I want to get the YouTube video ID from YouTube embed code using preg_match or regex. For a example
<iframe width="560" height="315" src="//www.youtube.com/embed/0gugBiEkLwU?rel=0" frameborder="0" allowfullscreen></iframe>
I want to take the ID 0gugBiEkLwU
Can anyone tell me how to do this. Really appropriate your help.
Using this pattern with a capturing group should give you the string you want:
d\/(\w+)\?rel=\d+"
example: https://regex101.com/r/kH5kA7/1
You can use :
src="\/\/(?:https?:\/\/)?.*\/(.*?)\?rel=\d*"
Check Demo Here
Explanation :
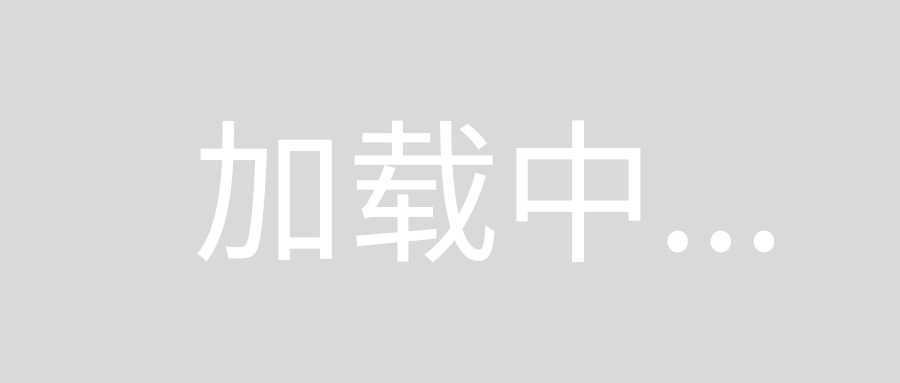
I know this is pretty late, but I came up with something for people who might still be looking.
Since not all Youtube iframe src attributes end in "?rel=", and can sometimes end in another query string or end with a double quote, you can use:
/embed\/([\w+\-+]+)[\"\?]/
This captures anything after "/embed/" and before the ending double-quote/query string. The selection can include any letter, number, underscore and hyphen.
Here's a demo with multiple examples: https://regex101.com/r/eW7rC1/1
The below function will extract the youtube video id from teh all format of youtube urls,
function getYoutubeVideoId($iframeCode) {
// Extract video url from embed code
return preg_replace_callback('/<iframe\s+.*?\s+src=(".*?").*?<\/iframe>/', function ($matches) {
// Remove quotes
$youtubeUrl = $matches[1];
$youtubeUrl = trim($youtubeUrl, '"');
$youtubeUrl = trim($youtubeUrl, "'");
// Extract id
preg_match("/^(?:http(?:s)?:\/\/)?(?:www\.)?(?:m\.)?(?:youtu\.be\/|youtube\.com\/(?:(?:watch)?\?(?:.*&)?v(?:i)?=|(?:embed|v|vi|user)\/))([^\?&\"'>]+)/", $youtubeUrl, $videoId);
return $youtubeVideoId = isset($videoId[1]) ? $videoId[1] : "";
}, $iframeCode);
}
$iframeCode = '<iframe width="560" height="315" src="http://www.youtube.com/embed/0gugBiEkLwU?rel=0" frameborder="0" allowfullscreen></iframe>';
// Returns youtube video id
echo getYoutubeVideoId($iframeCode);