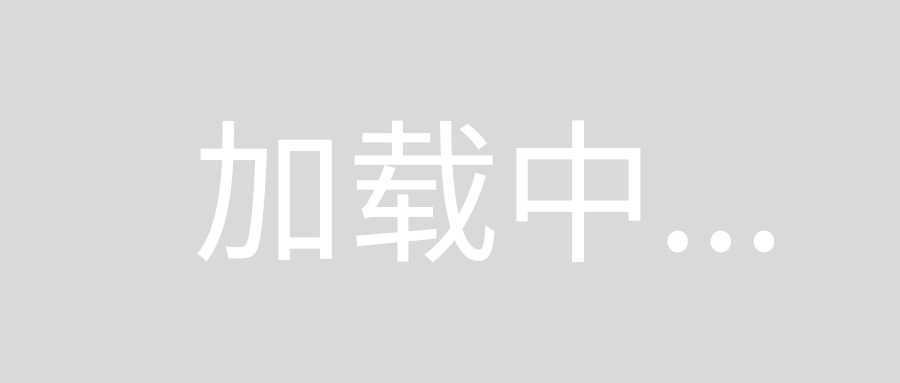
This image demonstrats what I need to do. I need the dropdown menu to always stay on the left next to the overflow menu icon
How can i do this?
My styles.xml
<?xml version="1.0" encoding="utf-8"?>
<resources xmlns:android="http://schemas.android.com/apk/res/android">
<!-- Variation on the Holo Light theme that styles the Action Bar -->
<style name="Theme.AndroidDevelopers" parent="Theme.Sherlock.Light">
<item name="android:actionBarItemBackground">@drawable/ad_selectable_background</item>
<item name="actionBarItemBackground">@drawable/ad_selectable_background</item>
<item name="android:popupMenuStyle">@style/MyPopupMenu</item>
<item name="popupMenuStyle">@style/MyPopupMenu</item>
<item name="android:dropDownListViewStyle">@style/MyDropDownListView</item>
<item name="dropDownListViewStyle">@style/MyDropDownListView</item>
<item name="android:actionBarTabStyle">@style/MyActionBarTabStyle</item>
<item name="actionBarTabStyle">@style/MyActionBarTabStyle</item>
<item name="android:actionDropDownStyle">@style/MyDropDownNav</item>
<item name="actionDropDownStyle">@style/MyDropDownNav</item>
<item name="android:listChoiceIndicatorMultiple">@drawable/ad_btn_check_holo_light</item>
<item name="android:listChoiceIndicatorSingle">@drawable/ad_btn_radio_holo_light</item>
<!-- <item name="android:actionOverflowButtonStyle">@style/MyOverflowButton</item> -->
</style>
<!-- style the overflow menu -->
<style name="MyPopupMenu" parent="Widget.Sherlock.Light.PopupMenu">
<item name="android:popupBackground">@drawable/ad_menu_dropdown_panel_holo_light</item>
</style>
<!-- style the items within the overflow menu -->
<style name="MyDropDownListView" parent="Widget.Sherlock.ListView.DropDown">
<item name="android:listSelector">@drawable/ad_selectable_background</item>
</style>
<!-- style for the tabs -->
<style name="MyActionBarTabStyle" parent="Widget.Sherlock.Light.ActionBar.TabBar">
<item name="android:background">@drawable/actionbar_tab_bg</item>
</style>
<!-- style the list navigation -->
<style name="MyDropDownNav" parent="Widget.Sherlock.Light.Spinner.DropDown.ActionBar">
<item name="android:background">@drawable/ad_spinner_background_holo_light</item>
<item name="android:popupBackground">@drawable/ad_menu_dropdown_panel_holo_light</item>
<item name="android:dropDownSelector">@drawable/ad_selectable_background</item>
</style>
<!--
the following can be used to style the overflow menu button
only do this if you have an *extremely* good reason to!!
-->
<!--
<style name="MyOverflowButton" parent="Widget.Sherlock.ActionButton.Overflow">
<item name="android:src">@drawable/ic_menu_view</item>
<item name="android:background">@drawable/action_button_background</item>
</style>
-->
<style name="customRatingBar" parent="@android:style/Widget.RatingBar">
<item name="android:progressDrawable" >@drawable/custom_ratingbar</item>
<item name="android:indeterminateDrawable">@drawable/money_off</item>
<item name="android:minHeight">10dip</item>
<item name="android:maxHeight">15dip</item>
<item name="android:scaleType">centerInside</item>
</style>
</resources>
As an example for implementing it as Action view, "Emanuel Moecklin" is the owner of following Code
ActionBar actionBar = getSupportActionBar();
final Context context = actionBar.getThemedContext();
final String[] entries = new String[] { "Entry 1", "Entry 2", "Entry 3" };
ArrayAdapter<String> adapter = new ArrayAdapter<String>(context,
R.layout.sherlock_spinner_item, entries);
adapter.setDropDownViewResource(R.layout.sherlock_spinner_dropdown_item);
// create ICS spinner
IcsSpinner spinner = new IcsSpinner(this, null,
R.attr.actionDropDownStyle);
spinner.setAdapter(adapter);
spinner.setOnItemSelectedListener(new OnItemSelectedListener() {
@Override
public void onItemSelected(IcsAdapterView<?> parent, View view,
int position, long id) {
Toast.makeText(context, "Item selected: " + entries[position],
Toast.LENGTH_SHORT).show();
}
@Override
public void onNothingSelected(IcsAdapterView<?> parent) {
}
});
// configure custom view
IcsLinearLayout listNavLayout = (IcsLinearLayout) getLayoutInflater()
.inflate(R.layout.abs__action_bar_tab_bar_view, null);
LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(
LayoutParams.WRAP_CONTENT, LayoutParams.MATCH_PARENT);
params.gravity = Gravity.CENTER;
listNavLayout.addView(spinner, params);
listNavLayout.setGravity(Gravity.RIGHT); // <-- align the spinner to the
// right
// configure action bar
actionBar.setCustomView(listNavLayout, new ActionBar.LayoutParams(
Gravity.RIGHT));
actionBar.setDisplayShowCustomEnabled(true);
As far as I know, it is impossible to move Action bar features from the left to the right. You can, however, add a Spinner
as an Action View and customize it so that it looks and behaves exactly like the Spinner
from the Action bar list navigation.
