with this code I get just a border:
<shape
xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="ring"
android:innerRadius="15dp"
android:thickness="2dp"
android:useLevel="false">
<solid android:color="#4d4d4d" />
</shape>
how can I make a ring shape like below image :
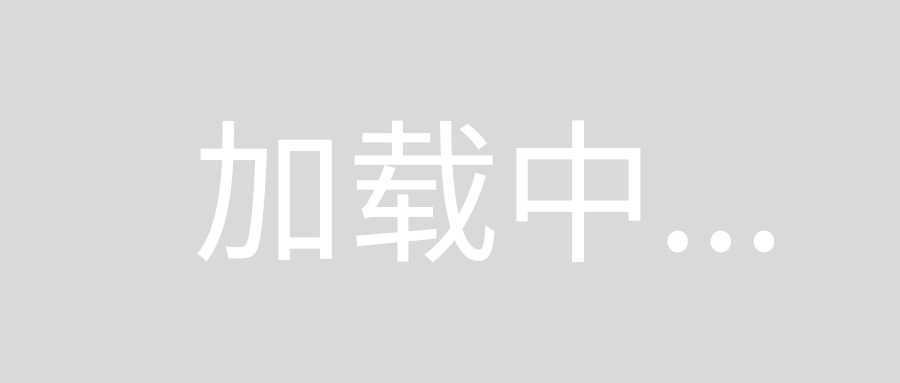
2dp outer ring with a 2dp gap:
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:top="4dp"
android:right="4dp"
android:bottom="4dp"
android:left="4dp">
<shape
android:shape="oval">
<solid android:color="#4d4d4d" />
</shape>
</item>
<item>
<shape
android:shape="oval">
<stroke android:width="2dp"
android:color="#4d4d4d"/>
</shape>
</item>
</layer-list>
Ring drawable is juxtaposition of elements, use layer-list
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item android:right="6dip" android:left="6dip">
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:innerRadius="0dp"
android:shape="ring"
android:thicknessRatio="3"
android:useLevel="false" >
<solid android:color="@android:color/transparent" />
<stroke
android:width="5dp"
android:color="@color/maroon" />
</shape>
</item>
<item android:right="20dip"
android:left="20dip"
android:bottom="0dip"
android:top="34dip">
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle"
android:innerRadius="0dp">
<solid android:color="@color/maroon" />
<stroke android:width="1dip" android:color="@android:color/transparent" />
</shape>
</item>
<item android:right="20dip"
android:left="20dip"
android:bottom="34dip"
android:top="0dip">
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle"
android:innerRadius="0dp">
<solid android:color="@color/maroon" />
<stroke android:width="1dip" android:color="@android:color/transparent" />
</shape>
</item>
</layer-list>
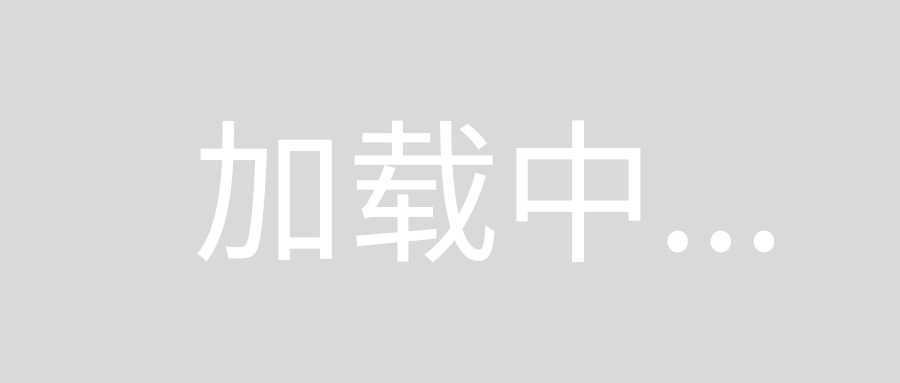
Xml drawable by all means more useful than static images, they can be correctly scaled without need of .9 library or generating set of different sizes from Gimp, Photoshop
I think using a shape in android is better than a shape in photoshop.
Correct, creating a drawable it's better because you can change the colour or shape with code instead of creating a new image resource, for example.
Create a FrameLayout
with 2 Views
and a TextView
. The first view background would be your outer ring (shape) and the second a filed circle (shape). Finally the last View
(bigger z-index) your TextView
:
<FrameLayout>
<View/><!-- (outer ring)-->
<View/><!-- (filed circle)-->
<TextView/><!-- (text)-->
</FrameLayout>
<item>
<shape
android:innerRadiusRatio="4"
android:shape="ring"
android:thicknessRatio="15"
android:useLevel="false" >
<solid android:color="@color/white_color" />
<size
android:height="48dip"
android:width="48dip" />
</shape>
</item>
<item>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="ring"
android:innerRadius="0dp"
android:thickness="55dp"
android:useLevel="false">
<solid android:color="@color/white_color"/>
<size android:height="200dp"
android:width="200dp"/>
<stroke android:color="@color/green_color" android:width="5dp"/>
</shape>
</item>
<?xml version="1.0" encoding="utf-8"
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item>
<shape android:innerRadius="10dp" android:shape="ring" android:thickness="2dp" android:useLevel="false">
<solid android:color="#dfdfdf" />
</shape>
</item>
</selector>