recently I started adding my application IOS 10 features while I encountered a weird bug:
When authenticating with facebook SDK via browser as soon as I click the confirmation button in facebook itself at the embeded browser, the app crashes.
Unfortunately this bug is not informative, the console doesn't tell me anything about it and there is not call stack to see where this exception was occurred.
Two points for demonstrating this bug cause:
1. This bug doesn't occur if the login is via System account but only when it in browser as you can see in the next photo:
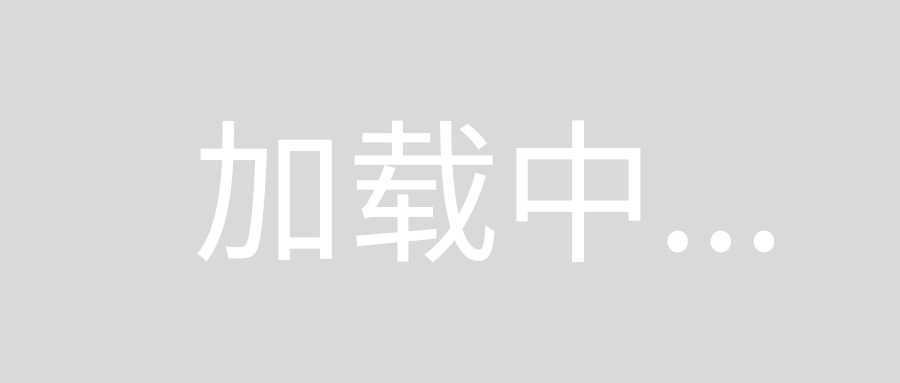
(As soon as I tap OK the exception occur)
- When I am running my app via Xcode 7.x the bug doesn't happen. So it's probably related to the integration of facebook SDK with the new compiler or something like that.
Hope someone has answer for that, or maybe an idea of how can I debug this kind of un informative bug.
Thanks in advance,
Liran.
I just ran into something similar but unfortunately i don't have the time to dig the problem. But i found this website, which explains pretty well how it works, and how you can debug it : What Is EXC_BAD_ACCESS and How to Debug It
So, here is why this error happens :
In summary, when you run into EXC_BAD_ACCESS, it means that you try to send a message to a block of memory that can't execute that message.
In some cases, however, EXC_BAD_ACCESS is caused by a corrupt pointer. Whenever your application attempts to dereference a corrupt pointer, an exception is thrown by the kernel.
One of the solutions given in this article is to use Zombie Objects :
Click the active scheme in the top left and choose Edit Scheme.
Select Run on the left and open the Diagnostics tab at the top. To enable zombie objects, tick the checkbox labeled Enable Zombie Objects.
If you now run into EXC_BAD_ACCESS, the output in Xcode's Console will give you a much better idea of where to start your search.
It should give you more information in the logs, so you could have an idea of what the root of the problem is. BTW, it didn't work for me.
Another solution is to check possible problematics areas in your code with the analyze Xcode tool.
Press Shift-Cmd-B
in order to use it, or Product -> Analyze
It should display the possible issues you have to check in the Issue Navigator on the left panel of Xcode. Click on the issue to display the block of code which is suspicious for Xcode, but which may finally not be a part of the problem at all.
For more information, check on the link above, I just summed up what the article says.
I was running into this issue as well. It seems one of the UIApplication Delegate methods was deprecated in iOS 9 and presumably removed in iOS 10.
optional func application(_ application: UIApplication, open url: URL, sourceApplication: String?, annotation: Any) -> Bool
I replaced it with the following method:
func application(_ app: UIApplication, open url: URL, options: [UIApplicationOpenURLOptionsKey : Any] = [:]) -> Bool {
return FBSDKApplicationDelegate.sharedInstance().application(app,
open: url,
sourceApplication: options[.sourceApplication] as! String,
annotation: options[.annotation])
}