I've been working with CORS and encountered the following issue.
Client complains about no 'Access-Control-Allow-Origin' header is present, while they are present, and client make the actual POST request and receives 200.
function initializeXMLHttpRequest(url) { //the code that initialize the xhr
var xhr = new XMLHttpRequest();
xhr.open('POST', url, true);
xhr.withCredentials = true;
xhr.setRequestHeader('Content-Type', 'application/json; charset=UTF-8');
//set headers
for (var key in headers) {
if (headers.hasOwnProperty(key)) { //filter out inherited properties
xhr.setRequestHeader(key,headers[key]);
}
}
return xhr;
}
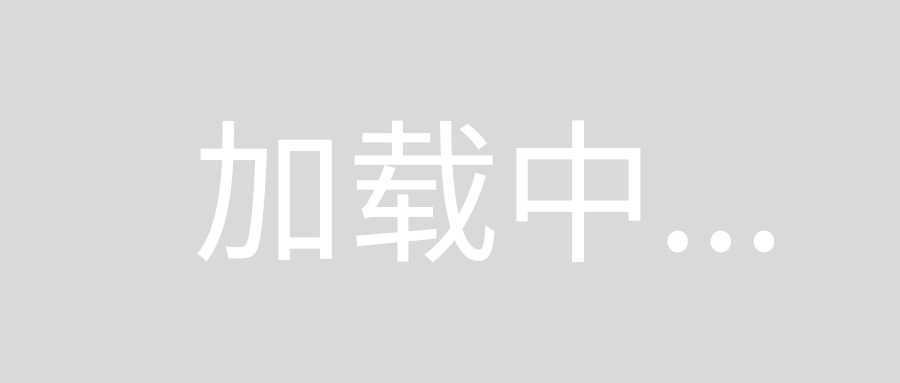
In Chrome
chrome console log
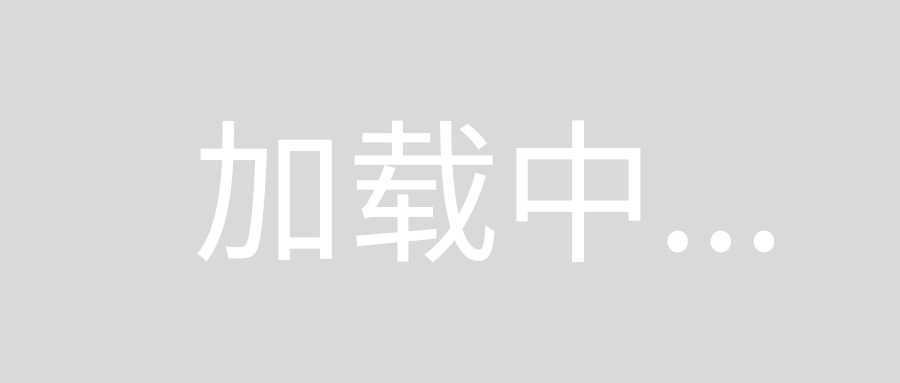
Chrome OPTIONS request
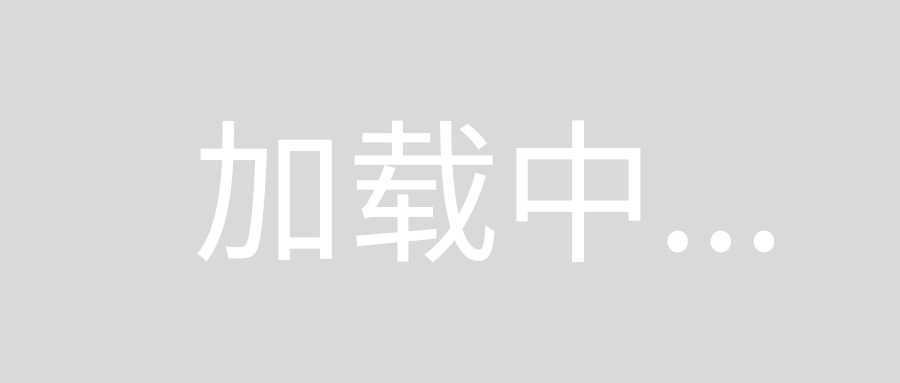
Chrome POST request
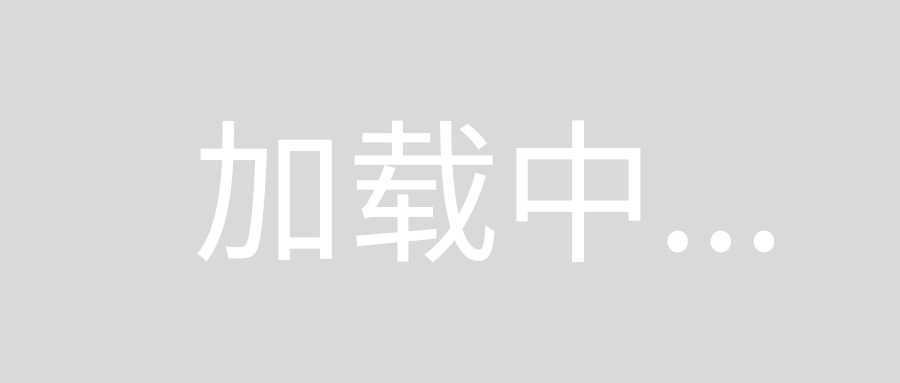
In Firefox
Firefox Console Log
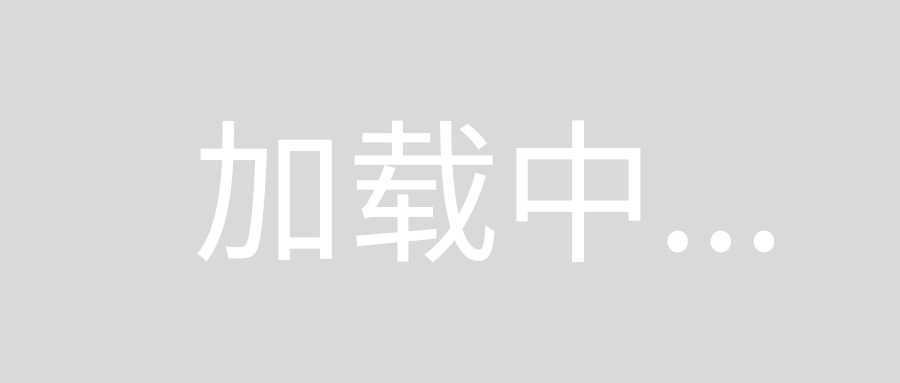
Firefox OPTIONS request
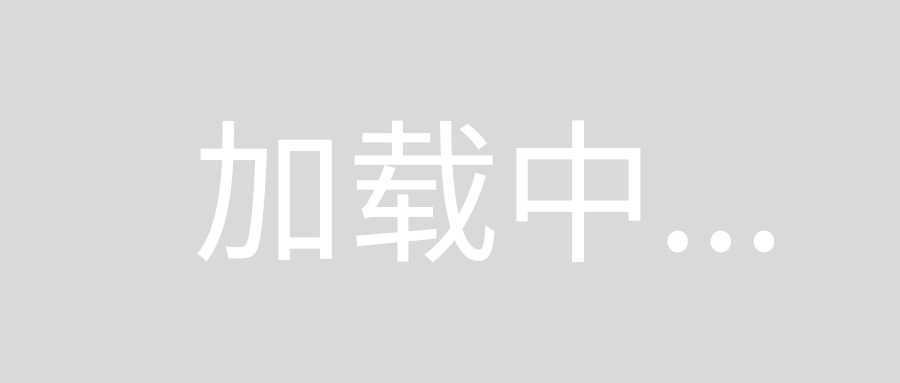
Firefox POST request
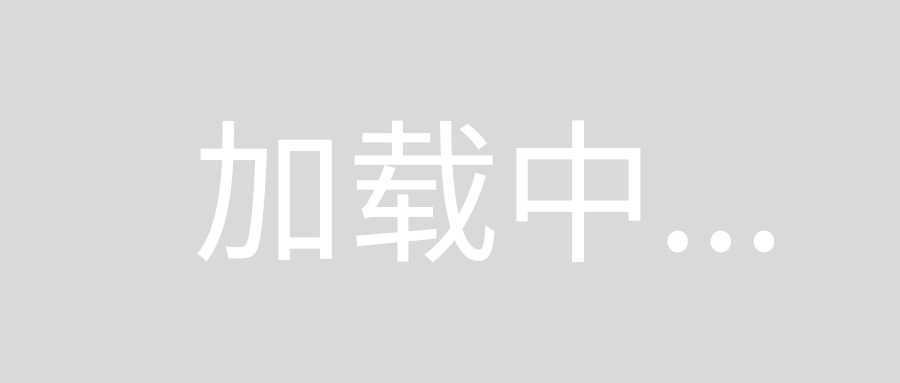
In short: Access control headers (e.g. Access-Control-Allow-Origin
) need to present in response for both OPTIONS and actual POST.
Work Flow:
Client make OPTIONS request with those HTTP access headers. (e.g. Origin, Access-Control-Request-Method, Access-Control-Request-Headers
)
Server respond with those access control headers, allowing access. (e.g. Access-Control-Allow-Origin, Access-Control-Expose-Headers, Access-Control-Max-Age, Access-Control-Allow-Credentials, Access-Control-Allow-Methods, Access-Control-Allow-Headers
)
Client makes POST request with data.
Server respond to POST. If Access-Control-Allow-Origin
header is NOT present in the server response. Although the POST is successful and shows 200 status code in network tab, xhr.status
is 0 and xhr.onerror
will be triggered. And browser would show up the error message.
Header References:
https://developer.mozilla.org/en-US/docs/Web/HTTP/Access_control_CORS
Value null
for Access-Control-Allow-Origin
won't do, it has to be either the origin domain or *
to allow any origin.
For more details, refer to MDN.