I have been reading couple of questions here from different newbies about Login and validation in codeigniter, some have mixed JavaScript and J-query. Codeigniter itself provide a robust form validation along with custom error setting support. I have decided to share with you the easiest way to kickstart in codeigniter with login boilerplate which I have created and I am sharing it with you. It has
Controller
Login
Model: Login_model
Views: Login and success
And basic configurations
Step-1
Download Codeigniter 3.x from Official Website
Step-2
Extract in a folder in your localhost root. (htdocs in xampp and www in wamp)
Step-3. Configuration
Open the folder you have extracted the codeigniter in go to
application->config->autoload.php. Go to line 55 and autoload these two libraries
$autoload['libraries'] = array('database', 'session');
Go to line 67 and load two helpers
$autoload['helper'] = array('url', 'file');
Save the file and now go the application->config->config.php
Set Base URL on line 19 as
$config['base_url'] = 'http://'.$_SERVER['SERVER_NAME'].'/folder_name/';
On line 31 remove the index.php from value and change it to
$config['index_page'] = '';
on line 49 set uri_protocol from AUTO to REQUEST_URI
$config['uri_protocol'] = 'REQUEST_URI';
on line 229 set an encryption key
$config['encryption_key'] = '!@#$%^&*()ASDFGHJKL:ZXCVBNM<>QWERTYUIOP';
// I recommend you create a hash and place it here
Save the file
Step-4 .htaccess
On the root of codeigniter installation folder create an .htaccess file write following in it and save
<IfModule mod_rewrite.c>
RewriteEngine On
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule ^(.*)$ index.php/$1 [L]
</IfModule>
Step-5. Create Database
Open your phpmyadmin or mysql terminal create a database , create a table users in it , you can use following query
CREATE TABLE `users` (
`id` int(11) NOT NULL,
`username` varchar(50) NOT NULL,
`password` varchar(50) NOT NULL,
`fullname` varchar(50) NOT NULL,
`status` enum('pending','approved') NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Step -6. Connecting Database to Codeigniter
Go to application->config->database.php. Assuming its a fresh install and you haven't created any environments. Go to line 52 and change the four lines to
$db['default']['username'] = 'username'; // will be root if you have xampp
$db['default']['password'] = 'password'; // will be empty if you haven't set
$db['default']['database'] = 'your_database_name';
$db['default']['dbdriver'] = 'mysqli'; // changed from mysql to mysqli
Step-7. The View
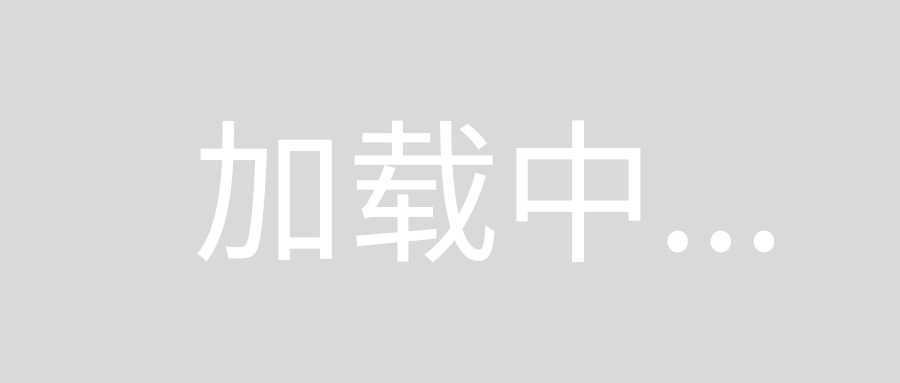
Source Code
Step-8. The Controller
class Login extends CI_Controller {
function __construct()
{
parent::__construct();
$this->load->model('Login_model');
$this->load->library('form_validation');
}
public function index()
{
if($this->isLoggedin()){ redirect(base_url().'login/dashboard');}
$data['title']='Login Boiler Plate';
if($_POST)
{
$config=array(
array(
'field' => 'username',
'label' => 'Username',
'rules' => 'trim|required'
),
array(
'field' => 'password',
'label' => 'Password',
'rules' => 'trim|required'
)
);
$this->form_validation->set_rules($config);
if ($this->form_validation->run() == false) {
// if validation has errors, save those errors in variable and send it to view
$data['errors'] = validation_errors();
$this->load->view('login',$data);
} else {
// if validation passes, check for user credentials from database
$user = $this->Login_model->checkUser($_POST);
if ($user) {
