How to open Google Play in popup like Wisher, Buzzfeed, Vimeo?
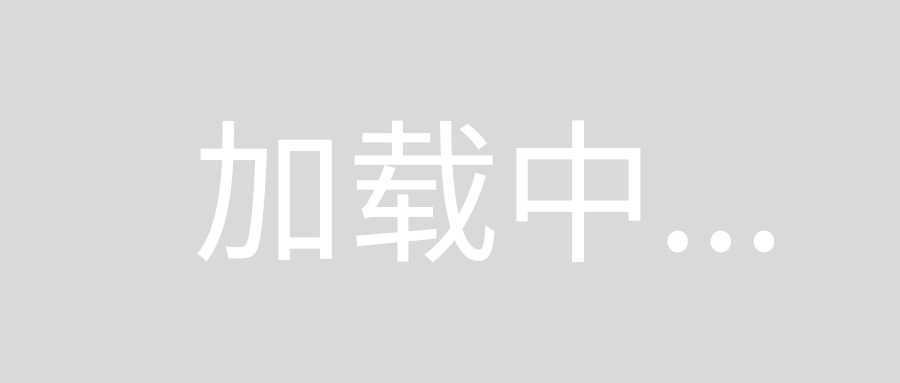
I looked at Google documentation, but there is only about opening by Google Play app (market://
) or by a browser (http://
).
I would like to open inside my instant app in order to install full app like on screens.
I think you're looking for showInstallPrompt.
TL;DR:
InstantApps.showInstallPrompt(activity,
postInstallIntent,
Constants.INSTALL_INSTANT_APP_REQUEST_CODE,
referrerString);
At Vimeo we're using Branch for our web -> mobile app install as well as our instant app -> mobile app install (since it gives some additional metrics and lets us compare the referrers a little better).
If you're interested in using Branch, the documentation for the install prompt can be found here. And the usage looks like:
if (Branch.isInstantApp(this)) {
myFullAppInstallButton.setVisibility(View.VISIBLE);
myFullAppInstallButton.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
BranchUniversalObject branchUniversalObject = new BranchUniversalObject()
.setCanonicalIdentifier("item/12345")
.setTitle("My Content Title")
.setContentDescription("My Content Description")
.setContentImageUrl("https://example.com/mycontent-12345.png")
.setContentMetadata(new ContentMetadata()
.addCustomMetadata("property1", "blue")
.addCustomMetadata("property2", "red"));
Branch.showInstallPrompt(myActivity, activity_ret_code, branchUniversalObject);
}
});
} else {
myFullAppInstallButton.setVisibility(View.GONE);
}
Branch's implementation ultimately calls through to the API mentioned in the other answer here.
Which looks like:
public static boolean showInstallPrompt(Activity activity,
Intent postInstallIntent,
int requestCode,
String referrer)
Shows a dialog that allows the user to install the current instant app. This method is a no-op if the current running process is an installed app. You must provide a post-install intent, which the system uses to start the application after install is complete.
You can find an example usage here. And looks like the following:
class InstallApiActivity : AppCompatActivity() {
/**
* Intent to launch after the app has been installed.
*/
private val postInstallIntent = Intent(Intent.ACTION_VIEW,
Uri.parse("https://install-api.instantappsample.com/")).
addCategory(Intent.CATEGORY_BROWSABLE).
putExtras(Bundle().apply {
putString("The key to", "sending data via intent")
})
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_install)
val isInstantApp = InstantApps.isInstantApp(this)
findViewById<Button>(R.id.start_installation).apply {
isEnabled = isInstantApp
// Show the installation prompt only for an instant app.
if (isInstantApp) {
setOnClickListener {
InstantApps.showInstallPrompt(this@InstallApiActivity,
postInstallIntent,
REQUEST_CODE,
REFERRER)
}
}
}
}
companion object {
private val REFERRER = "InstallApiActivity"
private val REQUEST_CODE = 7
}
}
It's not recommended because it's deprecated, but you can technically get the dialog to show using the following code:
InstantApps.showInstallPrompt(activity, Constants.INSTALL_INSTANT_APP_REQUEST_CODE, null);
See also: https://stackoverflow.com/a/47666873/1759443