Has any of you encountered a similar memory leak?
This is how I'm handling the VideoView at the moment
@Override
protected void onFinishInflate() {
super.onFinishInflate();
ButterKnife.bind(this);
Uri videoUri = Uri.parse(String.format("android.resource://%s/%s", getContext().getPackageName(), videoRes));
videoView.setVideoURI(videoUri);
videoView.setOnPreparedListener(mp -> {
mp.setLooping(true);
videoView.start();
});
}
This is what I get on LeakCanary
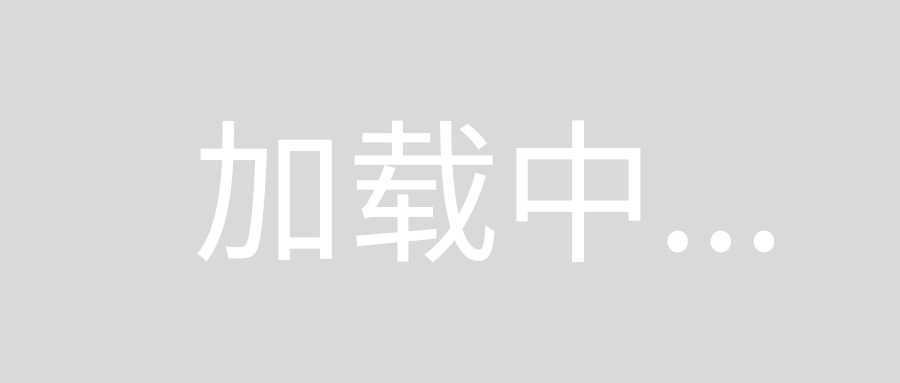
Any help appreciated!
When using ButterKnife with Fragments, you need to use the Unbinder
in onDestroyView()
to correctly dereference the Fragment's Views -- since Fragments have different life cycles to Activities.
There is a related issue here.
If you are using Butterknife make sure to unbind, and if you aint't make sure to call videoView.stopPlayback() in your onDestroy
This fixed it for me within an AppCompatActivity
that's not using Butterknife
Step 1: Create a utility class
public class AudioServiceContext extends ContextWrapper {
public AudioServiceContext(Context base) {
super(base);
}
public static ContextWrapper getContext(Context base) {
return new AudioServiceContext(base);
}
@Override
public Object getSystemService(String name) {
if (Context.AUDIO_SERVICE.equals(name)) {
return getApplicationContext().getSystemService(name);
}
return super.getSystemService(name);
}
}
Step 2: within your Activity, use this utility class
public class YourVideoActivity extends AppCompatActivity {
private VideoView videoView;
@Override
protected void attachBaseContext(Context newBase) {
super.attachBaseContext(AudioServiceContext.getContext(newBase));
}
//etc...
}
Credit: https://medium.com/@chauyan/confirmed-videoview-leak-on-android-ac502856a6cf
Call videoView.suspend()
in onPause()
or onDestory()
.