可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I would like to display an image (width: 320 pixels, height: 1250 pixels) in an image view.
When I compile the application I get no scrolling at all. The view stays fixed.
What I did:
- Added an
UIScrollView
via Interface Builder to my view.
- Added an
UIImageView
via Interface Builder to my view.
- Verified that
UIImageView
is below UIScrollView
in Interface Builder.
- Set the size of the
UIScrollView
with ViewDidLoad
.
How do I do this?
Code:
- (void)viewDidLoad
{
[super viewDidLoad];
scrollView.contentSize = CGSizeMake(320, 1250);
}
Screenshots:
ImageView
:
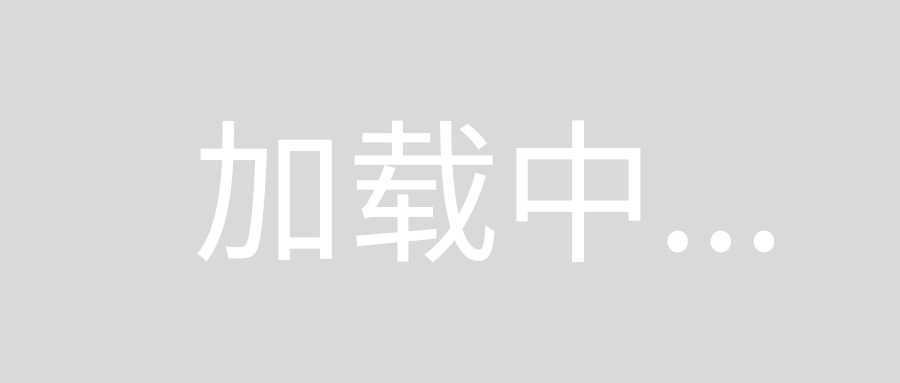
ScrollView
:
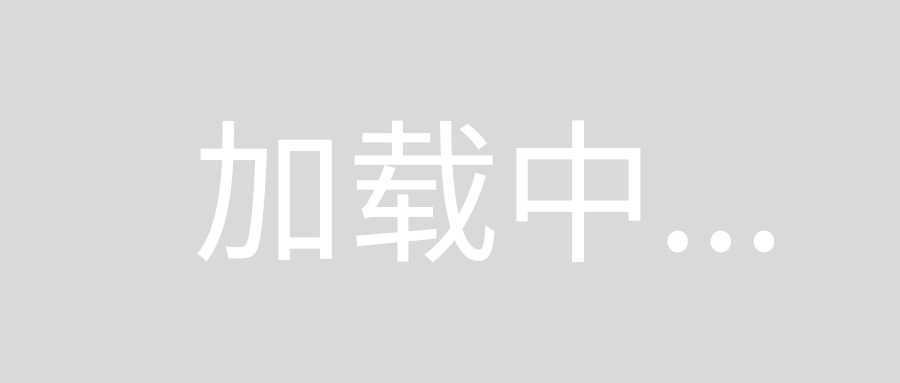
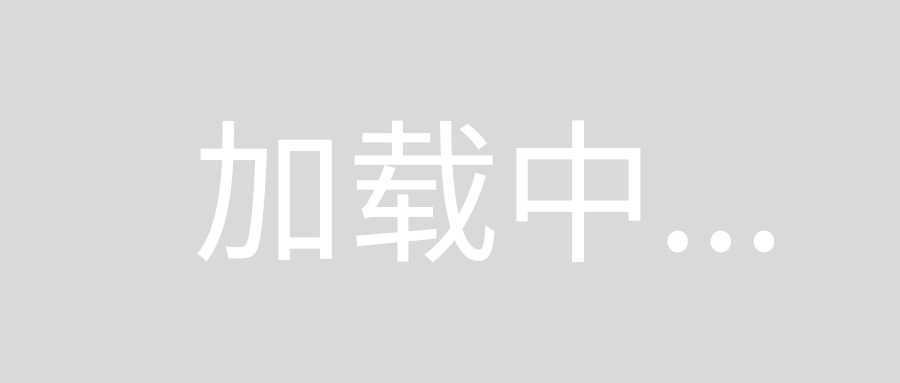
回答1:
I just have done the same task..
