I am setting the content inset of a UICollectionView:
[_collectionView setContentInset:UIEdgeInsetsMake(0.f, 0.f, 100.f, 0.f)];
Then I am scrolling programmatically all the way to the bottom of the UICollectionView with this method:
- (void)scrollToLastMessageAnimated:(BOOL)animated;
{
if (_messages.count == 0) { return; }
NSUInteger indexOfLastSection = _messagesBySections.count - 1;
NSInteger indexOfMessageInLastSection = [_messagesBySections[indexOfLastSection] count] - 1;
NSIndexPath *path = [NSIndexPath indexPathForItem:indexOfMessageInLastSection
inSection:indexOfLastSection];
[_collectionView scrollToItemAtIndexPath:path
atScrollPosition:UICollectionViewScrollPositionCenteredVertically
animated:animated];
}
It is scrolling down, but it is ignoring the contentInset, meaning that the last cells are below the specified content inset:
The left image, shows how it appear now after the view did appear. In the right image, I manually scrolled further down to the last message.
I am using AutoLayout, any ideas why this happens?
EDIT:
Here is a screenshot of the IB setup:
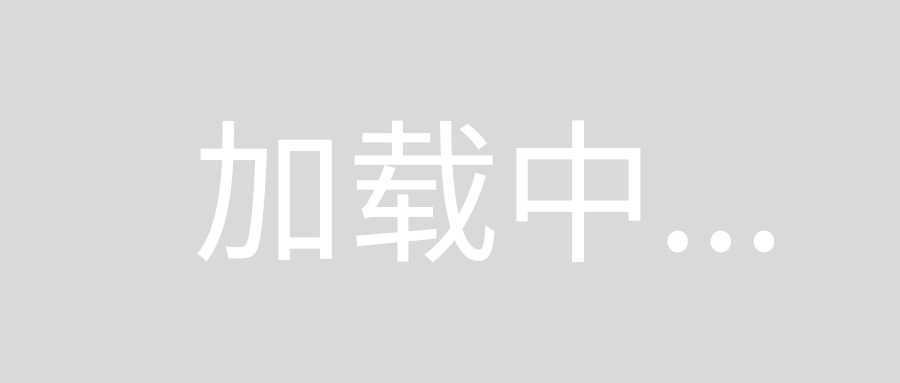
Today, by chance I discovered the solution!
Select your view controller and uncheck the option "Adjust Scroll View Insets".
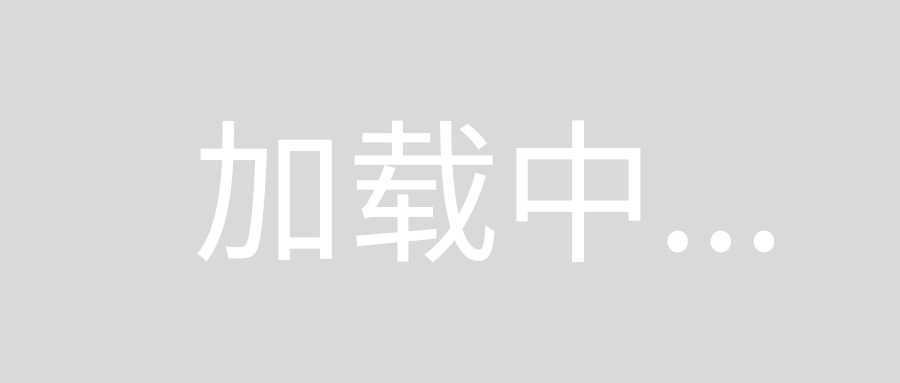
With this option unchecked, iOS does not automatically adjust your insets of the view (and probably its subviews), which caused the problems for me ... Uncheck it and configure your scroll insets like this programmatically:
- (void)configureInsetsOfCollectionView
{
[_collectionView setContentInset: UIEdgeInsetsMake(self.navigationController.navigationBar.bounds.size.height + [UIApplication sharedApplication].statusBarFrame.size.height + DEFAULT_SPACING, 0.f, _keyboardHeight + _toolbar.bounds.size.height + DEFAULT_SPACING, 0.f)];
[_collectionView setScrollIndicatorInsets:UIEdgeInsetsMake(self.navigationController.navigationBar.bounds.size.height + [UIApplication sharedApplication].statusBarFrame.size.height, 0.f, _keyboardHeight + _toolbar.bounds.size.height, 0.f)];
}
If you are using flow layout try to set _collectionView.collectionViewLayout.sectionInset.
Possible Issue
You have set the collectionView below the toolbar and added constraints to bottom of superview for both views.
Solution
Set the constraints of the toolbar to bottom, leading and set the width and height to fixed size. For your collectionView set the constraints to top, to bottom with the toolbar, leading to the superview and (alternative) with fixed size of width
Update
CollectionView
Follow these steps to make it work:
Check your collection view, and don't put it below toolbar, and add these constraints by selecting your collectionView on Document Outline, click ctrl and drag it to your view, a popup will appear, hold shift and select these constraints.
Toolbar
Check the leading and bottom, by dragging with ctrl in view. And add fixed width and height for toolbar.
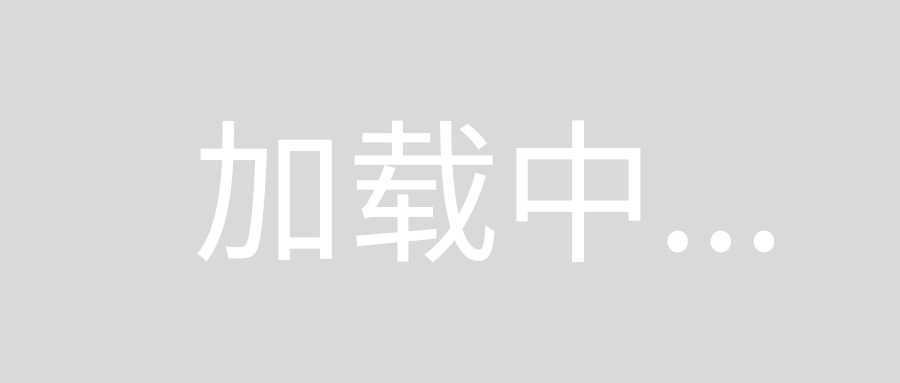
Dealing with scrolling before viewAppears
-(void)viewWillAppear:(BOOL)animated
{
[collectionView reloadData];
[self scrollToLastMessageAnimated:YES];
}
swift version
collectionview.contentInset = UIEdgeInsetsMake(44,0,0,0)
collectionview.scrollIndicatorInsets = UIEdgeInsetsMake(44,0,0,0)
You can set the referenceSizeForFooterInSection
function in your viewController class:
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, referenceSizeForFooterInSection section: Int) -> CGSize {
return CGSize(width: view.frame.width, height: 50)
}
Just set the height to your required value.