We are using jasperReports and iReports in our web application to generate reports.
When I explored jasper reports I was able to insert watermark inside the report easily..
However my purpose is to put the watermark String say (Michael Jackson) across the page.
Going through the properties I can only find rotation option of left/right and upside down
...
Is it possible to place watermark in the report across the page..
I am using ireport
to design the report...
Rotating text to any angle other than 90, 180 or 270 degrees is not natively supported by JasperReports. A solution would be to generate an image of the rotated text and render that on the report instead.
In the last post of this jasperforge thread, user "artduc" shares a report scriptlet for doing exactly that.
I'm just spelling out what GenericJon suggested...
Add an image element to the background band (settings see screenshot).
Implement Renderable and pass it to Jasper Reports via parameters map:
InputStream jasperReportInputStream = getClass().getResourceAsStream("/reports/Test.jasper");
JRBeanCollectionDataSource dataSource = ...
Map parameters = new HashMap();
parameters.put("watermark", new WaterMarkRenderer(true);
JasperPrint jasperPrint = JasperFillManager.fillReport(jasperReportInputStream, parameters, dataSource);
The WatermarkRenderer:
@SuppressWarnings("deprecation")
public class WaterMarkRenderer extends JRAbstractRenderer
{
private boolean m_licenseTrial = false;
public WaterMarkRenderer(boolean isLicenseTrial)
{
m_licenseTrial = isLicenseTrial;
}
@Override
public byte getType()
{
// no idea what this does
return RenderableTypeEnum.SVG.getValue();
}
@Override
public byte getImageType()
{
// no idea what this does
return ImageTypeEnum.UNKNOWN.getValue();
}
@Override
public Dimension2D getDimension() throws JRException
{
// A4 in pixel: 595x842
// this seems to override whatever is configured in jasperreports studio
return new Dimension(595 - 2 * 40, 700);
}
@Override
public byte[] getImageData() throws JRException
{
// no idea what this does
return new byte[0];
}
@Override
public void render(Graphics2D g2, Rectangle2D rectangle) throws JRException
{
if(m_licenseTrial)
{
AffineTransform originalTransform = g2.getTransform();
// just for debugging
g2.setColor(Color.BLUE);
g2.draw(rectangle);
g2.translate(rectangle.getX() + 100, rectangle.getMaxY());
g2.rotate(-55 * Math.PI / 180);
Font font = new Font("Arial", Font.PLAIN, 120);
Shape shape = font.createGlyphVector(g2.getFontRenderContext(), "Trial License").getOutline();
g2.setColor(new Color(255, 0, 0, 100));
g2.setStroke(new BasicStroke(1));
g2.draw(shape);
g2.setTransform(originalTransform);
}
}
}
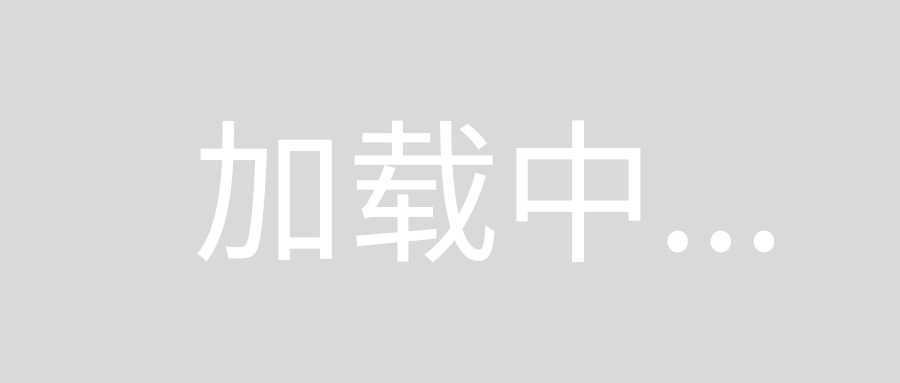
And the result:
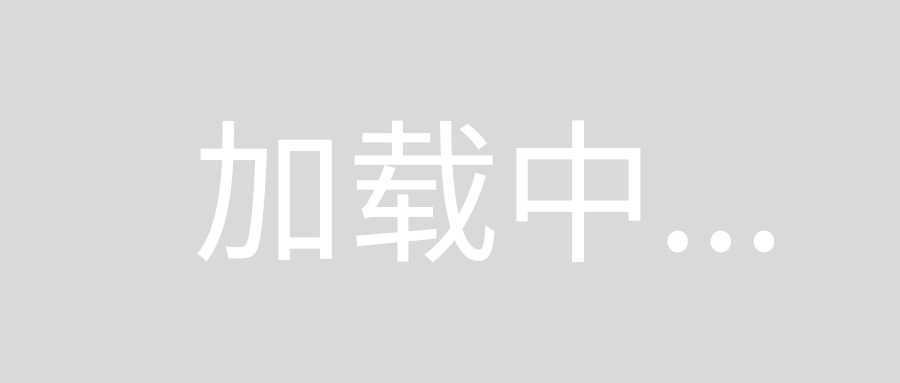
You can use iText to read the pdf you created using jasper reports. Either read the pdf as byteArray. Then use this using itext PdfReader
byte [] dataArray = JasperExportManager.exportReportToPdf(jasperPrint);
PdfReader pdfReader = new PdfReader(dataArray);
or read the pdf from a location if it is already written
JasperExportManager.exportReportToPdfFile(jasperPrint,pdfFileLocation);
PdfReader pdfReader = new PdfReader(pdfFileLocation);
I hope the following snippet helps from where I am reading the file and adding a watermark, deleting the existing file and writing a new one.
ByteArrayOutputStream baos = new ByteArrayOutputStream();
BaseFont bf = null;
PdfBoolean pdfBoolean_YES = new PdfBoolean(true);
PdfReader pdfReader = new PdfReader(pdfFileLocation);
PdfStamper pdfStamper = new PdfStamper(pdfReader, baos);
PdfContentByte contentunder = pdfStamper.getUnderContent(1);
contentunder.saveState();
contentunder.setColorFill(new Color(200, 200, 200));
contentunder.beginText();
bf = BaseFont.createFont(BaseFont.TIMES_ROMAN, BaseFont.CP1252, BaseFont.NOT_EMBEDDED);
contentunder.setFontAndSize(bf, 90);
contentunder.showTextAligned(Element.ALIGN_MIDDLE, " WaterMark Content", 200, 400, 45);
contentunder.endText();
contentunder.restoreState();
// We could stack those ViewerPreferences using '|' ... :)
pdfStamper.addViewerPreference(PdfName.HIDETOOLBAR, pdfBoolean_YES);
pdfStamper.addViewerPreference(PdfName.HIDEMENUBAR, pdfBoolean_YES);
//pdfStamper.addViewerPreference(PdfName.HIDEWINDOWUI, pdfBoolean_YES);
pdfReader.close();
pdfStamper.close();
//deleting existing file
FileUtil.delete(pdfFileLocation);
FileOutputStream fos = new FileOutputStream(pdfFileLocation);
baos.writeTo(fos);
fos.flush();
//close streams
baos.close();
fos.close();