I am using jquery-ui-resizable plugin in my project.
By default when you make a DOM Object jquery-ui-resizable the resizable handle will appear on right-bottom corner, I need the re-sizable handle on left-bottom corner.
--- EDIT ---
One more thing, it should not be re-sizable using right border but should be re-sizable using left border.
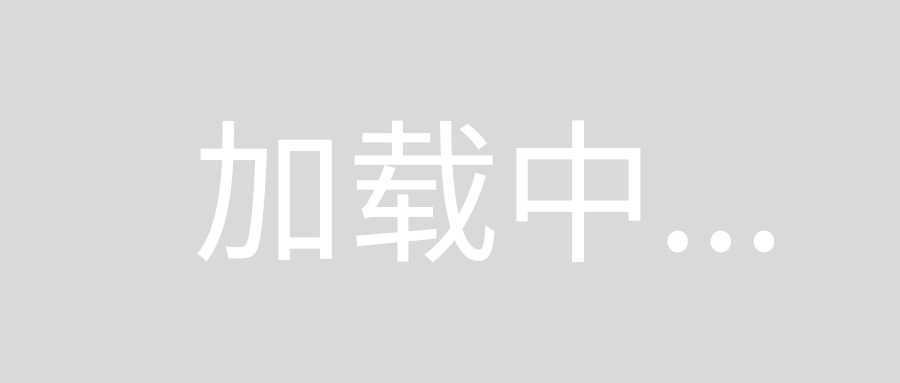
You should use the handles
supported: { n, e, s, w, ne, se, sw, nw }.
This will create the Handles on the side you specified. Default values are
'e, s, se'
Code examples
Initialize a resizable with the handles option specified.
$( ".selector" ).resizable({ handles: 'n, e, s, w' });
Get or set the handles option, after init.
//getter
var handles = $( ".selector" ).resizable( "option", "handles" );
//setter
$( ".selector" ).resizable( "option", "handles", 'n, e, s, w' );
Additional to Ahmed's answer:
jQueryUI doesn't include a icon for the sw-handle and also doesn't apply a icon-class for the sw-handle. You may use some CSS to add an icon:
#resizable .ui-resizable-sw{background:url(path/to/custom_icon.gif) no-repeat;}
To do re-sizing from all sides following code can also be used
var resizeOpts = {
handles: "all" ,autoHide:true
};
$( ".selector" ).resizable(resizeOpts);
and autoHide equal true means that grip icon will automatically hide when mouse pointer is taken away from dom object.
I was looking for this SW solution. What i had to do to make it work is add the ui-icon classes to the created div just after the call to resizable().
$( "#wproof" ).resizable({handles: "w, sw, s"});
$('.ui-resizable-sw').addClass('ui-icon ui-icon-gripsmall-diagonal-sw');
Then define the icon by taking it in images/ui-icons_222222_256x240.png
It is a small 9X9 area at the bottom. Make a .png file of it ( with a rotation ! ) and add the style to the given class :
<style>
.ui-icon-gripsmall-diagonal-sw {
background-image: url(your 9x9 icon.png); }
.ui-resizable-sw {
bottom: 1px;
left: 1px;
}
</style>
The bottom and left are set at -5px in jquery-ui css
Seems like the standard cursor property is what is used by the UI CSS
.ui-resizable-sw {
cursor: sw-resize;
height: 7px;
width: 100%;
top: -5px;
left: 0;
}
Also it seems that cursor does not display and resizable is not enabled for the side of the element that is used for positioning - for example: a div positioned using left:20px top:20px will not enable resizing on those left and top sides
I discovered this after adding all handles but then they all never showed up !
Update: Apparently there is some problem within my app that is causing my problem. Testing on JSFiddle all handles do work as expected using the same version of JQuery.....argggggg