How should I require
the jquery
in node
if I use it in multiple modules? Should I define it as a global or should I just use the require('jquery
)` in every module I need it?
I am getting an error when trying to use the package.
TypeError: Object function ( w ) {
if ( !w.document ) {
throw new Error( "jQuery requires a window with a document" );
}
return factory( w );
} has no method 'isArray'
It looks like a bug in the current release as it should not check if I am running it in a browser according to the official documentation. This issue is also mentioned in another post. It works with version 1.8.3
as mentioned in one of the answers.
To use jquery in node, you need to have two separate node package installations.
- jquery
- jsdom to create a dummy window object which jquery can use.
Installation:
npm install jquery
npm install jsdom
In code:
var jsdom = require("jsdom").jsdom;
global.$ = require('jquery/dist/jquery')(jsdom().createWindow());
Or, with newer versions of jsdom:
require("jsdom").env("", function(err, window) {
if (err) {
console.error(err);
return;
}
var $ = require("jquery")(window);
});
Using global.$ will make the jquery object($) available globally in your project.
You can use as below to handle as usual:
var $;
$ = require('jquery');
$('.tag').click(function() {
return console.log('clicked');
});
If you are using jQuery for client purposes this is how I did it and I am on MEAN stack using jade templating engine.
For example; Let's say I have simple layout.jade like this
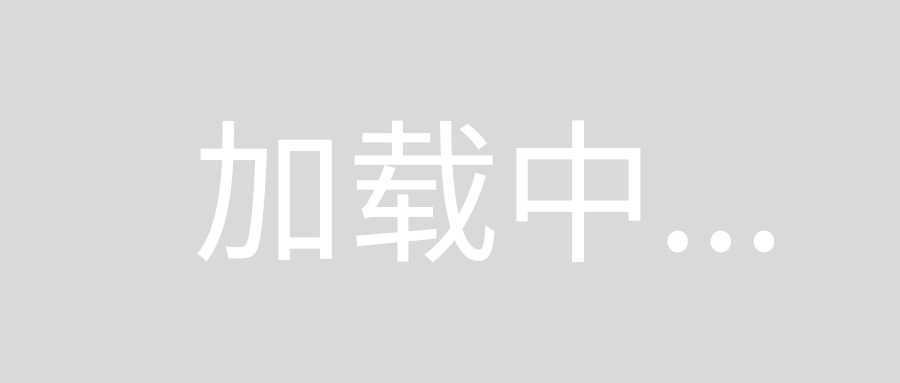
I will be using all jquery functionality on a single page app via index.jade and I will be loading like this.
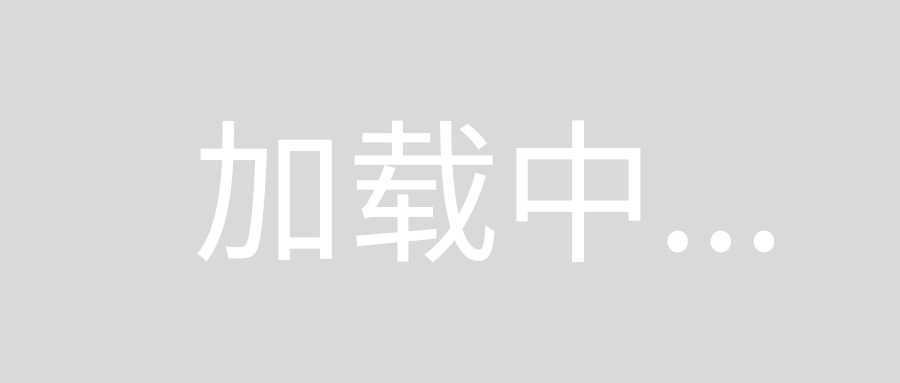
```
extends layout
block content
script(type='text/javascript', src="http://code.jquery.com/jquery-1.9.1.min.js")
div#container(style="min-width: 500px; height: 500px; margin: 0 auto")
script.
if (jQuery) {
console.log("jQuery library is loaded!");
} else {
console.log("jQuery library is not found!");
}
$(function () {
});
h1= title
p Welcome to #{title}
```