I have a string like this string strings=" black door,white door,red door "
Now I want to put this string into array.
I use split myarray = strings.split(',')
then array look like this: black,door,white,door,red,door.
I want to put the string into the array after each occurance of comma not on the space. I want it like this in the array:
black door,white door,red door.
if you have "black door,white door,red door" string then use only ,
as separator
var result = "black door,white door,red door".Split(',');
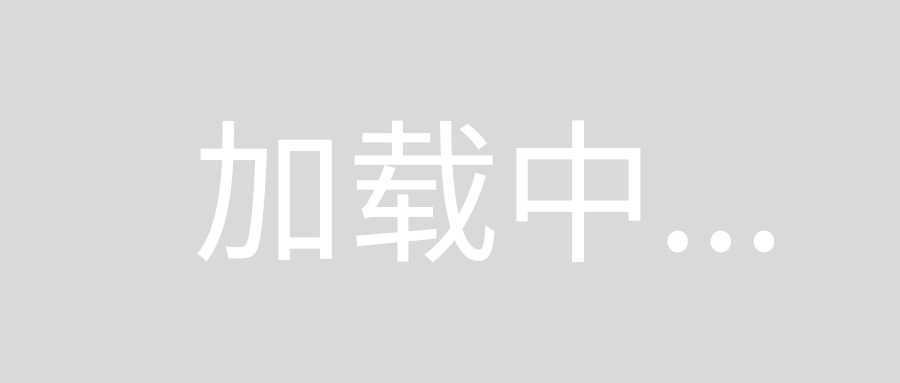
use split like this
var result = myString.Split(',');
It will split only on , and not the whitespace, and should give you the expected result.
You need:
var array = input.Split(',');
ToArray() was unnecessary.
string s = "black door,white door,red door";
string[] sarr;
sarr = s.Split(',');
Could you post your own code in its entirety? It seems we all agree that this is the proper way to do it.
Have you tried iterating through the array and printing out the values?
string strings = "black door,white door,red door";
string[] myarray = strings.Split(',');
foreach (string temp in myarray)
{
MessageBox.Show(temp);
}
Try this:
string input = "black door,white door,red door";
string[] values = input.Split(new char[] { ',' }, StringSplitOptions.RemoveEmptyEntries);