How to display icon with back arrow in android toolbar like WhatsApp ?
I use below code to set back arrow & icon in toolbar.
toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
getSupportActionBar().setHomeButtonEnabled(true);
toolbar.setLogo(icon);
but i got result as below image.
I want icon immediate next after back arrow. I don't want any gap between back arrow & icon like below image of WhatsApp.
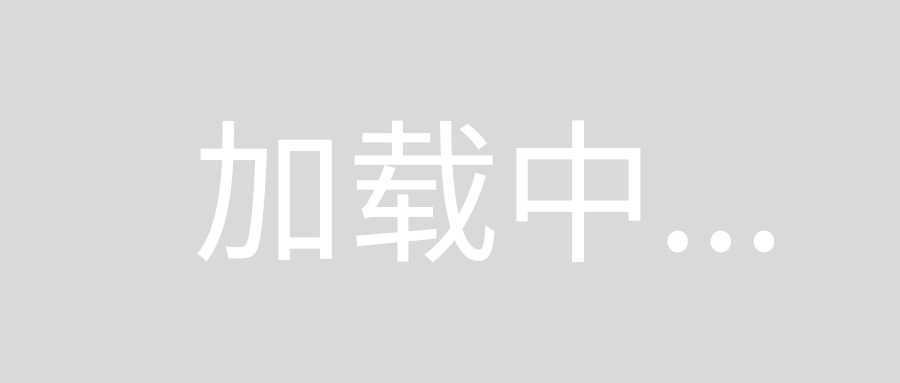
How to set icon with back arrow in toolbar like WhatsApp ?
As far as I Know,
WhatsApp is not using App-compat Support Library Toolbar,
Whatsapp is setting the Custom Action Bar using
actionBar.setCustomView(R.layout.conversation_actionbar);
Attached is the conversation_actionbar.xml , Whatsapp is using
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@id/custom_view"
android:layout_width="fill_parent"
android:layout_height="?actionBarSize"
android:clipChildren="false" >
<LinearLayout
android:id="@id/back"
style="@style/ActionBarButtonStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_centerVertical="true"
android:contentDescription="@string/abc_action_bar_up_description"
android:enabled="false"
android:orientation="horizontal"
android:padding="@dimen/abc_action_bar_default_padding_material" >
<ImageView
android:id="@id/up"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="left|center"
android:scaleType="center"
android:src="?homeAsUpIndicator" />
<FrameLayout
android:id="@id/conversation_contact_photo_frame"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="left|center"
android:layout_marginRight="0.0dip" >
<ImageView
android:id="@id/conversation_contact_photo"
android:layout_width="35.0dip"
android:layout_height="35.0dip"
android:scaleType="fitCenter" />
<View
android:id="@id/transition_start"
android:layout_width="35.0dip"
android:layout_height="35.0dip" />
<ProgressBar
android:id="@id/change_photo_progress"
style="?android:attr/progressBarStyleSmallInverse"
android:layout_width="35.0dip"
android:layout_height="35.0dip"
android:layout_gravity="center"
android:visibility="gone" />
</FrameLayout>
</LinearLayout>
<LinearLayout
android:id="@id/conversation_contact"
style="@style/ActionBarButtonStyle"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_centerVertical="true"
android:layout_toRightOf="@id/back"
android:clickable="true"
android:clipChildren="false"
android:orientation="vertical"
android:paddingBottom="2.0dip"
android:paddingLeft="4.0dip"
android:paddingRight="0.0dip"
android:paddingTop="0.0dip" >
<com.whatsapp.TextEmojiLabel
android:id="@id/conversation_contact_name"
style="@style/Theme.ActionBar.TitleTextStyle.Condensed"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="left"
android:ellipsize="end"
android:gravity="left"
android:lines="1"
android:scrollHorizontally="true"
android:singleLine="true" />
<LinearLayout
android:id="@id/conversation_contact_status_holder"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_gravity="left"
android:clipChildren="false"
android:clipToPadding="false"
android:orientation="horizontal" >
<TextView
android:id="@id/conversation_contact_status_prefix"