Try this one.....
scrollView.delegate = self;
scrollView.scrollEnabled = YES;
int scrollWidth = 120;
scrollView.contentSize = CGSizeMake(scrollWidth,80);
int xOffset = 0;
imageView.image = [UIImage imageNamed:[imagesName objectAtIndex:0]];
for(int index=0; index < [imagesName count]; index++)
{
UIImageView *img = [[UIImageView alloc] init];
img.bounds = CGRectMake(10, 10, 50, 50);
img.frame = CGRectMake(5+xOffset, 0, 50, 50);
NSLog(@"image: %@",[imagesName objectAtIndex:index]);
img.image = [UIImage imageNamed:[imagesName objectAtIndex:index]];
[images insertObject:img atIndex:index];
scrollView.contentSize = CGSizeMake(scrollWidth+xOffset,110);
[scrollView addSubview:[images objectAtIndex:index]];
xOffset += 70;
}
Also set this one....
imagesName = [[NSArray alloc]initWithObjects:@"image1.jpg",@"image2.jpg",@"image3.jpg",@"image4.jpg",@"image5.jpg",@"image6.png",@"image7.png",@"image9.png",nil];
images = [[NSMutableArray alloc]init];
回答2:
So for me the problem was that setting the content size didn't work in viewDidLoad()
. I tried everything and I didn't understand why it wouldn't want to work, and then I tried the same stuff in viewDidAppear()
and it magically worked...
回答3:
From you last screenshot and from your comments it looks like your scrollView is way to big.
The scrollview must be visible on screen completely. For example a full screen UIScrollView on iPhone would have a size of 320 x 460.
If the scrollview is the same size as its content you can't scroll.
The greenish rectangle shows the size of your scrollview, the pinkish the size of your content (your image):
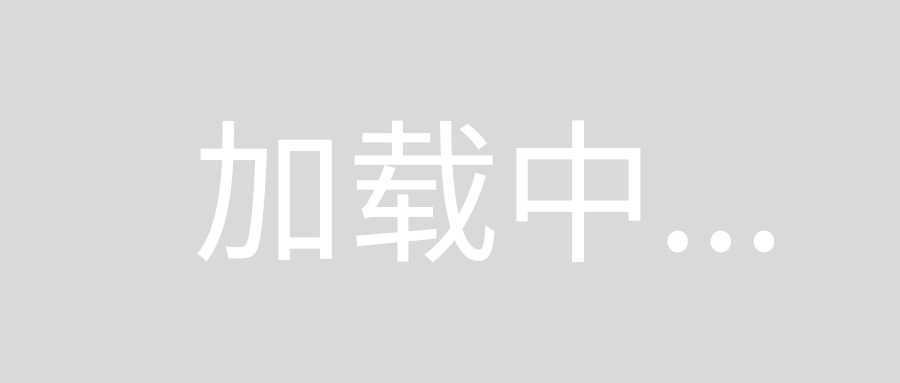
回答4:
I came across this same issue on iOS6 and the solution was to programmatically adjust the ContentSize.
So I will just quote from Raja (above) to show this:
CGSize scrollViewContentSize = CGSizeMake(320, 400);
[self.scrollView setContentSize:scrollViewContentSize];
NOTE: I was not having this issue on iOS5.. seems like iOS6 decided to do alot of prank just like the rotation/orientation saga
回答5:
Since Xcode 5 it does not work like before. Also scrolling to the end of a scrollable text field makes problems. There are also differences between doing it on iPhone or iPad. On iPhone it worked without delayed timer.
This worked for me:
- (void)viewDidLoad
{
[super viewDidLoad];
NSTimer *timerforScrollView;
timerforScrollView =[NSTimer scheduledTimerWithTimeInterval:0.1
target:self selector:@selector(forScrollView)userInfo:nil repeats:NO];
}
- (void) forScrollView {
[scrollviewPad setScrollEnabled:YES];
[scrollviewPad setContentSize:CGSizeMake(768, 1015)]; // must be greater then the size in Storyboard
}
回答6:
I found I had a similar problem but none of the above code worked. The issue was due to autolayout. I found that if I turned off autolayout by going to the storyboard clicking on Utilities -> File Inspector and unchecked Use Autolayout
the scrolling did work (I also set scroll.contentSize = ...
).
