I was hoping $('#childDiv2 .txtClass')
or $('#childDiv2 input.txtClass')
perform better when selecting <input type="text" id="txtID" class="txtClass"/>
element. But according to this performance analysis $('.txtClass');
is the best selector among this. I'm using JQuery 1.7.2
Does anybody have explanation for this?
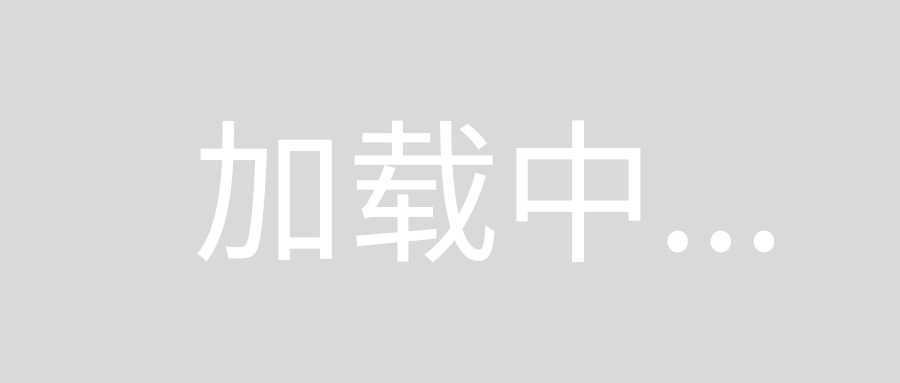
HTML
<div class="childDiv2">
<input type="text" id="txtID" class="txtClass"/>
<p class="child">Blah Blah Blah</p>
</div>
JS
$('.txtClass');
$('#childDiv2 .txtClass')
$('#childDiv2 > .txtClass')
$('input.txtClass')
$('#childDiv2 input.txtClass')
Modern browsers expose a very efficient getElementsByClassName() method that returns the elements having a given class. That's why a single class selector is faster in your case.
To elaborate on your examples:
$(".txtClass") => getElementsByClassName()
$("#childDiv2 .txtClass") => getElementById(),
then getElementsByClassName()
$("#childDiv2 > .txtClass") => getElementById(),
then iterate over children and check class
$("input.txtClass") => getElementsByTagName(),
then iterate over results and check class
$("#childDiv2 input.txtClass") => getElementById(),
then getElementsByTagName(),
then iterate over results and check class
As you can see, it's quite logical for the first form to be the fastest on modern browsers.
var obj = document.getElementById("childDiv");
xxx = obj.getElementsByClassName("txtClass");
is 30x faster.
http://jsperf.com/selectors-perf/6
CSS Selectors are parsed from right to left. So your example
$('#childDiv2 .txtClass')
will take two actions to complete. First find all elements with class txtClass
. Then check each element for being a child of the element with the id childDiv2
.
$('.txtClass')
This selector will just take one action. Find all elements with class txtClass
Have a look at this article on css-tricks.com
Looks like it also depends on the density of the elements with the class among the elements of the type.
I ran the tests with Google Chrome Version 30.0.1599.69 using JQuery 1.10.1. Feel free to try it on another browser and/or using another JQuery version.
I tried to run the following tests:
Sparse (10% of the div's have the class) link to the test on
jsbin
Dense (90% of the div's have the class) link to the test on
jsbin
Looks like in the Dense case div.class
wins, but in the Sparse case .class
wins.