I answered a question on how to set up a Chart
to look like a regular mathematical graph, i.e. with the axes centered and with nice arrows to the top and to the right..
However I found the built-in AxisArrowStyle.Triangle to be rather big and found no way to make it smaller.
Lines - A line-shaped arrow is used for the relevant axis.
None - No arrow is used for the relevant axis.
SharpTriangle - A sharp triangular arrow is used for the relevant axis.
Triangle - A triangular arrow is used for the relevant axis.
Here is the original look of it:
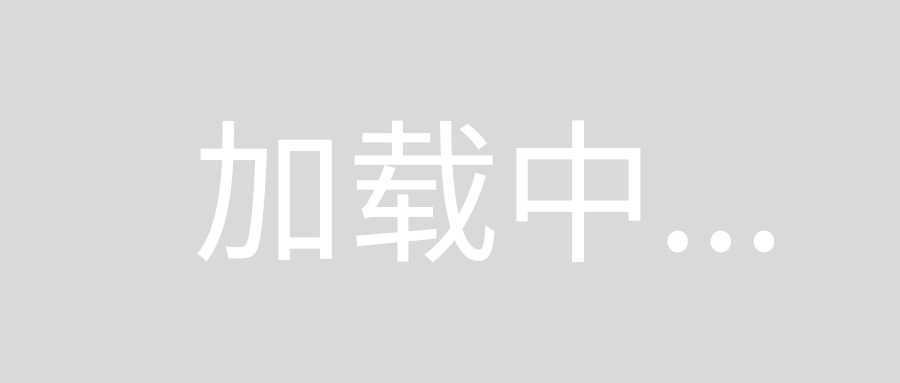
So how can we fix this?
The Chart's axis.AxisArrowStyle
enumeration doesn't let us pick a smaller arrow, only a slimmer one.
So we need to draw it ourselves:
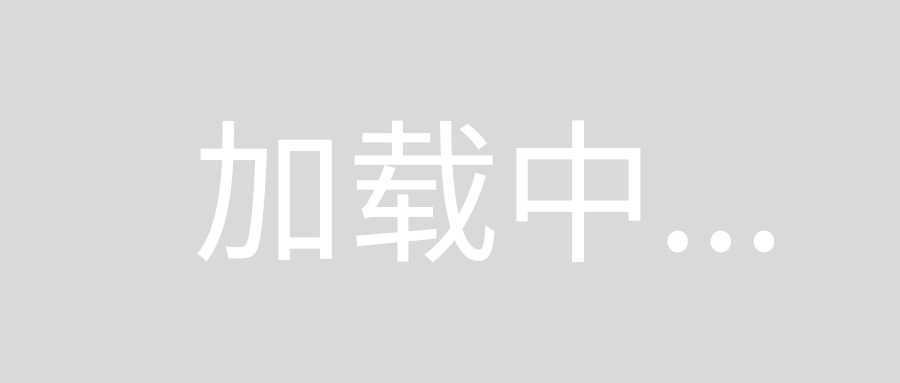
Here is a simple but effective piece of code that achieves just that:
private void chart1_PrePaint(object sender, ChartPaintEventArgs e)
{
if (e.ChartElement.ToString().StartsWith("ChartArea-") )
{
// get the positions of the axes' ends:
ChartArea CA = chart1.ChartAreas[0];
float xx = (float)CA.AxisX.ValueToPixelPosition(CA.AxisX.Maximum);
float xy = (float)CA.AxisY.ValueToPixelPosition(CA.AxisY.Crossing);
float yx = (float)CA.AxisX.ValueToPixelPosition(CA.AxisX.Crossing);
float yy = (float)CA.AxisY.ValueToPixelPosition(CA.AxisY.Maximum);
// a simple arrowhead geometry:
int arrowSize = 18; // size in pixels
Point[] arrowPoints = new Point[3] { new Point(-arrowSize, -arrowSize / 2),
new Point(-arrowSize, arrowSize / 2), Point.Empty };
// draw the two arrows by moving and/or rotating the graphics object:
e.ChartGraphics.Graphics.TranslateTransform(xx + arrowSize, xy);
e.ChartGraphics.Graphics.FillPolygon(Brushes.Black, arrowPoints);
e.ChartGraphics.Graphics.TranslateTransform(yx -xx -arrowSize, yy -xy -arrowSize);
e.ChartGraphics.Graphics.RotateTransform(-90);
e.ChartGraphics.Graphics.FillPolygon(Brushes.Black, arrowPoints);
e.ChartGraphics.Graphics.ResetTransform();
}
}