I have a Windows 10 UWP app running on mobile. When I run the app in an emulator, everything works fine. When I run it on a device (Lumia 550), the StatusBar is black with black font and the status indicators are not visible.
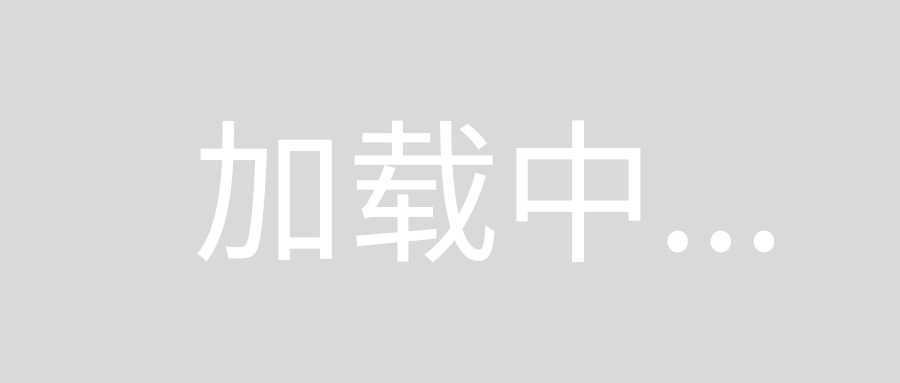
Is this some kind of bug?
I know I can force the StatusBar to have white background and black color, but the requirement for the app is to stick with the theme (black StatusBar in dark theme, white in Light theme).
If I create a new empty Windows 10 app and run it on a device, the problem is the same, it is not specific to my app.
Edit
Here's a more proper answer:
In Windows 10 Mobile the statusbar inherits it's background color from the topmost page. The foreground color is inherited from RequestedTheme
.
This means that if you set the background color of your page to black and your RequestedTheme
to Light
(which gives a white foreground color), the text will be black on black.
Original post
Have you read this?: https://stenobot.wordpress.com/2015/07/08/uwp-app-development-styling-the-mobile-status-bar/
It might help you.
I have struggled with this a bit.
A strange thing is that when I start my app, the theme (Application.RequestedTheme
) is always Light, ignoring the setting on the phone. Even when I try to set it to Dark in the constructor, it immediately reverts to Light. This could explain the black foreground color.
I also experience the inconsistency between simulator and device. To take care of that, I (as suggested in other answer) set the background color of the page (not the root Grid):
<Page
Background="{ThemeResource ApplicationPageBackgroundThemeBrush}"
... >
</Page>
With this, the status bar at least appear readable.
If you got the black font on the black background with your Light theme project, simple add this line into OnLaunched
method in App.xaml.cs
:
rootFrame.Background = new SolidColorBrush(Colors.White);
Just add RequestedTheme="Dark"
in App.xaml
In your reference Manager add Windows Mobile extensions for the UWP
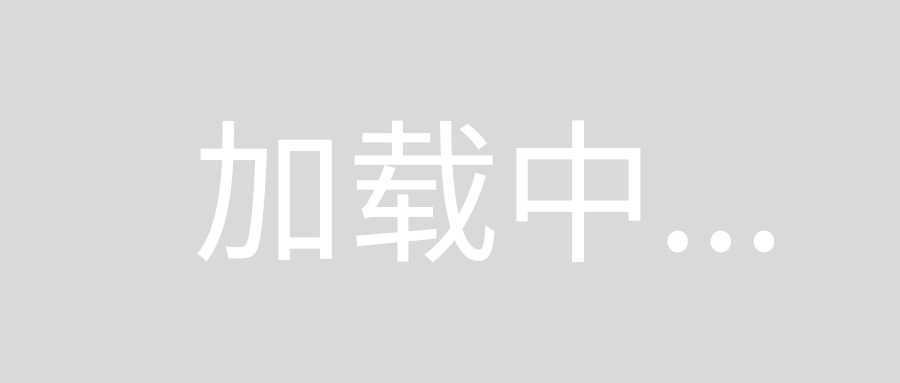
Then in App.XAML.cs
on
protected override void OnLaunched(LaunchActivatedEventArgs e)
add
if (Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
var statusBar = Windows.UI.ViewManagement.StatusBar.GetForCurrentView();
statusBar.BackgroundColor = Windows.UI.Colors.Green;
statusBar.BackgroundOpacity = 1;
statusBar.ForegroundColor = Colors.White;
}
If you want to change Title bar on PC version of UWP app, then you could use this
if (ApiInformation.IsTypePresent("Windows.UI.ViewManagement.ApplicationView"))
{
var titleBar = ApplicationView.GetForCurrentView().TitleBar;
if (titleBar != null)
{
titleBar.ButtonBackgroundColor = Colors.DarkBlue;
titleBar.ButtonForegroundColor = Colors.White;
titleBar.BackgroundColor = Colors.Blue;
titleBar.ForegroundColor = Colors.White;
}
}