I am working simple app where I can get current DateTime
and convert that into 24-hour
format.
Code:
String DATE_yyyy_MM_dd_hh_mm_ss = "yyyy-MM-dd hh:mm:ss";
String DATE_yyyy_MM_dd_HH_mm_ss = "yyyy-MM-dd HH:mm:ss";
TextView tv=(TextView)findViewById(R.id.textView);
tv.append("\n in 12-hour format: "+getDateFormatted(bootDate));
tv.append("\n in 24-hour format: "+getDateFormatted2(bootDate));
public String getDateFormatted(Date date){
return String.valueOf(DateFormat.format(DATE_yyyy_MM_dd_hh_mm_ss, date));
}
public String getDateFormatted2(Date date){
return String.valueOf(DateFormat.format(DATE_yyyy_MM_dd_HH_mm_ss, date));
}
Problem:
It's perfectly working in my devices Samsung Galaxy S3(Android 4.3), S4(Android 4.3) and Nexus 5(Android 4.4)
. while the same code I am running in Huawei Ascend Y330 device(Android 4.2.2)
it's not display properly.
Screenshot in Samsung Galaxy S3, S4 and Nexus 5:
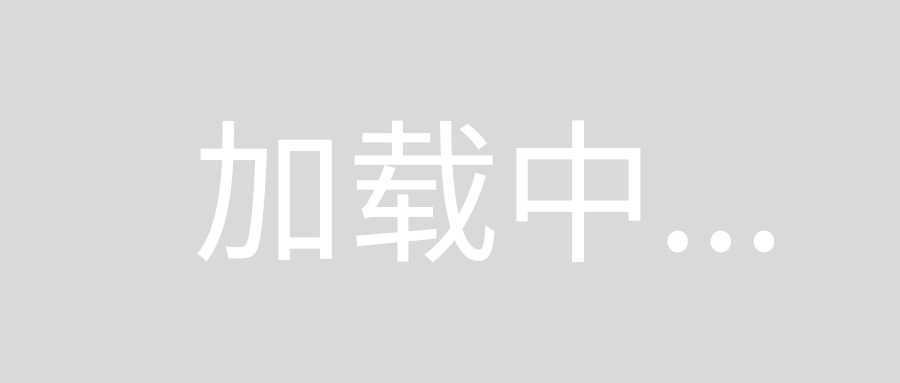
Screenshot in Huawei Ascend Y330:
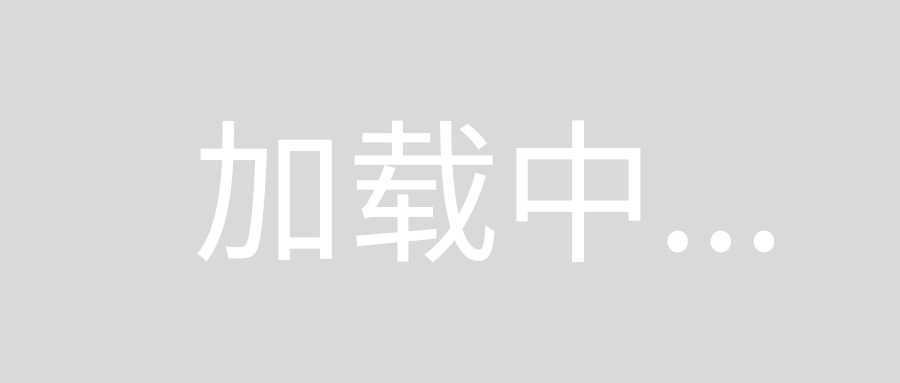
So, what is the problem actually? I don't understand. Is it android system issue? or device issue?
Any idea anyone.
So this post suggested to use SimpleDateFormat instead of DateFormat. For more explanation about the differences between these two classes, you could take a look at this post.
According to the documentation SimpleDateFormat
can handle the 'H' for 24h format but DateFormat
needs 'k'.
Use a literal 'H' (for compatibility with SimpleDateFormat and
Unicode) or 'k' (for compatibility with Android releases up to and
including Jelly Bean MR-1) instead. Note that the two are
incompatible.
Try this,
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss").format(new Date());
SimpleDateFormat sdf2 = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(new Date());
TextView tv=(TextView)findViewById(R.id.textView);
tv.append("\n in 12-hour format: " +sdf);
tv.append("\n in 24 -hour format: " +sdf2);
This works in all the devices,
static final SimpleDateFormat IN_DATE_FORMAT = new SimpleDateFormat("HH:mm:ss", Locale.US);
static final SimpleDateFormat OUT_DATE_FORMAT = new SimpleDateFormat("hh:mma", Locale.US);
/**
* To format the time.
*
* @param strDate The input date in HH:mm:ss format.
* @return The output date in hh:mma format.
*/
private String formatTime(String strDate) {
try {
Date date = IN_DATE_FORMAT.parse(strDate);
return OUT_DATE_FORMAT.format(date);
} catch (Exception e) {
return "N/A";
}
}
use the method : formatTime(YOUR_TIME)