I am developing a Xamarin.Forms
UWP application.
I am struggling to set the accent colour of my application. This is the colour that is used for certain behaviors by default on controls.
For example the Entry control has a default blue highlighting on focus shown below:
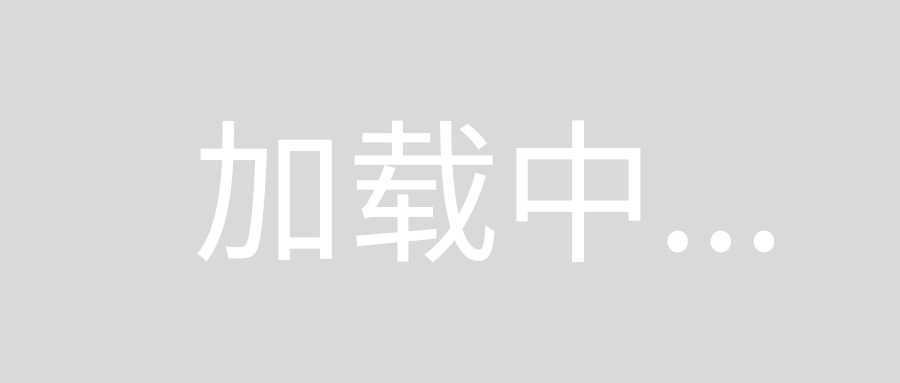
I have tried a few suggestions from this thread: Change Accent Color in Windows 10 UWP but none seemed to work.
I am not sure whether it is because I didn't fully understand how changing the colour for UWP differs for Xamarin.UWP, or whether what I'm trying to do is even possible with Xamarin.Forms.
Has anyone found out how to do this?
Here is the style code of FormsTextBox for UWP.
You need to override below styled colors:
<Setter Property="Foreground" Value="{ThemeResource SystemControlForegroundBaseHighBrush}" />
<Setter Property="Background" Value="{ThemeResource SystemControlBackgroundAltHighBrush}" />
<Setter Property="BackgroundFocusBrush" Value="{ThemeResource SystemControlBackgroundChromeWhiteBrush}" />
<Setter Property="BorderBrush" Value="{ThemeResource SystemControlForegroundChromeDisabledLowBrush}" />
So to change the colour of your textbox boarder brush you can add these ThemeResources
to your App.xaml
like so:
<Application.Resources>
<ResourceDictionary>
<ResourceDictionary.ThemeDictionaries>
<ResourceDictionary x:Key="Light">
<SolidColorBrush x:Key="SystemControlHighlightAccentBrush" Color="#ff0000" />
</ResourceDictionary>
</ResourceDictionary.ThemeDictionaries>
</ResourceDictionary>
</Application.Resources>
You can define a style setter property in App.xaml
<ResourceDictionary>
<Style TargetType="Button" x:Name="myNewButtonStyle">
<Setter Property="Background" Value="{ThemeResource ButtonBackgroundThemeBrush}" />
</Style>
</ResourceDictionary>
Then use CustomRenderer for the controls you need to change colors
protected override void OnElementChanged(ElementChangedEventArgs<Button> e)
{
base.OnElementChanged(e);
if (this.Element != null)
{
this.Control.Style = Windows.UI.Xaml.Application.Current.Resources["myNewButtonStyle"] as Windows.UI.Xaml.Style;
}
}
in a similar way you would be able to use Themed Resource Dictionary key and apply. This code can be used to have native styles on Xamarin's control.