I want to add a floating close (x) button at the corner of an UIModalPresentationPageSheet
View. The effect is like below:
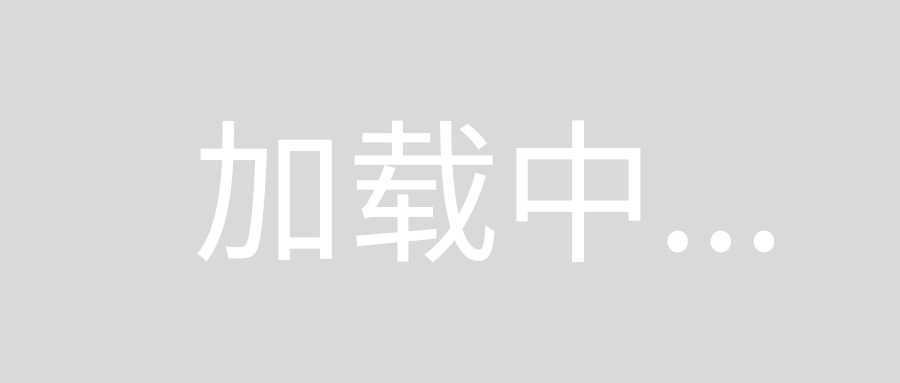
But adding it to the parent view makes it appear behind the Page Sheet (and also impossible to tap) and adding it to the Page Sheet will make part of it hidden, since it's out of the view area.
Is there any better solution?
Any suggestions are appreciated.
You can try and add it to the the topmost application window:
add.modalPresentationStyle = UIModalPresentationPageSheet;
[self presentViewController:add animated:YES completion:^{
[[[[UIApplication sharedApplication] windows] lastObject] addSubview:button];
}];
This should give you the right view to allow the button to appear on top of the modal and receive touch events.
--edit--
To position the button you can try something like this:
self.modalPresentationStyle = UIModalPresentationFormSheet;
add.modalTransitionStyle = UIModalTransitionStyleCoverVertical;
[self presentViewController:pvc animated:YES completion:^{
CGRect parentView = pvc.view.superview.frame;
CGRect buttonFrame = button.frame;
buttonFrame.origin = CGPointMake(parentView.origin.x - buttonFrame.size.width/2.0f, parentView.origin.y - buttonFrame.size.height/2.0f);
[button setFrame:buttonFrame];
[[[[UIApplication sharedApplication] windows] lastObject] addSubview:button];
}];
This will get the frame of the modalView that has been presented. You can then set your button's origin to be offset and set the adjusted frame.
See if this works:
myPresentedView.clipsToBounds = NO;
That will allow the button to draw itself beyond it's views. One drawback to this is that the touches will not land beyond the views bounds, so try not to set the button too far away.