Data is coming form JSON RESPONSE. In JSON, Every Tag contains number of nodes. Relevant data into second spinner is based on selection of First. My JSON Is Like Here 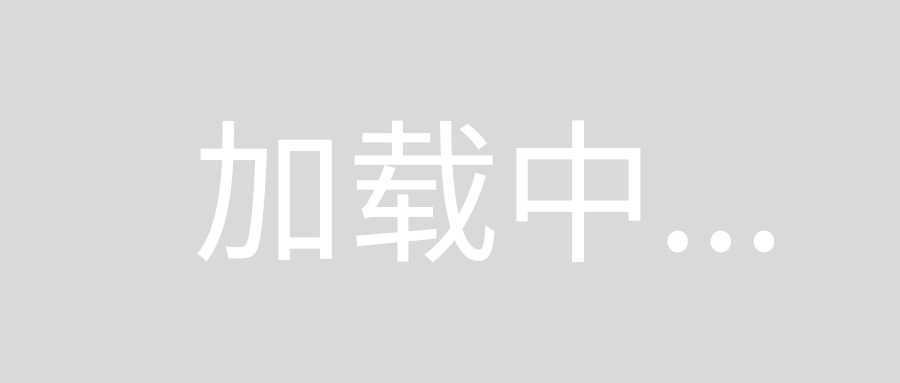
How to Parse JSON and firstly all major nodes into First Spinner. then when User Select that nods, according to selection Data must be reflect into second Spinner. Please guide me .
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Download JSON file AsyncTask
new DownloadJSON().execute();
}
// Download JSON file AsyncTask
private class DownloadJSON extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... params) {
// Locate the WorldPopulation Class
world = new ArrayList<WorldPopulation>();
// Create an array to populate the spinner
worldlist = new ArrayList<String>();
// JSON file URL address
jsonobject = JSONfunctions
.getJSONfromURL("http://www.androidbegin.com/tutorial/jsonparsetutorial.txt");
try {
// Locate the NodeList name
jsonarray = jsonobject.getJSONArray("worldpopulation");
for (int i = 0; i < jsonarray.length(); i++) {
jsonobject = jsonarray.getJSONObject(i);
WorldPopulation worldpop = new WorldPopulation();
worldpop.setRank(jsonobject.optString("rank"));
worldpop.setCountry(jsonobject.optString("country"));
worldpop.setPopulation(jsonobject.optString("population"));
worldpop.setFlag(jsonobject.optString("flag"));
world.add(worldpop);
// Populate spinner with country names
worldlist.add(jsonobject.optString("country"));
}
} catch (Exception e) {
Log.e("Error", e.getMessage());
e.printStackTrace();
}
return null;
}
@Override
protected void onPostExecute(Void args) {
// Locate the spinner in activity_main.xml
Spinner mySpinner = (Spinner) findViewById(R.id.my_spinner);
// Spinner adapter
mySpinner
.setAdapter(new ArrayAdapter<String>(MainActivity.this,
android.R.layout.simple_spinner_dropdown_item,
worldlist));
// Spinner on item click listener
mySpinner
.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> arg0,
View arg1, int position, long arg3) {
// TODO Auto-generated method stub
// Locate the textviews in activity_main.xml
TextView txtrank = (TextView) findViewById(R.id.rank);
TextView txtcountry = (TextView) findViewById(R.id.country);
TextView txtpopulation = (TextView) findViewById(R.id.population);
// Set the text followed by the position
txtrank.setText("Rank : "
+ world.get(position).getRank());
txtcountry.setText("Country : "
+ world.get(position).getCountry());
txtpopulation.setText("Population : "
+ world.get(position).getPopulation());
}
@Override
public void onNothingSelected(AdapterView<?> arg0) {
// TODO Auto-generated method stub
}
});
}
}
This code can give you little idea about populating your objects from JSON
which you get from the API's.
What you want to do is quite simple but i tried to keep things as generic as possible so anyone else ca make a use of this code.
the spinner
represented in the code is populated by ArrayList
of WorldPopulation
type. you will notice that toString
of WorldPopulation
is overridden that it can be used in the ArrayAdapter
so the name of the country will be picked up.
