I'm selecting a set of random questions without any duplicates using the following:
<?php
$amount = get_field('select_number_of_questions');
$repeater = get_field("step_by_step_test");
shuffle($repeater);
$repeater_limit = array_slice($repeater,0,$amount);
foreach($repeater_limit as $repeater_row) {
echo "<p>".$repeater_row['question']."</p>";
$rows = $repeater_row['answer_options'];
foreach($rows as $row) {
echo $row['answer']."<br />";
}
}
?>
Each question has a field: get_field('required_question');
that has a yes/no dropdown. The questions that have yes selected ALWAYS have to be incorporated into the loop above.
E.g The test has 20 questions to select from, 10 will be selected at random. Within the 20 questions, there are 2 required questions (i.e these will always be selected). So it will need to grab the 2 required questions and select 8 other random questions.
How can I include the required questions within the random selection?
The Question doesn't state it, but all suggests this is an Advanced Custom Fields set up using the Repeater Add-on.
In that case, this is the test configuration I've done:
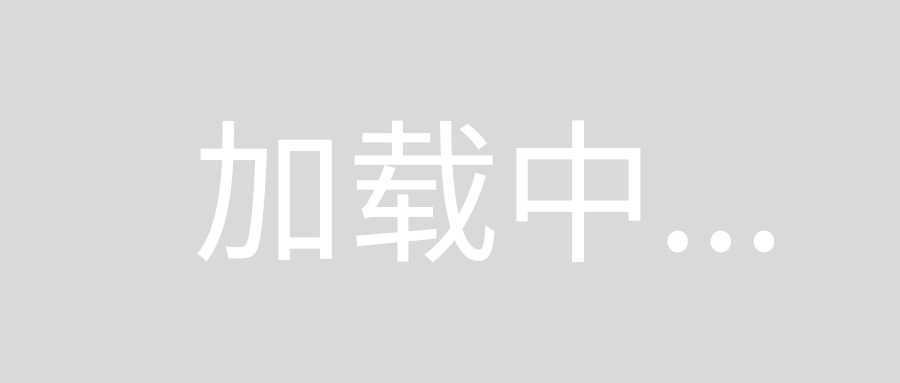
Note that here I'm using $repeater_row['title']
instead of the OP's $repeater_row['question']
. Also, I removed the answer_options
part. See comments for details:
// Get fields
$amount = get_field( 'select_number_of_questions' );
$repeater = get_field( 'step_by_step_test' );
// Auxiliary arrays to separate fields by Field Name
$not_enabled = array();
$enabled = array();
// Separate
foreach( $repeater as $field )
{
if( 'no' == $field['enabled'] )
$not_enabled[] = $field;
else
$enabled[] = $field;
}
// Discount the enabled from the the total amount
$amount = (int)$amount - count( $enabled );
// Shuffle before slicing
shuffle( $not_enabled );
$repeater_limit = array_slice( $not_enabled, 0, $amount );
// Add enabled fields and shuffle again
$final_array = array_merge( $repeater_limit, $enabled );
shuffle( $final_array );
foreach( $final_array as $repeater_row ) {
echo "<p>" . $repeater_row['title'] . "</p>";
}
First you need to filter out the required questions like so:
$all_questions = get_field("step_by_step_test");
$required = $optional = array();
foreach($all_questions as $question) {
if( $a['required_question']) $required[] = $question;
else $optional[] = $question;
}
$amount = get_field("select_number_of_questions")-count($required);
shuffle($optional);
$final = array_merge($required,array_slice($optional,0,$amount));
foreach($final as $repeater_row) {
...
}
Hope I helped you again :p