I have a button search that located in the right side of the navigation.
This is my code:
UIButton *btnSearch = [UIButton buttonWithType:UIButtonTypeCustom];
btnSearch.frame = CGRectMake(0, 0, 22, 22);
[btnSearch setImage:[UIImage imageNamed:@"search_btn.png"] forState:UIControlStateNormal];
[btnSearch addTarget:self action:@selector(showSearch:) forControlEvents:UIControlEventTouchUpInside];
UIBarButtonItem *searchItem = [[UIBarButtonItem alloc] initWithCustomView:_btnSearch];
self.navigationItem.rightBarButtonItems = searchItem ;
This is how it's look.
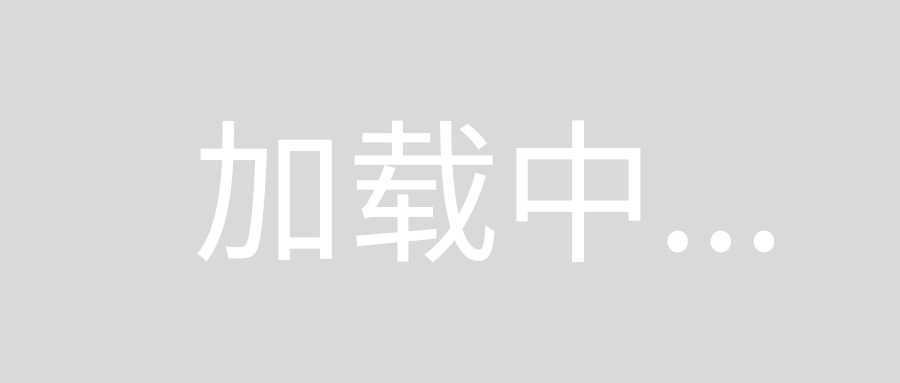
And I want to display search bar after clicked on the search button, and close it after clicked on cancel and then show the Navigationbar back, but I don't know how to code it.
- (IBAction)showSearch:(id)sender{
???
}
This what I want.
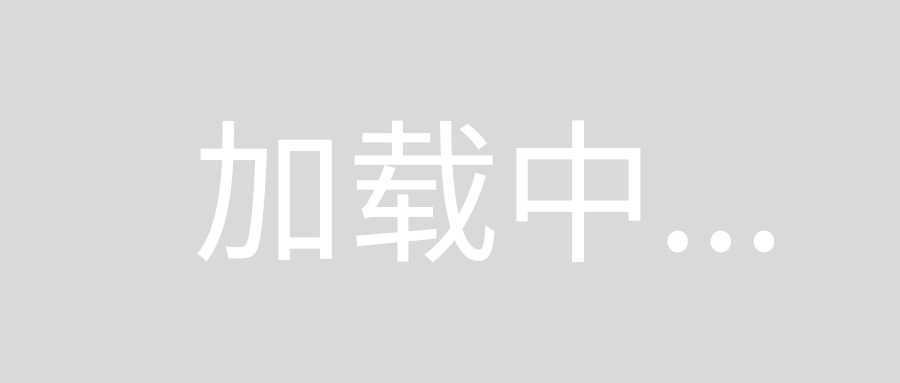
Please help or provide some sample code. I really need it.
Thank for reading.
Add a property UISearchBar *mySearchBar
to your viewController as
@property(nonatomic, retain) UISearchBar *mySearchBar;
Conform to UISearchBarDelegate
as
@interface HomeViewController () <UISearchBarDelegate>
...
...
@end
Then implement the showSearch
method as
-(void)showSearch:(id)sender {
if(!mySearchBar) {
mySearchBar = [[UISearchBar alloc] init];
[mySearchBar setFrame:CGRectMake(0, 0, self.view.frame.size.width, 55)];
[mySearchBar setShowsCancelButton:YES animated:YES];
[self.view addSubview: mySearchBar];
mySearchBar.delegate = self;
self.navigationController.navigationBarHidden = YES;
}else {
searchBar.alpha = 1.0;
self.navigationController.navigationBarHidden = YES;
}
Then implement the search bar delegate method as :
- (void)searchBarCancelButtonClicked:(UISearchBar *) searchBar {
self.navigationController.navigationBarHidden = NO;
[mySearchBar setAlpha:0.0];
}
Hope you have got the idea by doing this. Also you can add it directly to navigation controller, itself, if you want & then play with Showing/ hiding the searchBar
alone.
You can add it to navigation controller as just initialize the mysearchBar
& add it to navigationBar
as :
UIBarButtonItem *searchBarItem = [[UIBarButtonItem alloc] initWithCustomView:mySearchBar];
self.navigationItem.rightBarButtonItem = searchBarItem;