style="@style/Theme.ActionBar.SubtitleTextStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="left"
android:lines="1"
android:paddingRight="3.5sp"
android:singleLine="true"
android:text="@string/conversation_last_seen"
android:visibility="gone" />
<TextView
android:id="@id/conversation_contact_status"
style="@style/Theme.ActionBar.SubtitleTextStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="left"
android:ellipsize="end"
android:lines="1"
android:singleLine="true" />
<View
android:layout_width="0.0dip"
android:layout_height="1.0dip"
android:layout_weight="1.0" />
</LinearLayout>
</LinearLayout>
But I would suggest you to follow Materail Design Rules and use ToolBar of App-compat Support Library,
You can get the following result as shown in the Picture below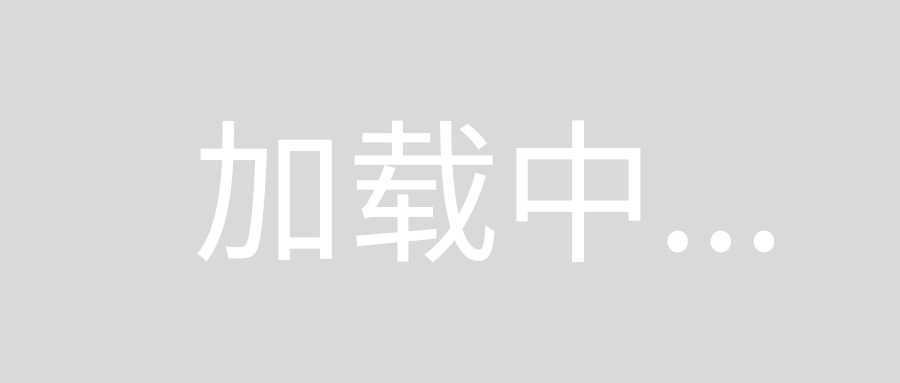
By using following code
your_activity.xml:
<android.support.design.widget.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar_chats"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar"
app:popupTheme="@style/ThemeOverlay.AppCompat.Light">
<include layout="@layout/toolbar_conversation"/>
</android.support.v7.widget.Toolbar>
</android.support.design.widget.AppBarLayout>
toolbar_conversation.xml:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:background="?attr/selectableItemBackgroundBorderless"
android:layout_width="fill_parent"
android:layout_height="?actionBarSize"
>
<!-- android:background="?attr/selectableItemBackgroundBorderless" will cause this Custom View to make ripple effect -->
<LinearLayout
android:id="@+id/conversation_image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_centerVertical="true"
android:contentDescription="@string/abc_action_bar_up_description"
android:orientation="horizontal">
<ImageView
android:id="@+id/conversation_contact_photo"
android:layout_width="35.0dip"
android:layout_height="35.0dip"
android:src="@drawable/icon"
android:scaleType="fitCenter" />
</LinearLayout>
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_centerVertical="true"
android:layout_toRightOf="@id/conversation_image"
android:orientation="vertical"
android:paddingBottom="2.0dip"
android:paddingLeft="4.0dip"
android:paddingRight="0.0dip"
android:paddingTop="0.0dip" >
<TextView
android:id="@+id/action_bar_title_1"
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_gravity="center_vertical"
android:layout_marginLeft="6dp"
android:layout_weight="0.6"
android:ellipsize="end"
android:gravity="center_vertical"
android:maxLines="1"
android:textSize="18sp"
android:text="shanraisshan"
android:textStyle="bold" />
<TextView
android:id="@+id/action_bar_title_2"
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_marginLeft="6dp"
android:layout_weight="0.4"
android:ellipsize="end"
android:text="last seen 1 hour ago"
android:maxLines="1"
android:textSize="12sp" />
</LinearLayout>
Activity.java
final Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar_chats);
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
toolbar.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Log.e("Toolbar","Clicked");
}
});
Just wait for it, Whatsapp will shift to App-compat Support Library in future.
