I changed the scope variable in an if statement and outside the if statement it turned into an undefined variable
app.controller("Login", function($scope, $window,$http){
var status;
$scope.loginUser = function(logData){
$http.post('/corporate/login',logData).then(function(response){
var data = response.data
var status = data.success;
if(status == true){
$scope.logStatus = true;
console.log($scope.logStatus); // prints true
}else{
$scope.logStatus = false;
}
})
console.log($scope.logStatus); //prints undefined
}
});
outside ... it turned into an undefined variable
It did not "turn into" an undefined
value. The last console.log
in the code executes before the console.log in the success handler. It is undefined
because it has not yet been set by the success handler.
Expaination of Promise-Based Asynchronous Operations
console.log("Part1");
console.log("Part2");
var promise = $http.get(url);
promise.then(function successHandler(response){
console.log("Part3");
});
console.log("Part4");
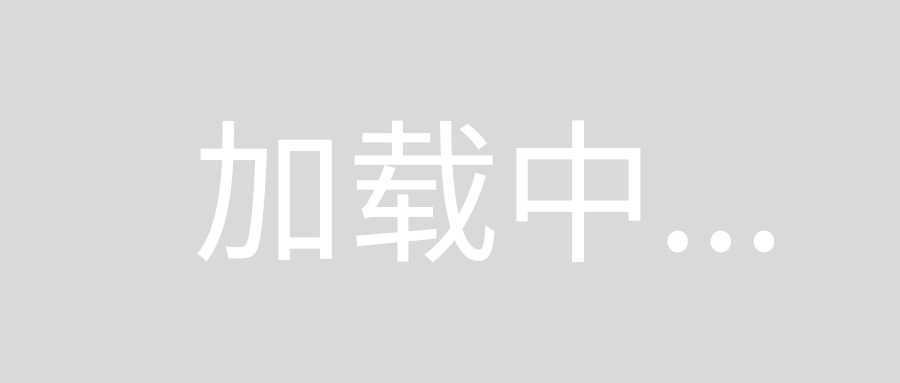
The console log for "Part4" doesn't have to wait for the data to come back from the server. It executes immediately after the XHR starts. The console log for "Part3" is inside a success handler function that is held by the $q service and invoked after data has arrived from the server and the XHR completes.
For more information, see How to use $http promise response outside success handler.
Demo
console.log("Part 1");
console.log("Part 2");
var promise = new Promise(r=>r());
promise.then(function() {
console.log("Part 3");
});
console.log("Part *4*");
$http
is asynchronous. Your if/else
conditions are in the success callback. Problem is that the console.log($scope.logStatus);
line is called before the success callback gets called
You should probably initialise $scope.logStatus = false
before the $http
call.
EDIT:
If like you said, $scope.logStatus
remains false, then check if your if block is being called. See fiddle:
https://jsfiddle.net/51bsbzL0/253/