I have created a slide out menu using Swift. I have done this many times before, but when I created it today, I get this error (see screenshot). It could just be a simple mistake I have made.
Here is the code, that I think is causing the problem:
override func tableView(tableView: UITableView!, cellForRowAtIndexPath indexPath: NSIndexPath!) -> UITableViewCell! {
var cell:UITableViewCell? = tableView.dequeueReusableCellWithIdentifier("Cell")! as UITableViewCell
if cell == nil{
cell = UITableViewCell(style: UITableViewCellStyle.Default, reuseIdentifier: "Cell")
cell!.backgroundColor = UIColor.clearColor()
cell!.textLabel!.textColor = UIColor.darkTextColor()
let selectedView:UIView = UIView(frame: CGRect(x: 0, y: 0, width: cell!.frame.size.width, height: cell!.frame.size.height))
selectedView.backgroundColor = UIColor.blackColor().colorWithAlphaComponent(0.3)
cell!.selectedBackgroundView = selectedView
}
cell!.textLabel!.text = tableData[indexPath.row]
return cell
}
Screenshot:
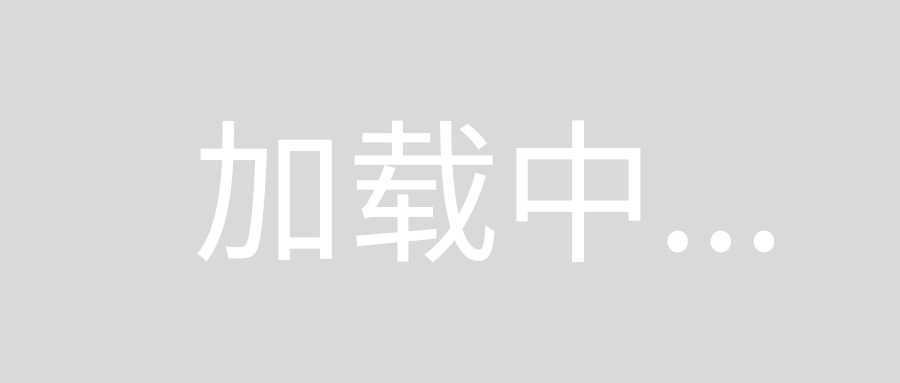
UPDATE: I have tried removing override
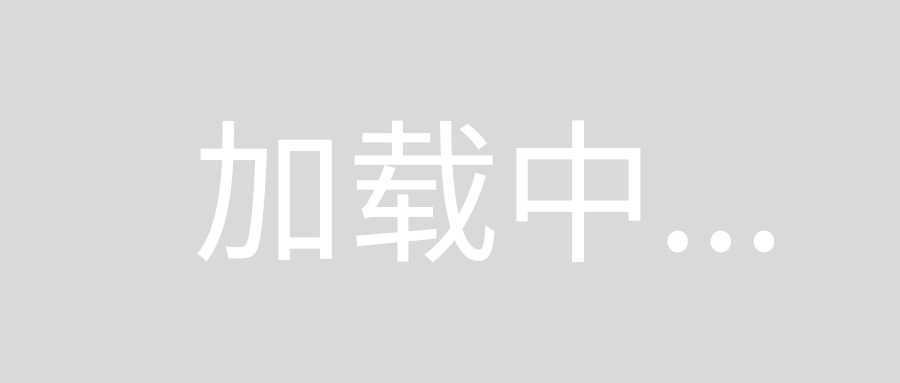
Hope someone can help!
The method does not override any method of the superclass because the signature is wrong.
The correct signature is
override func tableView(tableView: UITableView,
cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
All parameters are non-optional types.
And use also the recommended method
let cell = tableView.dequeueReusableCellWithIdentifier(cellIdentifier,
forIndexPath: indexPath)
which also returns always a non-optional type
Make sure that your class implements UITableViewDelegate
and UITableViewDataSource
.
class MyController: UIViewController, UITableViewDelegate, UITableViewDataSource {
// All your methods here
}
You won't need override
keyword unless any other superclass already implements those methods.
See this works for me
- Just confirm
UITableViewDelegate
and UITableViewDataSource
- Implement required methods
import UIKit
class ListViewController: UIViewController, UITableViewDelegate, UITableViewDataSource {
let containArray = ["One","two","three","four","five"]
// MARK: - View Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
}
override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
//MARK: Table view data source and delegate methods
//Number of rows
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return containArray.count
}
//Prepare Custom Cell
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell{
let identifier = "simpleTextCell"
var cell: SimpleTextCell! = tableView.dequeueReusableCellWithIdentifier(identifier) as? SimpleTextCell
if cell == nil {
tableView.registerNib(UINib(nibName: "SimpleTextCell", bundle: nil), forCellReuseIdentifier: identifier)
cell = tableView.dequeueReusableCellWithIdentifier(identifier) as? SimpleTextCell
}
let dayName = containArray[indexPath.row]
cell.lblProductName.text = dayName
return cell
}
//Handle click
func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath) {
self.performSegueWithIdentifier("productListSegue", sender: self)
}
}
Try to removing "!", declaration for this function is:
func tableView(_ tableView: UITableView,cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
and make sure you have set delegate and datasource of tableview to "self"