I'm new to java and I'm trying to print an english ruler horizontally instead of vertically, any help is appreciated.
I tried to put a sample output but I need 10 reputation but it's very similar to an english ruler. Here is a link of a photo http://i.stack.imgur.com/y8beS.jpg
public class MyRuler
{
public static void main(String[] args)
{
drawRuler(3, 3);
}
public static void drawOneTick(int tickLength)
{
drawOneTick(tickLength, -1);
}
// draw one tick
public static void drawOneTick(int tickLength, int tickLabel)
{
for (int i = 0; i < tickLength; i++)
System.out.print("|\n");
if (tickLabel >= 0)
System.out.print(" " + tickLabel + "\n");
}
public static void drawTicks(int tickLength)
{ // draw ticks of given length
if (tickLength > 0)
{ // stop when length drops to 0
drawTicks(tickLength - 1); // recursively draw left ticks
drawOneTick(tickLength); // draw center tick
drawTicks(tickLength - 1); // recursively draw right ticks
}
}
public static void drawRuler(int nInches, int majorLength)
{ // draw ruler
drawOneTick(majorLength, 0); // draw tick 0 and its label
for (int i = 1; i <= nInches; i++)
{
drawTicks(majorLength - 1); // draw ticks for this inch
drawOneTick(majorLength, i); // draw tick i and its label
}
}
}
If you're not going for a a special formula for presentation (i.e. this will likely not be scaled to an actual ruler) and just want the output to print horizontally, removing all instances of \n
from your code makes it print in a line.
public static void drawOneTick(int tickLength, int tickLabel)
{
for (int i = 0; i < tickLength; i++)
System.out.print("|");
if (tickLabel >= 0)
System.out.print(" " + tickLabel);
}
Even after looking at your sample picture I wasn't sure what you wanted to print exactly so I decided to print the top part of the ruler bellow:
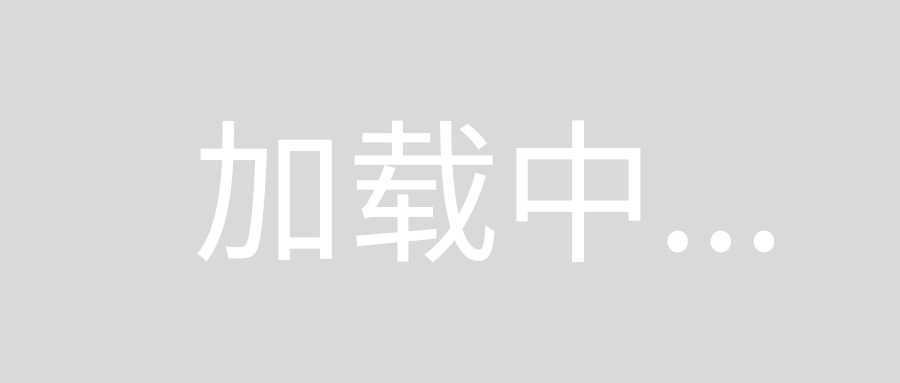
Considering I am European and I think the imperial system is weird and a major overkill, my ruler will measure in the metric system :) (centimeters and millimeters)
Ok, so the basic idea is to separate each row of ticks or labels as it's own String
like:
String1 = | | | | | | | | | | | | | | | | | | | | | | | ... // regular ticks
String2 = | | | ... // ticks to labels
String3 = 0 1 2 // labels
We build each string separately, then we combine them with a newline '\n'
character in between them so they will print properly. You have to also make sure the number of spaces is exact so that the strings align correctly.
Here is the code:
class MyRuler {
StringBuilder ticks = new StringBuilder();
StringBuilder ticksToLabels = new StringBuilder();
StringBuilder labels = new StringBuilder();
int millimetersPerCentimeter = 10;
String drawRuler(int centimeters) {
// append the first tick, tick to label, and label
ticks.append("| ");
ticksToLabels.append("| ");
labels.append(0);
for(int i = 0; i < centimeters; i++) {
for(int j = 0; j < millimetersPerCentimeter; j++) {
if(j == millimetersPerCentimeter - 1) {
ticksToLabels.append("| ");
labels.append(" " + (i + 1));
} else {
ticksToLabels.append(" ");
labels.append(" ");
}
ticks.append("| ");
}
}
ticks.append("\n" + ticksToLabels.toString() + "\n" + labels.toString());
return ticks.toString();
}
public static void main(String[] args) {
MyRuler ruler = new MyRuler();
System.out.println(ruler.drawRuler(5));
}
}
Output:
| | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | |
| | | | | |
0 1 2 3 4 5