I would like to call a POST Method(Magento REST API) in Retrofit with a JSON data(provide JSON as JsonObject). For that, I call as follow from the postman and work fine for me.
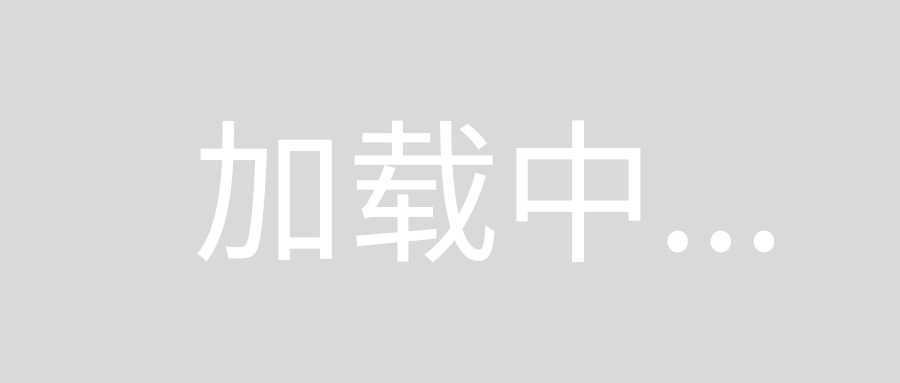
I have done the android parts as follow,
API INTERFACE
public interface APIService {
@POST("seq/restapi/checkpassword")
@Headers({
"Content-Type: application/json;charset=utf-8",
"Accept: application/json;charset=utf-8",
"Cache-Control: max-age=640000"
})
Call<Post> savePost(
@Body JSONObject jsonObject
);}
APIUtility class as
public class ApiUtils {
private ApiUtils() {
}
public static final String BASE_URL = "http://xx.xxxx.xxx.xxx/api/rest/";
public static APIService getAPIService() {
return RetrofitClient.getClient(BASE_URL).create(APIService.class);
}
}
RetrofitClient class as
private static Retrofit retrofit = null;
public static Retrofit getClient(String baseUrl) {
if (retrofit==null) {
retrofit = new Retrofit.Builder()
.baseUrl(baseUrl)
.addConverterFactory(GsonConverterFactory.create())
.build();
}
return retrofit;
}
And finally, call the function as following
public void sendPost(JSONObject jsonObject) {
Log.e("TEST", "****************************************** jsonObject" + jsonObject);
mAPIService.savePost(jsonObject).enqueue(new Callback<Post>() {
@Override
public void onResponse(Call<Post> call, Response<Post> response) {
if (response.isSuccessful()) {
}
}
@Override
public void onFailure(Call<Post> call, Throwable t) {
}
});
}
CallRetrofit API with POST method with body.
Change your code to something like this, GsonConverterFactory converts a User object to json itself.
public interface APIService {
@POST("seq/restapi/checkpassword")
@Headers({
"Content-Type: application/json;charset=utf-8",
"Accept: application/json;charset=utf-8",
"Cache-Control: max-age=640000"
})
Call<Post> savePost(@Body User user);
}
This is User Class:
public class User {
private String username;
private String password;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
I think the GsonConverterFactory
you've set up for all calls converts your JSONObject to json. That's why your call's body may look quite different than you think. This is the result of gson.toJson(new JSONObject())
:
{ "nameValuePairs": {} }
Try changing the object you send to this:
public class Credentials {
private String username;
private String password;
...
}
and then update your call
public interface APIService {
@POST("seq/restapi/checkpassword")
@Headers({
"Content-Type: application/json;charset=utf-8",
"Accept: application/json;charset=utf-8",
"Cache-Control: max-age=640000"
})
Call<Post> savePost(
@Body Credentials credentials
);}
To ensure your call is correct, call
Gson gson = new Gson();
gson.toJson(new Credentials("test_username", "test_password");
Try this
1.APIInterface.java
public interface APIInterface {
/*Login*/
@POST("users/login")
Call<UserResponse> savePost(@HeaderMap Map<String, String> header, @Body UserModel loginRequest);
}
2.ApiClient.java
public class ApiClient {
public static final String BASE_URL = "baseurl";
private static Retrofit retrofit = null;
public static Retrofit getClient() {
if (retrofit == null) {
HttpLoggingInterceptor interceptor = new HttpLoggingInterceptor();
interceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient client = new OkHttpClient.Builder().addInterceptor(interceptor)
.connectTimeout(30, TimeUnit.MINUTES)
.readTimeout(30, TimeUnit.MINUTES)
.build();
retrofit = new Retrofit.Builder()
.baseUrl(BASE_URL)
.client(client)
.addConverterFactory(GsonConverterFactory.create())
.build();
}
return retrofit;
}
}
API call
public void sendPost(JSONObject jsonObject) {
LoginRequestModel loginRequest = new LoginRequestModel(userName, userPassword);
Map<String, String> header = new HashMap<>();
header.put("Content-Type", "application/json");
APIInterface appInterface = ApiClient.getClient().create(APIInterface.class);
System.out.println("Final" + new Gson().toJson(loginRequest));
Call<LoginResponseModel> call = appInterface.savePost(header, loginRequest);
call.enqueue(new Callback<LoginResponseModel>() {
@Override
public void onResponse(Call<LoginResponseModel> call, Response<UserModel> response) {
hideProgressDialog();
if (response.body()!=null) {
} else {
Toast.makeText(mContext, "Invalid credentials", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onFailure(Call<LoginResponseModel> call, Throwable t) {
t.printStackTrace();
}
});
}
Dependencies in Gradle file
compile 'com.squareup.retrofit2:retrofit:2.3.0'
compile 'com.google.code.gson:gson:2.8.2'
compile 'com.squareup.retrofit2:converter-gson:2.3.0'
compile 'com.squareup.okhttp3:okhttp:3.9.0'
compile 'com.squareup.okhttp3:logging-interceptor:3.9.0'
5.User Model
public class UserModel {
@SerializedName("usename")
@Expose
String userEmail;
@SerializedName("password")
@Expose
String userPassword;
public UserModel(String userEmail, String userPassword) {
this.userEmail = userEmail;
this.userPassword = userPassword;
}
}