I need add some custom buttons on my MapFragment.
I use this code:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
android:layout_width="fill_parent"
android:layout_height="fill_parent"
xmlns:android="http://schemas.android.com/apk/res/android">
<!--suppress AndroidDomInspection -->
<fragment
android:id="@+id/map"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:name="com.google.android.gms.maps.SupportMapFragment"
android:layout_alignParentBottom="true"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentRight="true" />
<RelativeLayout
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:layout_alignParentBottom="true"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentRight="true">
<ImageButton
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_alignParentRight="true"
android:layout_alignParentTop="true"
android:layout_marginRight="10sp"
android:layout_marginTop="10sp"
android:src="@android:drawable/ic_menu_mylocation"/>
<ImageButton
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_alignParentRight="true"
android:layout_alignParentTop="true"
android:layout_marginRight="60sp"
android:layout_marginTop="10sp"
android:src="@android:drawable/ic_menu_mylocation"/>
</RelativeLayout>
I'd like that these button have the same style of zoom buttons: white semitrasparent background, gray border and gray background on click.
How can I set the same style?
Is there a more simple way? (for example official api)
This is my current approximation of a button for the google map api v2. It's all in the XML. I'm happy to share this technique.
<fragment
android:id="@+id/map"
android:layout_width="match_parent"
android:layout_height="fill_parent"
class="com.google.android.gms.maps.SupportMapFragment" />
<Button
android:id="@+id/btnFollow"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="34dp"
android:background="@drawable/mapbutton"
android:padding="14dp"
android:text="@string/btnFollowText"
android:textColor="@color/black" />
/drawable/mapbutton
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle" >
<corners android:radius="2dp" />
<stroke
android:width="1dp"
android:color="#CC444444" />
<solid android:color="#88FFFFFF" />
</shape>
/color/black
<color name="black">#000000</color>
Tailor it for your app.
The best that I could style it is as follows:
Style your button with ImageButton
, and set the android:layout_width
and android:layout_height
to 40dp
. Set android:src
to your own drawble, and android:tint
to #ff595959
. Set the android:background
to the following drawable.
/drawable/mapbutton_state.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_enabled="false">
<shape android:shape="rectangle">
<corners android:radius="1.3dp" />
<stroke android:width="1dp" android:color="#77888888" />
<solid android:color="#44FFFFFF" />
</shape>
</item>
<item
android:state_pressed="true"
android:state_enabled="true">
<shape android:shape="rectangle">
<corners android:radius="1.3dp" />
<stroke android:width="1dp" android:color="#DD888888" />
<solid android:color="#A95badce" />
</shape>
</item>
<item
android:state_focused="true"
android:state_enabled="true">
<shape android:shape="rectangle">
<corners android:radius="1.3dp" />
<stroke android:width="1dp" android:color="#DD888888" />
<solid android:color="#A9FFFFFF" />
</shape>
</item>
<item
android:state_enabled="true">
<shape android:shape="rectangle">
<corners android:radius="1.3dp" />
<stroke android:width="1dp" android:color="#DD888888" />
<solid android:color="#A9FFFFFF" />
</shape>
</item>
</selector>
I made a 9-patch image with a similar background of Google Maps button:
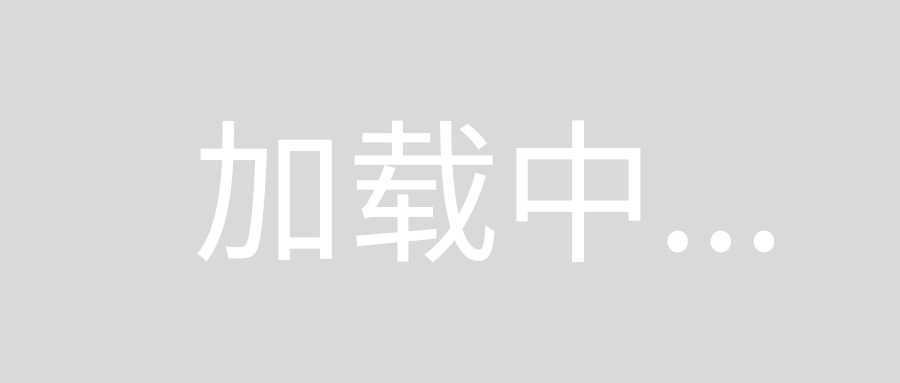
It's on my gist
You can achieve it using the 9Patch tool of the android SDK and using photoshop.
white semitrasparent background.
when you want semitransparent background you can photoshop your 9patch image using a background transparency eg. 80%
transparent which will have the same background effect as the zoom button of the map.
gray background on click
You can use State List of drawable with 2 image, 1 image for light transparency and the other is the graybackground