回答7:
Swift 4, iOS 11
The simplest way using autolayout:
- Add UIScrollView and pin it 0,0,0,0 to superview (or your desired size)
- Add UIView in ScrollView, pin it 0,0,0,0 to all 4 sides and center it horizontally and vertically.
- In size inspector, change 'bottom' and 'align center Y' priority to 250.
- Add all views that you need into this view. Don't forget to set the bottom constraint on the lowest view.
回答8:
Sometimes autoLayout checkbox is ticked in the xib. That also creates this issue in xcode 5. Which makes the UIScrollView scrolling off.
回答9:
Don't forget to add the category protocol to the interface, like this
@interface MyViewController : <UIScrollViewDelegate>
If you don't, you will not be able to set the scroll view delegate to self
(i.e. [MyScrollView setDelegate:self];
)
If you do this, it should work.
My code is:
-(void)viewDidAppear:(BOOL)animated {
[super viewDidAppear:animated];
[contentScrollView setDelegate:self];
[contentScrollView setScrollEnabled:YES];
contentScrollView.contentSize = CGSizeMake(310, 500);
contentScrollView.frame = CGRectMake(5, 188, 310, 193);
}
回答10:
Did you assign the scroll's view delegate? Always remember these:
[self.scrollView setDelegate:self];
[self.scrollView setScrollEnabled:YES];
回答11:
The image view has to be a subview (so inside AND below) of the scrollview. From your description it seems they are paralell
回答12:
You forgot one line. Add this to your view load function:
[scrollView setScrollEnabled:YES];
回答13:
You could try disabling AutoLayout. In XCode 5 I tested all the above answers and I could only scroll it by disabling autolayout and activating all autosizing masks under the Size Inspector. The following code was used too:
self.scrollView.contentSize = CGSizeMake(320, 900);
self.scrollView.delegate = self;
self.scrollView.scrollEnabled = YES;
self.scrollView.frame = self.view.frame;
回答14:
CGRect scrollViewFrame = CGRectMake(0, 0, 320, 400);
self.scrollView = [[UIScrollView alloc] initWithFrame:scrollViewFrame];
[self.view addSubview:self.scrollView];
CGSize scrollViewContentSize = CGSizeMake(320, 400);
[self.scrollView setContentSize:scrollViewContentSize];
scrollView.delegate = self;
[self.scrollView setBackgroundColor:[UIColor blackColor]];
[scrollView setCanCancelContentTouches:NO];
scrollView.indicatorStyle = UIScrollViewIndicatorStyleWhite;
scrollView.clipsToBounds = YES;
scrollView.scrollEnabled = YES;
scrollView.pagingEnabled = YES;
NSUInteger nimages = 0;
CGFloat cx = 0;
for (; ; nimages++) {
NSString *imageName = [NSString stringWithFormat:@"image%d.jpg", (nimages + 1)];
UIImage *image = [UIImage imageNamed:imageName];
if (image == nil) {
break;
}
UIImageView *imageView = [[UIImageView alloc] initWithImage:image];
CGRect rect = imageView.frame;
rect.size.height = image.size.height;
rect.size.width = image.size.width;
rect.origin.x = ((scrollView.frame.size.width - image.size.width) / 2) + cx;
rect.origin.y = ((scrollView.frame.size.height - image.size.height) / 2);
imageView.frame = rect;
[scrollView addSubview:imageView];
[imageView release];
cx += scrollView.frame.size.width;
}
[scrollView setContentSize:CGSizeMake(cx, [scrollView bounds].size.height)];
回答15:
Assuming that scrollView is a subview of view and fills it entirely you can use the following in viewDidLoad:
[scrollView setContentSize: CGSizeMake(self.view.frame.size.width, self.view.frame.size.height)];
I had a UIScrollView that was not scrolling and this allowed it to scroll.
回答16:
Just add code
scrollView.contentSize = CGSizeMake(WIDTH,HEIGHT);
to method -(void)viewDidLayoutSubviews.
For more information checkout Stanford CS193p Lecture No 8 to understand View Controller Life cycle.
回答17:
I had the same issue and was looking for the answer in this thread. I tried all the stuff, but nothing works. Then I found this:
.
You just need to deselect "Use Auto Layout" in File Inspector of your ViewController
. Ta-Da, it works immediately for me. Enjoy.