// if an record of user is returned from model, save it in session and send user to dashboard
$this->session->set_userdata($user);
redirect(base_url() . 'Login/dashboard');
} else {
// if nothing returns from model , show an error
$data['errors'] = 'Sorry! The credentials you have provided are not correct';
$this->load->view('login',$data);
}
}
}
else
{
$this->load->view('login',$data);
}
}
public function change_password()
{
if($this->isLoggedin()){
$data['title']='Change Password';
if($_POST)
{
$config=array(
array(
'field' => 'old_password',
'label' => 'Old Password',
'rules' => 'trim|required|callback_checkPassword'
),
array(
'field' => 'password',
'label' => 'Password',
'rules' => 'trim|required'
),
array(
'field' => 'conf_password',
'label' => 'Confirm Password',
'rules' => 'trim|required|matches[password]'
)
);
$this->form_validation->set_rules($config);
if ($this->form_validation->run() == false)
{
// if validation has errors, save those errors in variable and send it to view
$data['errors'] = validation_errors();
$this->load->view('change_password',$data);
}
else
{
// if validation passes, check for user credentials from database
$this->Login_model->updatePassword($_POST['password'],$this->session->userdata['id']);
$this->session->set_flashdata('log_success','Congratulations! Password Changed');
redirect(base_url() . 'Login/dashboard');
}
}
else
{
$this->load->view('change_password',$data);
}
}
else
{
redirect(base_url().'Login');
}
}
public function dashboard()
{
if($this->isLoggedin())
{
$data['title']='Welcome! You are logged in';
$this->load->view('success',$data);
}
else
{
redirect(base_url().'Login');
}
}
public function logout()
{
$this->session->sess_destroy();
redirect(base_url().'Login');
}
public function isLoggedin()
{
if(!empty($this->session->userdata['id']))
{
return true;
}
else
{
return false;
}
}
}
Step-8. The Model
class Login_model extends CI_Model{
function __construct(){
parent::__construct();
}
public function checkUser($data)
{
$st=$this->db->SELECT('*')->from('users')
->WHERE('username',$data['username'])
->WHERE('password',sha1(md5($data['password'])))
->get()->result_array();
if(count($st)>0)
{
return $st[0];
}
else
{
return false;
}
}
public function checkPassword($str)
{
$st=$this->db->SELECT('*')->from('users')
->WHERE('id',$this->session->userdata['id'])
->WHERE('password',sha1(md5($str)))
->get()->result_array();
if(count($st)>0)
{
return true;
}
else
{
return false;
}
}
public function updatePassword($password,$id)
{
$pass=array(
'password' => sha1(md5($password))
);
$this->db->WHERE('id',$id)->update('users',$pass);
}
}
Step-9. Testing
Open Database in Phpmyadmin and insert sample data in to your table using following query
INSERT INTO `users` (`id`, `username`, `password`, `fullname`, `status`)
VALUES
(1, 'john', '56f5950b728849d0b97c1bccf1691c090ab6734c', 'John Vick',
'approved');
Test-1
Empty Submit
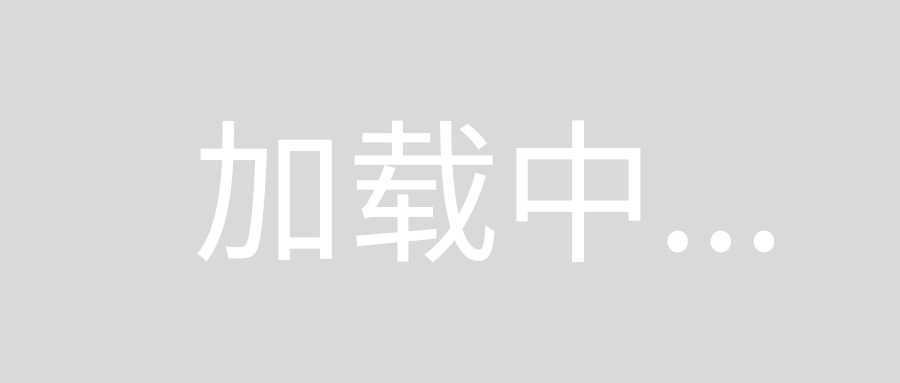
It will produce the error, which we are storing in an errors index in Controller, passing it to view and displaying it in the view if the value exists.
Test-2. Wrong Credentials
Provide any username and password (random)
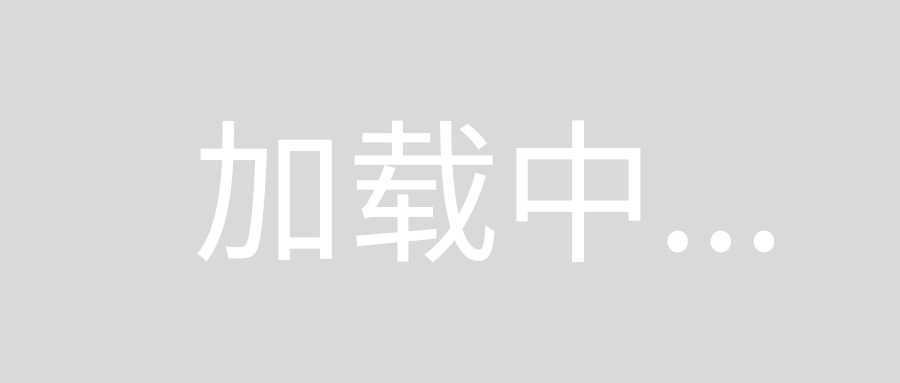
Test-3. Correct Credentials
Username: john
Password: john
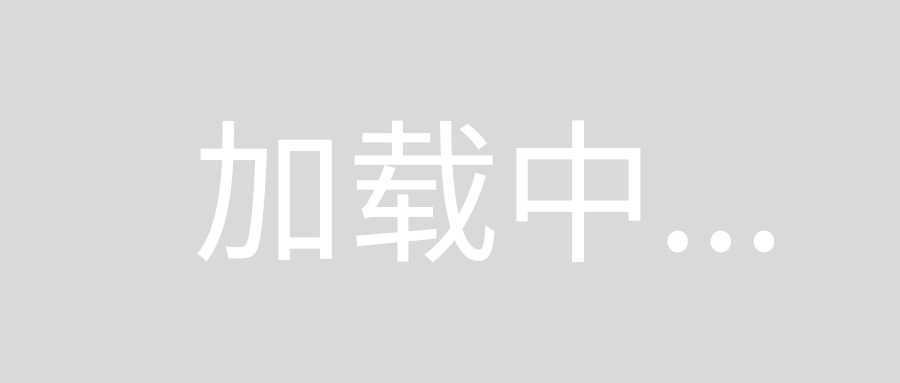
Success View Source Code
ALERTS!!
This is a basic code for kick starters and there is a lot more room for improvements like Security Features and Encryption
Complete Source Code
You can download the complete source code from Git at Boiler-Plates-Codeigniter-3.x-Login