I'm new to Project Server development and was wondering if you can use the PSI to set resource custom field values.
I can't seem to find any information for a beginner at this.
I currently have web references set up for the CustomFields and the Resource web service, but am unsure how to set a custom field for a particular resource.
Any help would be really appreciated!
Thanks!
I know your problem. Microsoft has really bad examples on MSDN. Lot of stuff doesn't work or has just been copied from the 2007 manual. I've started yesterday to develop with the Webservice for Project Server 2010.
Here is a simple (maybe not the best) solution for setting custom fields:
Complete source code: http://pastebin.com/tr7CGJsW
Prepare your solution
First I've added a Service Reference to:
http: //servername/instance/_vti_bin/PSI/Project.asmx?wsdl
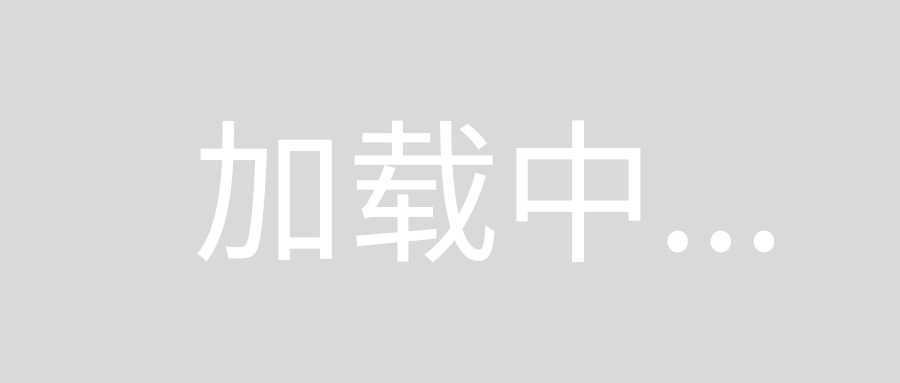
After that, I've edited the app.config because I had to use special credentials to login into the project server (maybe this is not necessarily for you):
...
<security mode="TransportCredentialOnly">
<transport clientCredentialType="Ntlm" proxyCredentialType="Ntlm" realm="" />
<message clientCredentialType="UserName" algorithmSuite="Default" />
</security>
<!--<security mode="None">
<transport clientCredentialType="None" proxyCredentialType="None"
realm="" />
<message clientCredentialType="UserName" algorithmSuite="Default" />
</security>-->
</binding>
The commented code has been created by Visual Studio
Connect and Update
Now we can create a new SoapClient which communicates with the Project Server:
//Creating a new service client object
ProjectSoapClient projectSvc = new ProjectSoapClient();
//Just if you need to authenticate with another account!
projectSvc.ClientCredentials.Windows.ClientCredential = new NetworkCredential("test", "test", "demo");
projectSvc.ClientCredentials.Windows.AllowedImpersonationLevel = TokenImpersonationLevel.Impersonation;
Now I have declared two Guids, that we know, what we want to update. The other variables are used later and are comment in the code:
//Guid of my project
Guid myProjectId = new Guid("{610c820f-dc74-476c-b797-1e61a77ed6c6}");
//Guid of the custom field
Guid myCustomFieldId = new Guid("{cd879634-b3ee-44eb-87f7-3063a3523f45}");
//creating a new sessionId and a new jobId
Guid sessionId = Guid.NewGuid(); //the sessionId stays for the whole updating process
Guid jobId = Guid.NewGuid(); //for each job, you give to the server, you need a new one
//indicator if you have to update the project
Boolean updatedata = false;
Then we are ready to load the ProjectDataSet from the server, find the CustomField and updating the data. It's really simple:
- loading the ProjectDataSet
- iterate trough the CustomFieldsRow
- checking if the CustomField matches with our Guid
- updating the value
- setting the indicator to update
//loading project data from server
//Every change on this dataset will be updated on the server!
ProjectDataSet project = projectSvc.ReadProject(myProjectId, DataStoreEnum.WorkingStore);
//To find your custom field, you have to search for it in the CustomFieldsRow
foreach (ProjectServerCSVImport.PSS.Project.ProjectDataSet.ProjectCustomFieldsRow row in project.ProjectCustomFields)
{
//check if the GUID is the same
if (row.MD_PROP_UID == myCustomFieldId)
{
//if yes, write it into the container
row.NUM_VALUE = 12345;
//and set the indicater
updatedata = true;
}
}
If we changed a value, we have to send the ProjectDataSet to the ProjectServer now. It will update the changed values in the ProjectDataSet. For this, we have to check out our project, update it and check in again:
//update if you have changed anything
if (updatedata)
{
//check out the project first
projectSvc.CheckOutProject(myProjectId, sessionId, "custom field update checkout");
//send the dataset to the server to update the database
bool validateOnly = false;
projectSvc.QueueUpdateProject(jobId, sessionId, project, validateOnly);
//wait 4 seconds just to be sure the job has been done
System.Threading.Thread.Sleep(4000);
//create a new jobId to check in the project
jobId = Guid.NewGuid();
//CheckIn
bool force = false;
string sessionDescription = "updated custom fields";
projectSvc.QueueCheckInProject(jobId, myProjectId, force, sessionId, sessionDescription);
//wait again 4 seconds
System.Threading.Thread.Sleep(4000);
But now we just have updated the database. The server will still show us the "old" value and not the new one. This is because we didn't have published our project yet:
//again a new jobId to publish the project
jobId = Guid.NewGuid();
bool fullPublish = true;
projectSvc.QueuePublish(jobId, myProjectId, fullPublish, null);
//maybe we should wait again ;)
System.Threading.Thread.Sleep(4000);
Finally we're done and our project is updated!
Enhancements
I'm very new to this platform (Project Server 2010), so this code maybe is not the best example. So there are some enhancements, which would made the solution better:
- The Sleep function is a very bad workaround, but I couldn't find an example which handles the same thing (like here) with a QueueSystem for 2010?!
- If you don't know the Guid of your project, but the name, you can resolve it by following function:
/// <summary>
/// Returns the GUID for a specified project
/// and sets the guid for this class
/// </summary>
/// <param name="client">soap service client</param>
/// <param name="projectname">name of the project</param>
/// <returns>Project GUID</returns>
public Guid GetGuidByProjectName(ProjectSoapClient client, string projectname)
{
Guid pguid = new Guid();
ProjectDataSet data = client.ReadProjectList();
foreach (DataRow row in data.Tables[0].Rows)
{
if (row[1].ToString() == projectname) //compare - case sensitive!
{
pguid = new Guid(row[0].ToString());
}
}
return pguid;
}