I am working on R and trying to draw a clock using a pie chart.
code:
pie(c(25,20,15,10,10,30),
labels = c(1,2,3,4,5,6,7,8,9,10,11,12),
col = rainbow(length(lbls)), clockwise = TRUE, init.angle = 90)
but i need all 12 labels to be there independent of no of segments in input.
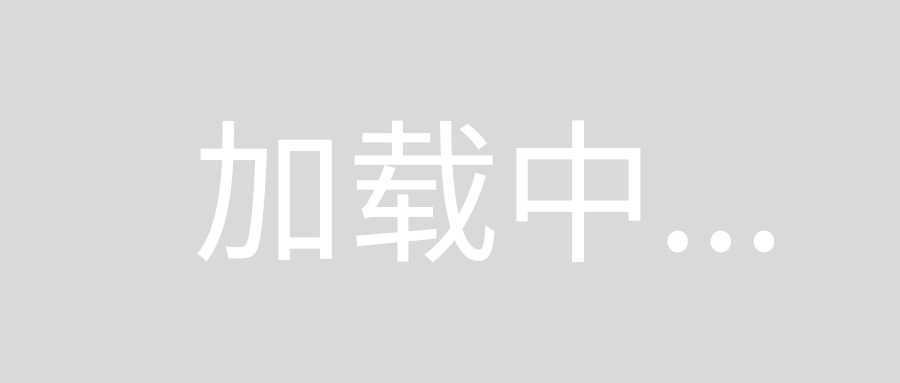
How can i implement it?
Regards
Paul Murrell in his last book (R graphics 2nd Edition) have some code for drawing an interactive clock using grid
and gwidget
.
Here is a sample code for drawing a simple non interactive clock :
require(grid)
drawClock <- function(hour, minute) {
t <- seq(0, 2*pi, length=13)[-13]
x <- cos(t)
y <- sin(t)
grid.newpage()
pushViewport(dataViewport(x, y, gp=gpar(lwd=4)))
# Circle with ticks
grid.circle(x=0, y=0, default="native",
r=unit(1, "native"))
grid.segments(x, y, x*.9, y*.9, default="native")
# Hour hand
hourAngle <- pi/2 - (hour + minute/60)/12*2*pi
grid.segments(0, 0,
.6*cos(hourAngle), .6*sin(hourAngle),
default="native", gp=gpar(lex=4))
# Minute hand
minuteAngle <- pi/2 - (minute)/60*2*pi
grid.segments(0, 0,
.8*cos(minuteAngle), .8*sin(minuteAngle),
default="native", gp=gpar(lex=2))
grid.circle(0, 0, default="native", r=unit(1, "mm"),
gp=gpar(fill="white"))
}
Now you can try it like this
drawClock(hour = 2, minute = 30)
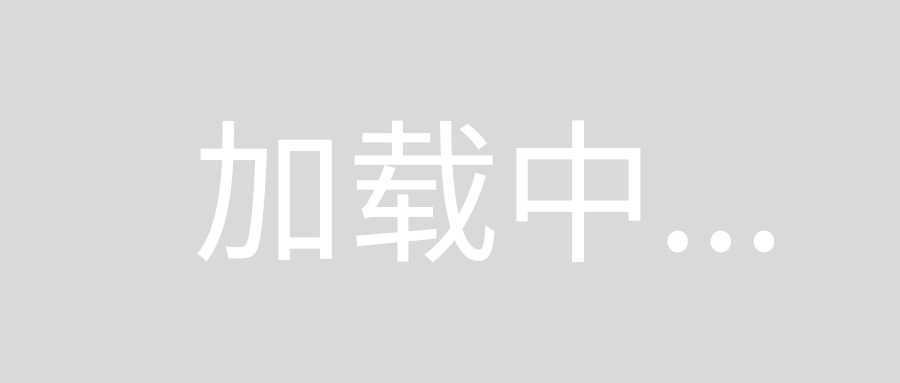
Full code here
An update on this topic after having the same issue.
You should use 'GridBase' package if you want to keep your pie and the clock on top of it.
Example (using dickoa's excellent solution as a basis for the code):
require('grid')
require('gridBase')
pie(c(1,2),
clockwise=TRUE,
radius=1.0,
init.angle=90,
col=c('pink', 'lightblue'),
border=NA)
drawClock <- function(hour, minute)
{
t <- seq(0, 2*pi, length=13)[-13]
x <- cos(t)
y <- sin(t)
vps <- baseViewports()
pushViewport(vps$inner, vps$figure, vps$plot)
# ticks
grid.segments(x, y, x*.9, y*.9, default="native")
# Hour hand
hourAngle <- pi/2 - (hour + minute/60)/12*2*pi
grid.segments(0, 0,
.6*cos(hourAngle), .6*sin(hourAngle),
default="native", gp=gpar(lex=4))
# Minute hand
minuteAngle <- pi/2 - (minute)/60*2*pi
grid.segments(0, 0,
.8*cos(minuteAngle), .8*sin(minuteAngle),
default="native", gp=gpar(lex=2))
popViewport(3)
}
drawClock(hour = 2, minute = 25)
Result:
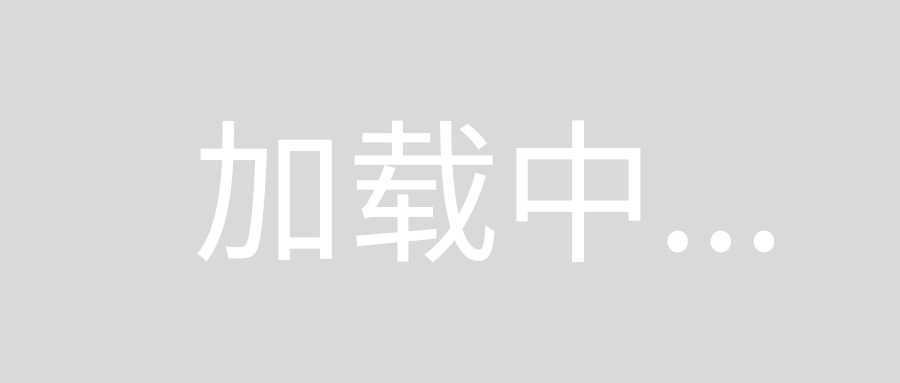