public class LoadDataActivity extends AppCompatActivity {
private Spinner spinner;
private ArrayList<WorldPopulation> worldPopulations;
private TextView rank_TextView;
private TextView country_TextView;
private TextView population_TextView;
private TextView url_TextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_load_data);
spinner = (Spinner) findViewById(R.id.LoadDataActivity_spinner);
rank_TextView = (TextView) findViewById(R.id.LoadDataActivity_rank_textView);
country_TextView = (TextView) findViewById(R.id.LoadDataActivity_country_textView);
population_TextView = (TextView) findViewById(R.id.LoadDataActivity_population_textView);
url_TextView = (TextView) findViewById(R.id.LoadDataActivity_url_textView);
spinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id) {
WorldPopulation population = (WorldPopulation) spinner.getItemAtPosition(position);
rank_TextView.setText("" + population.getRank());
country_TextView.setText("" + population.getCountry());
population_TextView.setText("" + population.getPopulation());
url_TextView.setText("" + population.getFlag());
}
@Override
public void onNothingSelected(AdapterView<?> parent) {
}
});
new DownloadData().execute();
}
private class DownloadData extends AsyncTask<Void, Void, Void> {
@Override
protected void onPreExecute() {
super.onPreExecute();
}
@Override
protected Void doInBackground(Void... params) {
try {
worldPopulations = new ArrayList<>();
URL url = new URL("http://www.androidbegin.com/tutorial/jsonparsetutorial.txt");
HttpURLConnection httpURLConnection = (HttpURLConnection) url.openConnection();
httpURLConnection.connect();
String result = IOUtils.toString(httpURLConnection.getInputStream());
System.out.println("" + result);
JSONObject jsonObject = new JSONObject(result);
JSONArray jsonArray = jsonObject.getJSONArray("worldpopulation");
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject singleItem = jsonArray.getJSONObject(i);
WorldPopulation population = new WorldPopulation();
population.rank = singleItem.getString("rank");
population.country = singleItem.getString("country");
population.population = singleItem.getString("population");
population.flag = singleItem.getString("flag");
worldPopulations.add(population);
}
Log.d("TAG", "" + result);
Log.d("TAG", "size: " + worldPopulations.size());
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
@Override
protected void onPostExecute(Void aVoid) {
super.onPostExecute(aVoid);
ArrayAdapter<WorldPopulation> adapter = new ArrayAdapter<WorldPopulation>(getApplicationContext(), android.R.layout.simple_spinner_dropdown_item, worldPopulations);
adapter.setDropDownViewResource(android.R.layout.simple_list_item_1);
spinner.setAdapter(adapter);
}
}
class WorldPopulation {
private String rank;
private String country;
private String population;
private String flag;
public String getRank() {
return rank;
}
public void setRank(String rank) {
this.rank = rank;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public String getPopulation() {
return population;
}
public void setPopulation(String population) {
this.population = population;
}
public String getFlag() {
return flag;
}
public void setFlag(String flag) {
this.flag = flag;
}
@Override
public String toString() {
return country;
}
}
}
activity_load_data.xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="4dp"
android:orientation="vertical">
<Spinner
android:id="@+id/LoadDataActivity_spinner"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_margin="4dp" />
<TextView
android:id="@+id/LoadDataActivity_rank_textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="4dp"
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/LoadDataActivity_country_textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="4dp"
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/LoadDataActivity_population_textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="4dp"
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/LoadDataActivity_url_textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="4dp"
android:textAppearance="?android:attr/textAppearanceMedium" />
</LinearLayout>
Output
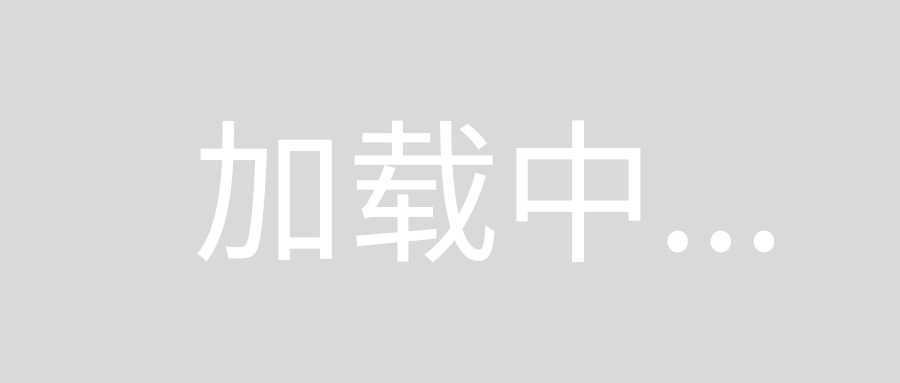
Now that you have the data being populated in the Spinner
you can utilize this to trigger the populate second spinner
depending on the selection on the first spinner
.
Apparently I didn't see any link that would tell me about the second source of data, i will leave the answer here until next update.