可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
Implement an algorithm to merge an arbitrary number of sorted lists into one sorted list. The aim is to create the smallest working programme, in whatever language you like.
For example:
input: ((1, 4, 7), (2, 5, 8), (3, 6, 9))
output: (1, 2, 3, 4, 5, 6, 7, 8, 9)
input: ((1, 10), (), (2, 5, 6, 7))
output: (1, 2, 5, 6, 7, 10)
Note: solutions which concatenate the input lists then use a language-provided sort function are not in-keeping with the spirit of golf, and will not be accepted:
sorted(sum(lists,[])) # cheating: out of bounds!
Apart from anything else, your algorithm should be (but doesn't have to be) a lot faster!
Clearly state the language, any foibles and the character count. Only include meaningful characters in the count, but feel free to add whitespace to the code for artistic / readability purposes.
To keep things tidy, suggest improvement in comments or by editing answers where appropriate, rather than creating a new answer for each "revision".
EDIT: if I was submitting this question again, I would expand on the "no language provided sort" rule to be "don't concatenate all the lists then sort the result". Existing entries which do concatenate-then-sort are actually very interesting and compact, so I won't retro-actively introduce a rule they break, but feel free to work to the more restrictive spec in new submissions.
Inspired by Combining two sorted lists in Python
回答1:
Common Lisp already has a merge
function for general sequences in the language standard, but it only works on two sequences. For multiple lists of numbers sorted ascendingly, it can be used in the following function (97 essential characters).
(defun m (&rest s)
(if (not (cdr s))
(car s)
(apply #'m
(cons (merge 'list (car s) (cadr s) #'<)
(cddr s)))))
edit: Revisiting after some time: this can be done in one line:
(defun multi-merge (&rest lists)
(reduce (lambda (a b) (merge 'list a b #'<)) lists))
This has 79 essential characters with meaningful names, reducing those to a single letter, it comes out at 61:
(defun m(&rest l)(reduce(lambda(a b)(merge 'list a b #'<))l))
回答2:
OCaml in 42 characters:
let f=List.fold_left(List.merge compare)[]
I think I should get extra credit for 42 exactly?
回答3:
Python: 113 characters
def m(c,l):
try:
c += [l[min((m[0], i) for i,m in enumerate(l) if m)[1]].pop(0)]
return m(c,l)
except:
return c
# called as:
>>> m([], [[1,4], [2,6], [3,5]])
[1, 2, 3, 4, 5, 6]
EDIT: seeing as talk of performance has come up in a few places, I'll mention that I think this is pretty efficient implementation, especially as the lists grow. I ran three algorithms on 10 lists of sorted random numbers:
- my solution (Merge)
sorted(sum(lists, []))
(Built-in)
- Deestan's solution which sorted in a different way (Re-implement)
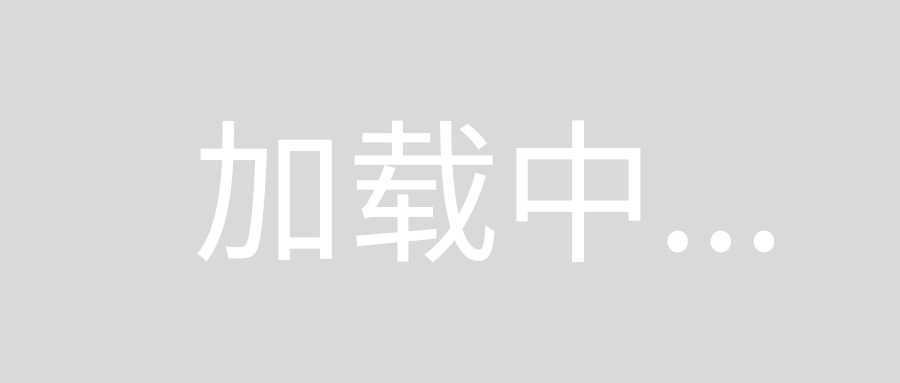
EDIT2: (JFS)
The figure's labels:
merge_26
-- heapq.merge()
from Python 2.6 stdlib
merge_alabaster
-- the above code (labeled Merge
on the above figure)
sort_builtin
-- L = sum(lists,[]); L.sort()
- Deestan's solution is O(N**2) so it is excluded from the comparison (all other solutions are O(N) (for the input provided))
Input data are [f(N) for _ in range(10)]
, where f()
is:
max_ = 2**31-1
def f(N):
L = random.sample(xrange(max_), n)
L.sort()
return L
f.__name__ = "sorted_random_%d" % max_
NOTE: merge_alabaster()
doesn't work for N > 100
due to RuntimeError: "maximum recursion depth exceeded"
.