getSupportActionBar().setDisplayShowHomeEnabled(true);
along with
getSupportActionBar().setIcon(R.drawable.ic_launcher);
It seems like you need to set three of the action bar related values to display the icon:
actionBar.setDisplayHomeAsUpEnabled(true);
actionBar.setDisplayShowHomeEnabled(true);
actionBar.setIcon(R.drawable.ic_cast_dark);
I would have thought that setDisplayHomeAsUpEnabled' and 'setIcon
should have been enough but seems not.
I am using android.support.v7.app.ActionBar
You can achieve this result by creating a custom Drawable
containing an arrow and image icon then add it to the toolbar toolbar.setNavigationIcon(drawable)
as shown below.
setSupportActionBar(mBinding.toolbar);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
Drawable drawable = new Drawable() {
@Override
public void draw(Canvas canvas) {
int width = canvas.getWidth();
int height = canvas.getHeight();
Bitmap bMap = CircularImageView.getCircularBitmap(BitmapFactory.decodeResource(getResources(), R.drawable.beautiful_eyes_small));
canvas.drawBitmap(bMap,(width-(bMap.getWidth())), (height/2)-(bMap.getHeight()/2), null);
Bitmap bMap2 = BitmapFactory.decodeResource(getResources(), R.drawable.ic_arrow_back);
canvas.drawBitmap(bMap2,0, (height/2)-(bMap2.getHeight()/2), null);
}
@Override
public void setAlpha(int i) {
}
@Override
public void setColorFilter(ColorFilter colorFilter) {
}
@Override
public int getOpacity() {
return PixelFormat.TRANSLUCENT;
}
};
mBinding.toolbar.setNavigationIcon(drawable);
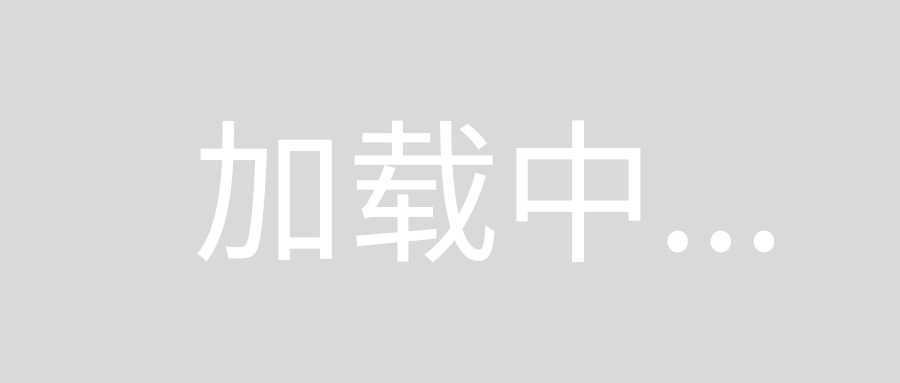
You can set the padding of the Home Icon using the below code
((ImageView) findViewById(android.R.id.home)).setPadding(x, x, x, x);
x = set your value.
Well I think you should try to do some edits in back-button image like apply some conciseness reduce image width and also making it transparent. May be helpful
you can't do this with the normal back arrow in toolbar because it has a
minWidth 56dp with scaleType center you can do something like this:
<android.support.v7.widget.Toolbar xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="?attr/colorPrimary">
<ImageButton
android:id="@+id/ibCustomBack"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:background="?attr/selectableItemBackground"
android:src="@drawable/ab_back_mtrl_am_alpha" />
<ImageView
android:id="@+id/yourImage"
android:layout_width="35dp"
android:layout_height="35dp"
android:src="@drawable/pb" />
</android.support.v7.widget.Toolbar>
and handle ibCustomBack by your self.