In a Swift 4 Playground this code:
let time = 1234
let description: String? = nil
let keyed: [String : Any?] = [
"time": time,
"description": description
]
let filtered: [String : String] = keyed
.filter{ _, value in value != nil }
.mapValues { value in return String(describing: value!) }
print(keyed)
print(filtered)
Produces this output:
["description": nil, "time": Optional(1234)]
["time": "1234"]
Which is exactly what I want (only key-value pairs where the original value is not nil
, with the value unwrapped and converted to a string). However in Xcode 9 (beta 3) my build fails with 'filter' is unavailable
. Is this a beta ¯\_(ツ)_/¯
kind of thing, or am I missing something?
You are using Swift 3.2 instead of Swift 4.
You can change it under your target's Build Options
> Swift Compiler - Language
> Swift Language Version
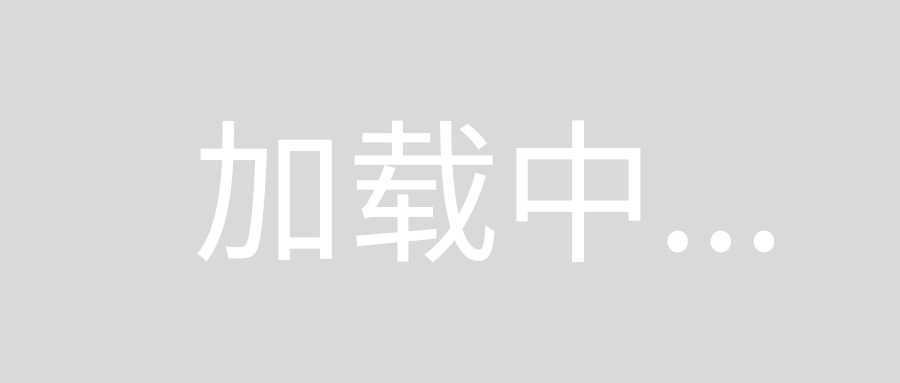
Excerpt from Foundation's docs:
https://developer.apple.com/documentation/swift/dictionary/2903389-filter?changes=latest_minor
func filter(_ isIncluded: (Dictionary.Element) throws -> Bool) rethrows -> [Dictionary.Key : Dictionary.Value]
Xcode 9.0+
I tried to resolve this a few ways, including rebooting Xcode, clearing DerivedData
etc., but at the time nothing worked. I came back to the project several days later and found that the same code which had previously failed to build now built without issue (without my having made any relevant changes). So I'm blaming this on a quirk of the Xcode 9 beta. Or perhaps something was just gummed up somewhere and Xcode eventually cleared a cache or something of that nature. ¯\_(ツ)_/¯
I had the same issue today.
My project that was created in Swift 3.x with xCode 8.x. After the upgrade to xCode 9 it was working fine until today when the 'filter is unavailable'
error kicked in.
Setting the Language Version explicitly to Swift 4 didn't solve it. (Neither did cleaning the project, relaunching xCode etc.)
The trick -that worked for me- was to convert to whole project to Swift 4.
(I used started with the migration tool at Edit/Convert/To current Swift syntax, which wasn't too helpful in may case but that's a completely different matter.)
After that the error was gone...