To get Python scripts that generated this figure, type:
$ git clone git://gist.github.com/51074.git
Conclusion: For reasonably large lists the built-in sort shows near linear behaviour and it is the fastest.
回答4:
Ruby: 100 characters (1 significant whitespace, 4 significant newlines)
def m(i)
a=[]
i.each{|s|s.each{|n|a.insert((a.index(a.select{|j|j>n}.last)||-1)+1,n)}}
a.reverse
end
Human version:
def sorted_join(numbers)
sorted_numbers=[]
numbers.each do |sub_numbers|
sub_numbers.each do |number|
bigger_than_me = sorted_numbers.select { |i| i > number }
if bigger_than_me.last
pos = sorted_numbers.index(bigger_than_me.last) + 1
else
pos = 0
end
sorted_numbers.insert(pos, number)
end
end
sorted_numbers.reverse
end
This can all just be replaced by numbers.flatten.sort
Benchmarks:
a = [[1, 4, 7], [2, 4, 8], [3, 6, 9]]
n = 50000
Benchmark.bm do |b|
b.report { n.times { m(a) } }
b.report { n.times { a.flatten.sort } }
end
Produces:
user system total real
2.940000 0.380000 3.320000 ( 7.573263)
0.380000 0.000000 0.380000 ( 0.892291)
So my algorithm performs horribly, yey!
回答5:
resubmitted
Python - 74 chars (counting whitespace and newlines)
def m(i):
y=[];x=sum(i,[])
while x:n=min(x);y+=[n];x.remove(n)
return y
i
is input as list of lists
Usage:
>>> m([[1,5],[6,3]])
[1, 3, 5, 6]
回答6:
Haskell: 127 characters (without indentation and newlines)
m l|all null l=[]
|True=x:(m$a++(xs:b))
where
n=filter(not.null)l
(_,min)=minimum$zip(map head n)[0..]
(a,((x:xs):b))=splitAt min n
It basically generalizes the merging of two lists.
回答7:
I'll just leave this here...
Language: C, Char count: 265
L[99][99];N;n[99];m[99];i;I;b=0;main(char t){while(scanf("%d%c",L[i]+I,&t)+1){++
I;if(t==10){n[i++]=I;I=0;}}if(I)n[i++] = I;N=i;while(b+1){b=-1;for(i=0;i<N;++i){
I=m[i];if(I-n[i])if(b<0||L[i][I]<L[b][m[b]])b=i;}if(b<0)break;printf("%d ",L[b][
m[b]]);++m[b];}puts("");}
Takes input like such:
1 4 7
2 5 8
3 6 9
(EOF)
回答8:
Though I have not had the patience to try this, a colleague of mine showed me a way that it may be possible to do this using 0 character key - Whie Space
回答9:
(all other solutions are O(N) (for the input provided))
If we let N be the number of elements in the output and k the number of input lists, then you can't do faster than O(N log k) -- suppose that each list was only a single element, and you'd have faster-than-O(N log N) comparison-based sorting.
Those I've looked at look more like they're O(N*k).
You can fairly easily get down to O(N log k) time: just put the lists in a heap. This is one of the ways to do I/O-efficient sorting (you can generalize quicksort and heaps/heapsort as well).
