I've retrieved createdAt column from Parse and i wrote it on UITableview for each row, but the time and date looks like this on tableview :
23-12-2015 01:04:56.380
28-08-2015 19:45:09.101
I want to convert that to time ago for example:
Today 1:04 PM -- Yestarday 5:24 AM -- 3 days ago -- 1 week ago -- 1 month ago
Here is my code:
let dateFormatter = NSDateFormatter()
dateFormatter.dateFormat = "dd-MM-yyyy HH:mm:ss.SSS"
let date = dateFormatter.stringFromDate((msg.createdAt as NSDate?)!)
Cell.timelbl.text = date
I'm using xcode 7 beta
Any help please?
You can use NSCalendar isDateInToday to check if createdAt date is in same day as today, and use isDateInYesterday to check if it was yesterday. Just add a conditional and return a custom string for those conditions, for all other conditions just let date components formatter take care of it for you.
extension Formatter {
static let time: DateFormatter = {
let formatter = DateFormatter()
formatter.calendar = Calendar(identifier: .gregorian)
formatter.dateFormat = "h:mm a"
return formatter
}()
static let dateComponents: DateComponentsFormatter = {
let formatter = DateComponentsFormatter()
formatter.calendar = Calendar(identifier: .iso8601)
formatter.unitsStyle = .full
formatter.maximumUnitCount = 1
formatter.zeroFormattingBehavior = .default
formatter.allowsFractionalUnits = false
formatter.allowedUnits = [.year, .month, .weekOfMonth, .day, .hour, .minute, .second]
return formatter
}()
}
extension Date {
var time: String { return Formatter.time.string(from: self) }
var year: Int { return Calendar.autoupdatingCurrent.component(.year, from: self) }
var month: Int { return Calendar.autoupdatingCurrent.component(.month, from: self) }
var day: Int { return Calendar.autoupdatingCurrent.component(.day, from: self) }
var elapsedTime: String {
if timeIntervalSinceNow > -60.0 { return "Just Now" }
if isInToday { return "Today at \(time)" }
if isInYesterday { return "Yesterday at \(time)" }
return (Formatter.dateComponents.string(from: Date().timeIntervalSince(self)) ?? "") + " ago"
}
var isInToday: Bool {
return Calendar.autoupdatingCurrent.isDateInToday(self)
}
var isInYesterday: Bool {
return Calendar.autoupdatingCurrent.isDateInYesterday(self)
}
}
testing:
Calendar.autoupdatingCurrent.date(byAdding: .second, value: -59, to: Date())!
.elapsedTime // "Just Now"
Calendar.autoupdatingCurrent.date(byAdding: .minute, value: -1, to: Date())!
.elapsedTime // "Today at 5:03 PM"
Calendar.autoupdatingCurrent.date(byAdding: .hour, value: -1, to: Date())!
.elapsedTime // "Today at 4:04 PM"
Calendar.autoupdatingCurrent.date(byAdding: .day, value: -1, to: Date())!
.elapsedTime // "Yesterday at 5:02 PM"
Calendar.autoupdatingCurrent.date(byAdding: .weekOfYear, value: -1, to: Date())!
.elapsedTime // "1 week ago"
Calendar.autoupdatingCurrent.date(byAdding: .month, value: -2, to: Date())!
.elapsedTime // "2 months ago"
Calendar.autoupdatingCurrent.date(byAdding: .year, value: -1, to: Date())!
.elapsedTime // "1 year ago"
Wow, the above answer was too much work for me! This is what I did! I think you will find that it's less of a headache on your end!
First go and download and import NSDate-TimeAgo from Kevin Lawler on Github into your project.
If you are using a tableView, which it looks like you are. This is how I did it. Assign a tag to your label in Interface Builder under "Attributes Inspector" (make sure you have your label selected).
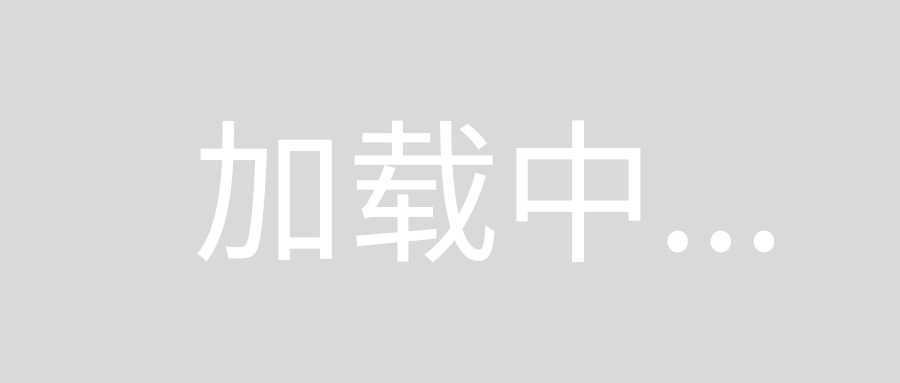
In your tableViewController.m file, you will need to find cellForRowAtIndexPath, since you are using Parse, I'm assuming you are using a PFQueryTableViewController, so you will be placing your code inside:
- (PFTableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath object: (PFObject *)object
{
}
Import NSDate+TimeAgo.h into your tableView.h file and then just place your code inside the curly braces and make sure you return your cell. (note: make sure you have a reference to PFObject like in the above code)
///////////////DATE LABEL (WHEN CREATED)//////////////
//Reference to label in interface builder//
UILabel *myDateLabel = (UILabel*) [cell viewWithTag:103];
//reference to PFObject date of creation from Parse//
NSDate *createdAt = object.createdAt;
//create a string from timeAgo and Parse date//
NSString *timeAgo = [createdAt timeAgo];
//assign string to your label//
myDateLabel.text = timeAgo;