I have implemented navigation drawer in my android app. but now I want to be able to change the layout using fragments when the user clicks any list item in the navigation bar.
Here is what I have got so far:
XML
<android.support.v4.widget.DrawerLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/drawer_layout"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:id="@+id/content_frame"
android:layout_width="match_parent"
android:background="#000000"
android:layout_height="match_parent" >
</FrameLayout>
<ListView android:id="@+id/left_drawer"
android:layout_width="220dp"
android:layout_height="match_parent"
android:layout_gravity="start"
android:choiceMode="singleChoice"
android:divider="@android:color/transparent"
android:dividerHeight="0dp"
android:background="#111"/>
</android.support.v4.widget.DrawerLayout>
Java File
public class MainActivity extends Activity {
final String[] data ={"one","two","three"};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, data);
final DrawerLayout drawer = (DrawerLayout)findViewById(R.id.drawer_layout);
final ListView navList = (ListView) findViewById(R.id.left_drawer);
navList.setAdapter(adapter);
navList.setOnItemClickListener(new AdapterView.OnItemClickListener(){
@Override
public void onItemClick(AdapterView<?> parent, View view, final int pos,long id){
drawer.setDrawerListener( new DrawerLayout.SimpleDrawerListener(){
@Override
public void onDrawerClosed(View drawerView){
super.onDrawerClosed(drawerView);
}
});
drawer.closeDrawer(navList);
}
});
}
}
Using the above code, I implemented navigation drawer in my app and I see "one", "two" and "Three" list items in the navigation drawer but nothing happens when I click on them except the drawer closes.
So, my question is :
How do I add the fragment functionality to the above given code?
I am beginner. Thanks in advance!
On click have
selectItem(pos);
Then
public void selectItem(int position)
{
switch(position)
{
case 0:
// fragment1
// use fragment transaction and add the fragment to the container
FragmentManager fragmentManager = getFragmentManager()
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
Fragment1 fragment = new Fragment1();
fragmentTransaction.add(R.id.content_frame, fragment);
fragmentTransaction.commit();
break;
case 1:
// fragment2
break;
case 2:
// fragment2
break;
}
}
use This:
public class MenuFragmentActivity extends FragmentActivity{
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.base_layout);
addFragments(new Sample(), false, false,
AndyConstants.CONTENT_PAGE);
}
public void addFragments(Fragment fragment, boolean animate,
boolean addToBackStack, String tag) {
FragmentManager manager = getSupportFragmentManager();
FragmentTransaction ft = manager.beginTransaction();
if (animate) {
ft.setCustomAnimations(R.anim.fragment_from_right,
R.anim.fragment_from_left, R.anim.fragment_from_right,
R.anim.fragment_from_left);
}
if (addToBackStack) {
ft.addToBackStack(tag);
}
ft.replace(R.id.content_frame, fragment);
ft.commit();
}
}
For Fragment:
public class Sample extends Fragment {
@Override
public void onCreate(Bundle savedInstanceState) {
// TODO Auto-generated method stub
super.onCreate(savedInstanceState);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// TODO Auto-generated method stub
View view = inflater.inflate(R.layout.page, container, false);
return view;
}
}
After generate a Navigation Drawer Activity
by File->New->Activity->Navigation Drawer Activity
, here are 3 steps
First, go to app_bar_main.xml
then
replace
<include layout="@layout/content_main"/>
by
<FrameLayout
android:id="@+id/frame_content"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior"
/>
Second, go to MainActivity
-> onNavigationItemSelected
then modify it like
public boolean onNavigationItemSelected(@NonNull MenuItem item) {
int id = item.getItemId();
Fragment fragment = null;
if (id == R.id.nav_camera) {
fragment = CameraFragment.newInstance();
} else if (id == R.id.nav_gallery) {
fragment = GalleryFragment.newInstance();
} else if (id == R.id.nav_slideshow) {
fragment = SlideShowFragment.newInstance();
}
FragmentManager fragmentManager = getSupportFragmentManager();
fragmentManager.beginTransaction().replace(R.id.frame_content, fragment).commit();
setTitle(item.getTitle());
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
drawer.closeDrawer(GravityCompat.START);
return true;
}
Finally, create Fragment
class, example a Fragment
public class CameraFragment extends Fragment{
public static CameraFragment newInstance() {
Bundle args = new Bundle();
CameraFragment fragment = new CameraFragment();
fragment.setArguments(args);
return fragment;
}
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container,
@Nullable Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_camera, null, false);
return rootView;
}
}
Demo project
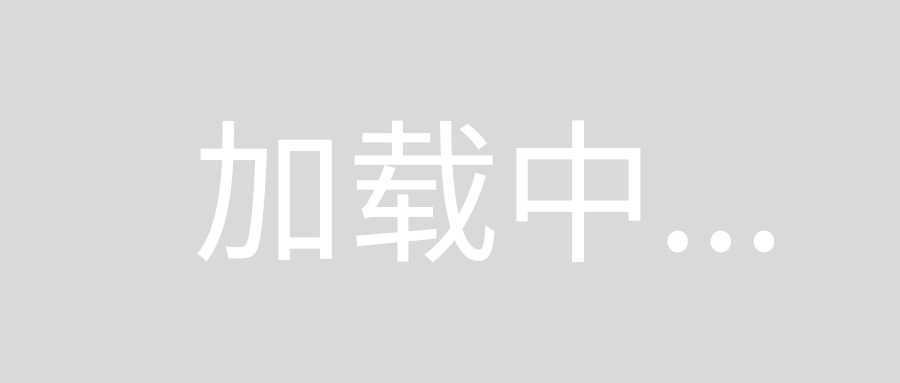