Add UI components at the Right side of Actionbar using ToolBar:
Check this link for more details of ToolBar.
Screenshot attached.
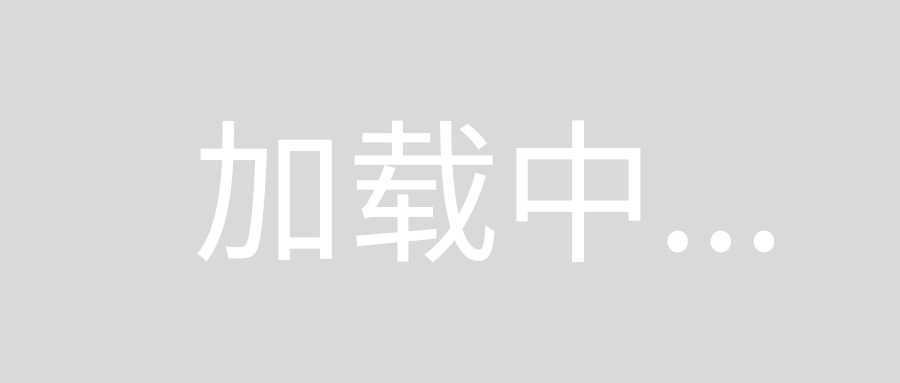
toolbar.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.Toolbar
style="@style/ToolBarStyle"
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="?attr/colorPrimary"
android:minHeight="@dimen/abc_action_bar_default_height_material">
<RelativeLayout
android:id="@+id/rlToolBarMain"
android:layout_width="wrap_content"
android:layout_marginRight="10dp"
android:layout_marginLeft="10dp"
android:layout_height="wrap_content"
android:layout_gravity="right|center_vertical">
</RelativeLayout>
</android.support.v7.widget.Toolbar>
Style for Application
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.NoActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/myPrimaryColor</item>
<item name="colorPrimaryDark">@color/myPrimaryDarkColor</item>
<item name="colorAccent">@color/myAccentColor</item>
<item name="android:textColorPrimary">@color/myTextPrimaryColor</item>
<item name="drawerArrowStyle">@style/DrawerArrowStyle</item>
<item name="android:windowBackground">@color/myWindowBackground</item>
</style>
<style name="DrawerArrowStyle" parent="Widget.AppCompat.DrawerArrowToggle">
<item name="spinBars">true</item>
<item name="color">@android:color/white</item>
</style>
<style name="ToolBarStyle" parent="">
<item name="popupTheme">@style/ThemeOverlay.AppCompat.Light</item>
<item name="theme">@style/ThemeOverlay.AppCompat.Dark.ActionBar</item>
</style>
</resources>
Include xml in activity_main.xml
<include
android:id="@+id/toolbar_actionbar"
layout="@layout/toolbar_default"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
Your Activity must be extends AppCompatActivity
on onCreate of Activity
Toolbar mToolbar = (Toolbar) findViewById(R.id.toolbar_actionbar);
setSupportActionBar(mToolbar);
getSupportActionBar().setDisplayShowHomeEnabled(true);
on onCreateView of Fragment
Toolbar mToolBar = (Toolbar) getActivity().findViewById(R.id.toolbar_actionbar);
RelativeLayout rlToolBarMain = (RelativeLayout)mToolBar.findViewById(R.id.rlToolBarMain);
Spinner mSpinner = new Spinner(getActivity());
String[] frags = new String[]{
"category1",
"category2",
"category3",
};
ArrayAdapter<String> arrayAdapter = new ArrayAdapter<String>(getActivity(),android.R.layout.simple_list_item_1,frags);
mSpinner.setAdapter(arrayAdapter);
rlToolBarMain.addView(mSpinner);
Done
Just try this ,
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
app:layout_scrollFlags="scroll|enterAlways"
app:popupTheme="@style/AppTheme.PopupOverlay"
app:title="@string/app_name">
<Spinner
android:id="@+id/Method"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_gravity="right|center_vertical"
android:layout_weight="1"
android:gravity="right|center_vertical" />
</android.support.v7.widget.Toolbar>