body>
</html>
admin_sms.php
<?php
$db = new PDO('mysql:host=localhost; dbname=data','root','');
if(isset($_GET['id'])){
$id= $_GET['id'];
$results = $db->query("SELECT * FROM contactdetails WHERE ID = $id");
foreach ($results as $value) {
$contactNo = $value['ContactNO'];
$message = $value['Message'];
}
}
?>
in your html
<div style="margin:0 auto; width:50%">
<p>Message for: <?php echo $contactNo; ?></p>
<textarea><?php echo "$message"; ?></textarea>
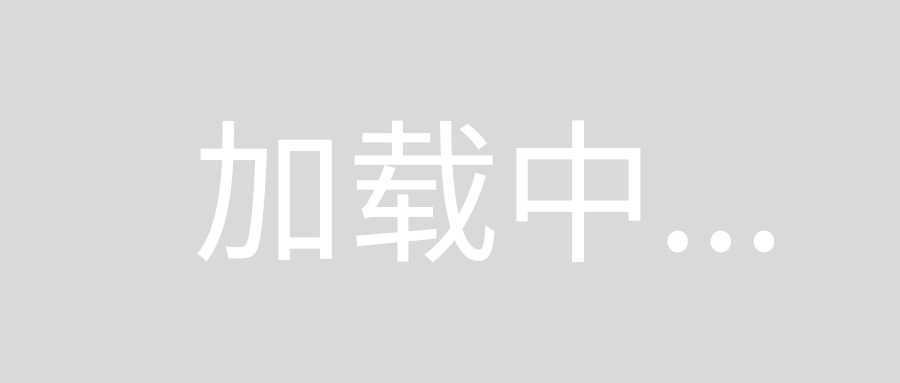
Approach 1 :
Use the POST
method to submit the form. That way, you can query the Message element, pertaining the Contact No., depending on what name or Id you have given to the <td>
elements. (you have not given any yet).
Approach 2 :
Set the href property of the contact No. element dynamically, using, say jQuery
. Set the action attribute to a URL that includes both the ContactNo and Message as URL parameters
. That way you can access the message also from the GET
array just as you are doing with the Contact No. (Note : Not sure if long texts like message can be passed on as URL parameters, depends on the length, and may be security issues).