None of the other questions I have read on stackoverflow have been able to help with my problem. As far as I can tell, I am doing everything correctly.
I have a master/detail flow with fragments.
Upon creation of the main activity, the master fragment is loaded with the following code:
Fragment frag;
frag = new MainListFragment();//<-- **the master fragment**
FragmentManager fm = getFragmentManager();
FragmentTransaction transaction = fm.beginTransaction();
transaction.replace(R.id.fragment_container, frag);
Log.d("My Debug Bitches", "stack:" + fm.getBackStackEntryCount());
transaction.commit();
The master fragment has a ListView
; clicking on a list item brings up the details fragment like so:
@Override
public void onListItemClick(ListView listView, View view, int position, long id) {
super.onListItemClick(listView, view, position, id);
FragmentManager fm = getFragmentManager();
FragmentTransaction transaction = fm.beginTransaction();
SubListFragment frag = new SubListFragment();//<-- **the detail fragment**
transaction.replace(R.id.fragment_container, frag);
transaction.addToBackStack(null);
transaction.commit();
fm.executePendingTransactions();
Log.d("My Debug Bitches", "stack:" + fm.getBackStackEntryCount());
}
Now, according to LogCat, the BackStackEntryCount
changes from 0 to 1 after I navigate from master fragment to detail fragment: 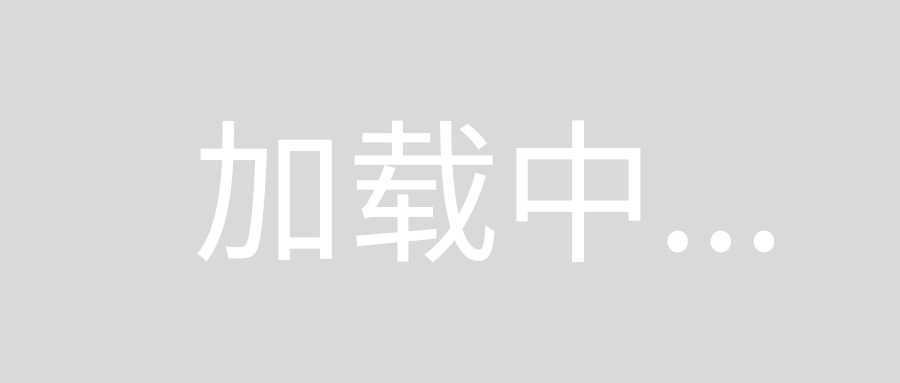
So why is it that, when I click the back button while in the details fragment, that the app closes instead of returning to the master fragment??????????
You have to add the popBackStack()
call to the onBackPressed()
method of the activity.
Ex:
@Override
public void onBackPressed() {
if (fragmentManager.getBackStackEntryCount() > 0) {
fragmentManager.popBackStack();
} else {
super.onBackPressed();
}
}
@Bobbake4's answer is awesome, but there is one little problem.
Let's say I have three fragments A, B and C.
A is the main Fragment (the fragment that shows when I launch my app), B and C are fragments I can navigate to from the navigation drawer or from A.
Now, when I use the back button from B or C, I go back to the previous fragment (A) alright, but the title of the previous fragment (fragment B or C) now shows in the actionBar title of Fragment A. I have to press the back button again to "truly" complete the back navigation (to display the view and correct title for the fragment and returning to)
This is how I solved this problem. Declare these variables.
public static boolean IS_FRAG_A_SHOWN = false;
public static boolean IS_FRAG_B_SHOWN = false;
public static boolean IS_FRAG_C_SHOWN = false;
In the MainActivity of my app where am handling navigation drawer methods, I have a method displayView(position)
which handles switching of my fragments.
private void displayView(int position) {
IS_FRAG_A_SHOWN = false;
IS_FRAG_B_SHOWN = false;
IS_FRAG_C_SHOWN = false;
// update the main content by replacing fragments
Fragment fragment = null;
switch (position) {
case 0:
fragment = new FragmentA();
IS_FRAG_A_SHOWN = true;
break;
case 1:
fragment = new FragmentB();
IS_FRAG_B_SHOWN = true;
break;
case 2:
fragment = new FragmentC();
IS_FRAG_C_SHOWN = true;
break;
default:
break;
}
finally, in my onBackPressed
method, I do this:
public void onBackPressed() {
if(fragmentManager.getBackStackEntryCount() != 0) {
fragmentManager.popBackStack();
if (IS_FRAG_A_SHOWN) { //If we are in fragment A when we press the back button, finish is called to exit
finish();
} else {
displayView(0); //else, switch to fragment A
}
} else {
super.onBackPressed();
}
}