CodePen: http://codepen.io/leongaban/pen/hbHsk
I've found multiple answers to this question on stack here and here
However they all suggest the same fix, using type="number"
or type="tel"
None of these are working in my codepen or project :(
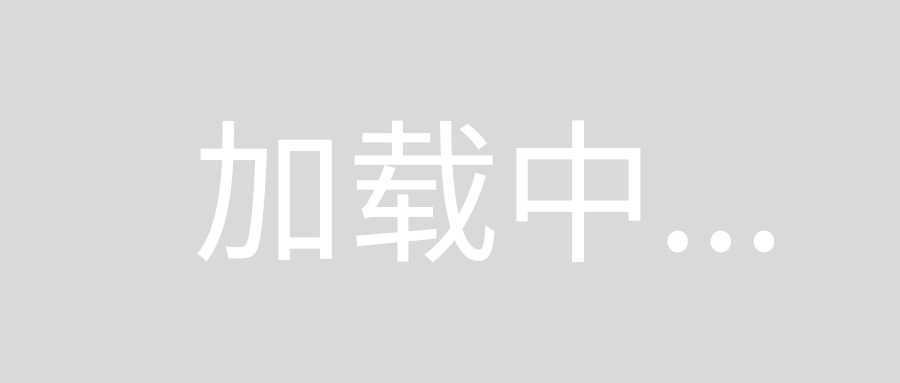
Do you see what I'm missing?
Firstly, what browsers are you using? Not all browsers support the HTML5 input types, so if you need to support users who might use old browsers then you can't rely on the HTML5 input types working for all users.
Secondly the HTML5 input validation types aren't intended to do anything to stop you entering invalid values; they merely do validation on the input once it's entered. You as the developer are supposed to handle this by using CSS or JS to determine whether the field input is invalid, and flag it to the user as appropriate.
If you actually want to prevent non-digit characters from ever getting into the field, then the answer is yes, you need to use Javascript (best option is to trap it in a keyUp
event).
You should also be careful to ensure that any validation you do on the client is also replicated on the server, as any client-side validation (whether via the HTML5 input fields or via your own custom javascript) can be bypassed by a malicious user.
It doesn't stop you from typing, but it does invalidate the input. You can see that if you add the following style:
input:invalid {
border:1px solid red;
}
Firstly, in your Codepen, your inputs are not fully formatted correctly in a form.... Try adding the <form></form>
tags like this:
<form>
<lable>input 1 </lable>
<input type='tel' pattern='[0-9]{10}' class='added_mobilephone' name='mobilephone' value='' autocomplete='off' maxlength='20' />
<br/>
<lable>input 2 </lable>
<input type="number" pattern='[0-9]{10}'/>
<br/>
<lable>input 3 </lable>
<input type= "text" name="name" pattern="[0-9]" title="Title"/>
</form>
I use a dirty bit of JS inline, it's triggered upon paste/keying (input).
Within your input tag add the following:
oninput="this.value=this.value.replace(/(?![0-9])./gmi,'')"
All it's doing is replacing any character not 0-9 with nothing.
I've written a tiny demo which you can try below:
Numbers only: <input oninput="this.value=this.value.replace(/(?![0-9])./gmi,'')"></input>
Just add a check to the onkeypress
event to make sure that the no alphanumeric characters can be added
<input
type="text"
placeholder="Age"
autocomplete="off"
onkeypress="return event.charCode >= 48 && event.charCode <= 57"
maxlength="10"
/>