[no code, just commentary]
回答10:
F#: 116 chars:
let p l=
let f a b=List.filter(a b) in
let rec s=function[]->[]|x::y->s(f(>)x y)@[x]@s(f(<=)x y) in
[for a in l->>a]|>s
Note: this code causes F# to throw a lot of warnings, but it works :)
Here's the annotated version with whitespace and meaningful identifiers (note: the code above doesn't use #light syntax, the code below does):
let golf l=
// filters my list with a specified filter operator
// uses built-in F# function
// ('a -> 'b -> bool) -> 'a -> ('b list -> 'b list)
let filter a b = List.filter(a b)
// quicksort algorithm
// ('a list -> 'a list)
let rec qsort =function
| []->[]
| x :: y -> qsort ( filter (>) x y) @ [x] @ qsort ( filter (<=) x y)
// flattens list
[for a in l ->> a ] |> qsort
回答11:
C#
static void f(params int[][] b)
{
var l = new List<int>();
foreach(var a in b)l.AddRange(a);
l.OrderBy(i=>i).ToList().ForEach(Console.WriteLine);
}
static void Main()
{
f(new int[] { 1, 4, 7 },
new int[] { 2, 5, 8 },
new int[] { 3, 6, 9 });
}
回答12:
Javascript
function merge(a) {
var r=[], p;
while(a.length>0) {
for (var i=0,j=0; i<a.length && p!=a[j][0]; i++)
if (a[i][0]<a[j][0])
j = i;
r.push(p = a[j].shift());
if (!a[j].length)
a.splice(j, 1);
}
return r;
}
Test:
var arr = [[1, 4, 7], [2, 5, 8], [3, 6, 9]];
alert(merge(arr));
回答13:
VB is usually not the language of choice for code golf, but here goes anyway.
The setup -
Dim m1 As List(Of Integer) = New List(Of Integer)
Dim m2 As List(Of Integer) = New List(Of Integer)
Dim m3 As List(Of Integer) = New List(Of Integer)
Dim m4 As List(Of Integer) = New List(Of Integer)
m1.Add(1)
m1.Add(2)
m1.Add(3)
m2.Add(4)
m2.Add(5)
m2.Add(6)
m3.Add(7)
m3.Add(8)
m3.Add(9)
Dim m5 As List(Of List(Of Integer)) = New List(Of List(Of Integer))
m5.Add(m1)
m5.Add(m2)
m5.Add(m3)
An attempt in VB.NET (without sort)
While m5.Count > 0
Dim idx As Integer = 0
Dim min As Integer = Integer.MaxValue
For k As Integer = 0 To m5.Count - 1
If m5(k)(0) < min Then min = m5(k)(0) : idx = k
Next
m4.Add(min) : m5(idx).RemoveAt(0)
If m5(idx).Count = 0 Then m5.RemoveAt(idx)
End While
Another VB.NET attempt (with an allowed sort)
Private Function Comp(ByVal l1 As List(Of Integer), ByVal l2 As List(Of Integer)) As Integer
Return l1(0).CompareTo(l2(0))
End Function
.
.
.
While m5.Count > 0
m5.Sort(AddressOf Comp)
m4.Add(m5(0)(0)) : m5(0).RemoveAt(0)
If m5(0).Count = 0 Then m5.RemoveAt(0)
End While
The entire program -
Dim rand As New Random
Dim m1 As List(Of Integer) = New List(Of Integer)
Dim m2 As List(Of Integer) = New List(Of Integer)
Dim m3 As List(Of Integer) = New List(Of Integer)
Dim m4 As List(Of Integer) = New List(Of Integer)
Dim m5 As List(Of List(Of Integer)) = New List(Of List(Of Integer))
m5.Add(m1)
m5.Add(m2)
m5.Add(m3)
For Each d As List(Of Integer) In m5
For i As Integer = 0 To 100000
d.Add(rand.Next())
Next
d.Sort()
Next
Dim sw As New Stopwatch
sw.Start()
While m5.Count > 0
Dim idx As Integer = 0
Dim min As Integer = Integer.MaxValue
For k As Integer = 0 To m5.Count - 1
If m5(k)(0) < min Then min = m5(k)(0) : idx = k
Next
m4.Add(min) : m5(idx).RemoveAt(0)
If m5(idx).Count = 0 Then m5.RemoveAt(idx)
End While
sw.Stop()
'Dim sw As New Stopwatch
'sw.Start()
'While m5.Count > 0
' m5.Sort(AddressOf Comp)
' m4.Add(m5(0)(0)) : m5(0).RemoveAt(0)
' If m5(0).Count = 0 Then m5.RemoveAt(0)
'End While
'sw.Stop()
Console.WriteLine(sw.Elapsed)
Console.ReadLine()
回答14:
Ruby:
41 significant chars, 3 significant whitespace chars in the body of the merge method.
arrs is an array of arrays
def merge_sort(arrs)
o = Array.new
arrs.each do |a|
o = o | a
end
o.sort!
end
To test in irb:
arrs = [ [ 90, 4, -2 ], [ 5, 6, -100 ], [ 5, 7, 2 ] ]
merge_sort(arrs)
Returns:
[-100, -2, 2, 4, 5, 6, 7, 90]
Edit: Used the language provided merge/sort because it is likely backed by C code and meets the 'faster' requirement. I'll think about the solution without later (it's the weekend here and I am on holiday).
