可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
In the following code below, how do I dismiss the alert box? I don't want to cause a memory leak. I tried the .dismiss() on alertDialog, but that didn't work...
Thanks
// User pressed the stop button
public void StopMsg_button_action(View view){
final EditText password_input = new EditText(this); // create an text input field
password_input.setHint("Enter Password"); // put a hint in it
password_input.setInputType(InputType.TYPE_CLASS_TEXT | InputType.TYPE_TEXT_VARIATION_PASSWORD); // change it to password type
AlertDialog.Builder alertDialog = new Builder(this); // create an alert box
alertDialog.setTitle("Enter Password"); // set the title
alertDialog.setView(password_input); // insert the password text field in the alert box
alertDialog.setPositiveButton("OK", new DialogInterface.OnClickListener() { // define the 'OK' button
public void onClick(DialogInterface dialog, int which) {
String entered_password = password_input.getText().toString();
if (entered_password.equals(my_password)) {
locManager.removeUpdates(locListener); // stop listening for GPS coordinates
startActivity(new Intent(Emergency_1Activity.this,Main_MenuActivity.class)); // go to main menu
} else {
alert("Incorrect Password");
}
}
});
alertDialog.setNeutralButton("Cancel", new DialogInterface.OnClickListener() { // define the 'Cancel' button
public void onClick(DialogInterface dialog, int which) {
}
});
alertDialog.show(); // show the alert box
}
回答1:
What didn't work about dismiss()?
You should be able to use either Dialog.dismiss(), or Dialog.cancel()
alertDialog.setNeutralButton("Cancel", new DialogInterface.OnClickListener() { // define the 'Cancel' button
public void onClick(DialogInterface dialog, int which) {
//Either of the following two lines should work.
dialog.cancel();
//dialog.dismiss();
}
});
回答2:
With the following code you can show a ListBox AlertDialog and when you press on a item you dismiss the Dialog. The order of code is importan.
AlertDialog.Builder alertDialog = new AlertDialog.Builder(mContext);
String names[] ={"A","B","C","D"};
ArrayAdapter<String> adapter = new ArrayAdapter<String>(mContext,android.R.layout.simple_list_item_1,names);
LayoutInflater inflater = getLayoutInflater();
View convertView = (View)inflater.inflate(R.layout.list_layout, null);
ListView lv = (ListView) convertView.findViewById(R.id.listView1);
lv.setAdapter(adapter);
alertDialog.setView(convertView);
alertDialog.setTitle("List");
final AlertDialog ad = alertDialog.show();
lv.setOnItemClickListener(new AdapterView.OnItemClickListener()
{
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id)
{
//Toast.makeText(mContext, position, Toast.LENGTH_LONG).show();
ad.dismiss();
}
});
回答3:
The code is very simple:
final AlertDialog show = alertDialog.show();
finally in the action of button for example:
show.dismiss();
For example with a custom alertdialog:
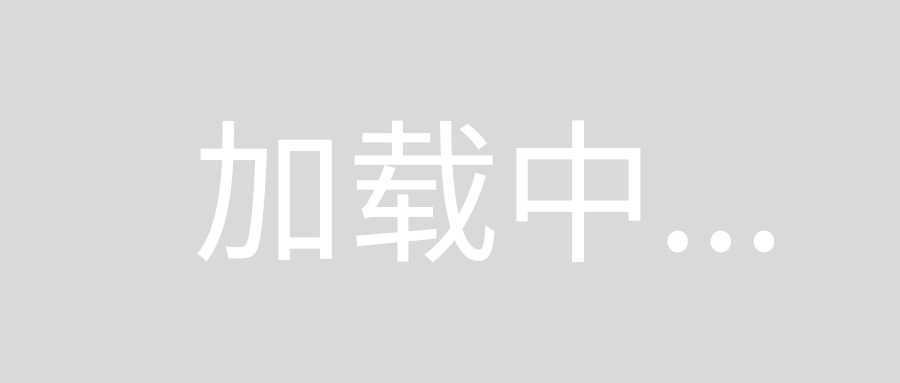
Code on java, you could create a Object AlertDialog:
public class ViewAlertRating {
Context context;
public ViewAlertRating(Context context) {
this.context = context;
}
public void showAlert(){
AlertDialog.Builder alertDialog = new AlertDialog.Builder(context);
LayoutInflater inflater = ((Activity) context).getLayoutInflater();
View alertView = inflater.inflate(R.layout.layout_test, null);
alertDialog.setView(alertView);
final AlertDialog show = alertDialog.show();
Button alertButton = (Button) alertView.findViewById(R.id.btn_test);
alertButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
show.dismiss();
}
});
}
}
Code XML layout_test.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Valoración"
android:id="@+id/text_test1"
android:textSize="20sp"
android:textColor="#ffffffff"
android:layout_centerHorizontal="true"
android:gravity="center_horizontal"
android:textStyle="bold"
android:paddingTop="10dp"
android:paddingBottom="10dp"
android:background="#ff37dabb"
android:paddingLeft="20dp"
android:paddingRight="20dp" />
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingLeft="20dp"
android:paddingRight="20dp"
android:layout_marginTop="15dp">
<EditText
android:layout_width="match_parent"
android:layout_height="120dp"
android:id="@+id/edit_test"
android:hint="Descripción"
android:textColor="#aa000000"
android:paddingLeft="10dp"
android:paddingRight="10dp"
android:textColorHint="#aa72777a"
android:gravity="top" />
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal"
android:paddingTop="10dp"
android:paddingLeft="15dp"
android:paddingRight="15dp"
android:paddingBottom="15dp" >
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:weightSum="1.00"
android:gravity="right" >
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Enviar"
android:id="@+id/btn_test"
android:gravity="center_vertical|center_horizontal"
android:textColor="#ffffffff"
android:background="@drawable/btn_flat_blue_selector" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
</LinearLayout>
finally, call on Activity:
ViewAlertRating alertRating = new ViewAlertRating(this);
alertRating.showAlert();
回答4:
You must use this way if you don't want to put any buttons and have custom layout in which you have say textview and you want to dismiss alert dialog upon clicking of that textview:
AlertDialog alertDialog = builder.show();
then check
if(alertDialog != null && alertDialog.isShowing()){
alertDialog.dismiss();
}
This is also true if you want to dismiss it somewhere else in your activity after checking some condition.
回答5:
This method demos the code that's needed .. from Namrata's earlier answer
@SuppressLint("InflateParams")
private static void showDialog(Activity activity, int layoutId)
{
LayoutInflater inflater = activity.getLayoutInflater();
View dialoglayout = inflater.inflate(layoutId, null);
AlertDialog.Builder builder = new AlertDialog.Builder(activity);
builder.setView(dialoglayout);
CustomDialog.doCustomLogic(activity, layoutId, dialoglayout);
final AlertDialog alertDialog = builder.show();
// caller assumes there will be a btn_close element present
Button closeNowBtn = (Button) dialoglayout.findViewById(R.id.btn_close);
closeNowBtn.setOnClickListener(new View.OnClickListener()
{
public void onClick(View v)
{
alertDialog.dismiss();
}
});
}
回答6:
Just override create method and save the dialog instance. Then you can call dismiss
@Override
public AlertDialog create() {
this.dialog = super.create();
return this.dialog;
}
Somewhere at the code:
dialog.dismiss();
回答7:
Instead of alertDialog.setNeutralButton
just use alertDialog.setNegativeButton
. Use dialog.cancel()
, because dialog.dismiss()
is not a method available for Alert dialogs.
回答8:
To dismiss or cancel AlertDialog.Builder
dialog.setNegativeButton("إلغاء", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
dialogInterface.dismiss()
}
});
You have to call dismiss()
on the dialog interface.
回答9:
i tried this and worked!
.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
alertDialog.setCancelable(true);
}
});