回答15:
Perl: 22 characters, including two significant whitespace characters.
sub a{sort map{@$_}@_}
Only builtins here. See? ;)
Call like so:
my @sorted = a([1, 2, 3], [5, 6, 89], [13, -1, 3]);
print "@sorted" # prints -1, 1, 1, 2, 3, 3, 5, 6, 89
Honestly, denying language features (note: not libraries...) seems kind-of counter the point. Shortest code to implement in a language should include buildins/language features. Of course, if you import a module, you should count that code against your solution.
Edit: removed unnecessary {}'s around the $_.
回答16:
F#, 32 chars
let f x=List.sort(List.concat x)
And without using a built in function for the concat (57 chars):
let f x=List.sort(Seq.toList(seq{for l in x do yield!l}))
回答17:
I don't think you can get much better than @Sykora's response, here, for Python.
Changed to handle your inputs:
import heapq
def m(i):
return list(heapq.merge(*i))
print m(((1, 4, 7), (2, 5, 8), (3, 6, 9)))
For the actual function, 59 characters, or the 52 in reduced version:
import heapq
def m(i): return list(heapq.merge(*i))
This also has the benefit of using a tested and true implementation built into Python
Edit: Removed the semi-colons (thanks @Douglas).
回答18:
Even though it might break the rules. Here's a nice and short c++ entry:
13 Characters
l1.merge(l2); // Removes the elements from the argument list, inserts
// them into the target list, and orders the new, combined
// set of elements in ascending order or in some other
// specified order.
回答19:
GNU system scripting (I guess that's cheating, but it is nice to know too).
sort -m file1 file2 file3 ...
回答20:
Implementation in C via Linked lists.
http://datastructuresandalgorithmsolutions.blogspot.com/2010/02/chapter-2-basic-abstract-data-types_7147.html
回答21:
BASH in about 250 essential chars
BASH is not really good at list manipulation, anyway this is does the job.
# This merges two lists together
m(){
[[ -z $1 ]] && echo $2 && return;
[[ -z $2 ]] && echo $1 && return;
A=($1); B=($2);
if (( ${A[0]} > ${B[0]} ));then
echo -n ${B[0]}\ ;
unset B[0];
else
echo -n ${A[0]}\ ;
unset A[0];
fi;
m "${A[*]}" "${B[*]}";
}
# This merges multiple lists
M(){
A=$1;
shift;
for x in $@; do
A=`m "$A" "$x"`
done
echo $A
}
$ M '1 4 7' '2 5 8' '3 6 9'
1 2 3 4 5 6 7 8 9
回答22:
VB.NET (2008) 185 chars
Accepts List(Of List(Of Byte))
Function s(i)
s=New List(Of Byte)
Dim m,c
Dim N=Nothing
Do
m=N
For Each l In i:
If l.Count AndAlso(l(0)<m Or m=N)Then m=l(0):c=l
Next
If m<>N Then s.Add(m):c.Remove(m)
Loop Until m=N
End Function
回答23:
Python, 107 chars:
def f(l):
n=[]
for t in l:
for i in t: n+=[t]
s=[]
while n: s.+=[min(n)]; n.remove(min(n))
return s
回答24:
Haskell like (158, but more than 24 spaces could be removed.):
mm = foldl1 m where
m [] b = b
m a [] = a
m (a:as) (b:bs)
| a <= b = a : m as (b:bs)
| true = b : m (a:as) bs
回答25:
VB
The setup:
Sub Main()
f(New Int32() {1, 4, 7}, _
New Int32() {2, 5, 8}, _
New Int32() {3, 6, 9})
End Sub
The output:
Sub f(ByVal ParamArray b As Int32()())
Dim l = New List(Of Int32)
For Each a In b
l.AddRange(a)
Next
For Each a In l.OrderBy(Function(i) i)
Console.WriteLine(a)
Next
End Sub
回答26:
Python, 181 chars
from heapq import *
def m(l):
r=[]
h=[]
for x in l:
if x:
heappush(h, (x[0], x[1:]))
while h:
e,f=heappop(h)
r.append(e)
if f:
heappush(h, (f.pop(0),f))
return r
This runs in O(NlgM) time, where N is the total number of items and M is the number of lists.