I am developing an app in which I have to open a card like this video
https://www.youtube.com/watch?v=srnRuhFvYl0&feature=youtu.be
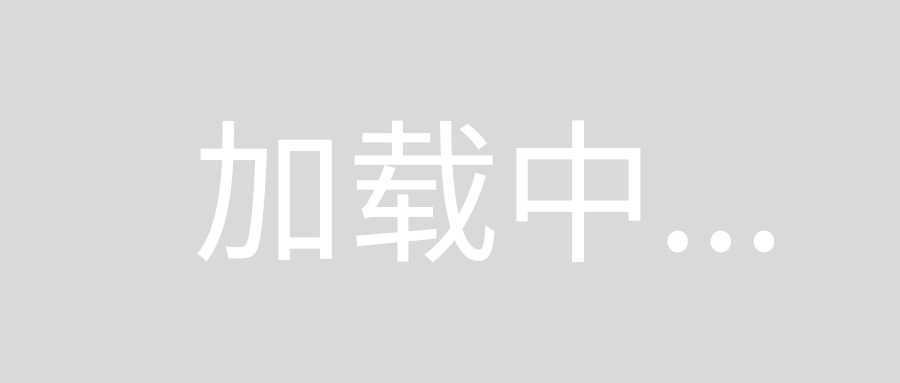
I googled but did not find any code for this functionality.
Greeting card has four sides. I need to view every side of greeting card either by Animation or UIGesturerecognizer when tapped or touched like in Just Wink App.
Any one suggest ideas or sample code will be appreciated.
"Thanks in Advance"
I have written this code for you.
*Put this in .pch file
define DEGREES_TO_RADIANS(d) (d * M_PI / 180)
and don't forget to add and import QuartzCore framework*
#import "MyViewController.h"
#import <QuartzCore/QuartzCore.h>
@interface MyViewController (){
CATransform3D intialTransform;
CATransform3D middelTransform;
CATransform3D finalTransform;
}
@property(nonatomic,strong) UIView *myView1;
@property(nonatomic,strong) UIView *myView2;
@end
@implementation MyViewController
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
UIButton *myButton =[[UIButton alloc]initWithFrame:CGRectMake(300, 100, 120, 50)];
[myButton addTarget:self action:@selector(unfoldGreeting:) forControlEvents:UIControlEventTouchDown];
[myButton setTitle:@"Unfold" forState:UIControlStateNormal];
[myButton setBackgroundColor:[UIColor blackColor]];
[self.view addSubview:myButton];
_myView1 = [[UIView alloc]initWithFrame:CGRectMake(400, 200, 200, 300)];
[_myView1 setBackgroundColor:[UIColor redColor]];
[self.view addSubview:_myView1];
UILabel *myLabel = [[UILabel alloc]initWithFrame:CGRectMake(10, 150, 100, 100)];
[myLabel setText:@"Happy Birthday"];
[myLabel setFont:[UIFont fontWithName:@"Arial" size:25]];
[myLabel setTextColor:[UIColor whiteColor]];
[myLabel sizeToFit];
[_myView1 addSubview:myLabel];
intialTransform = CATransform3DIdentity;
intialTransform.m34 = 1.0 / -500;
intialTransform = CATransform3DRotate(intialTransform, DEGREES_TO_RADIANS(70), 1.0f, 0.0f, 0.0f);
_myView1.layer.transform = intialTransform;
_myView2 = [[UIView alloc]initWithFrame:CGRectMake(400, 200, 200, 300)];
[_myView2 setBackgroundColor:[UIColor purpleColor]];
[self.view addSubview:_myView2];
_myView2.layer.transform = intialTransform;
middelTransform = CATransform3DIdentity;
middelTransform.m34 = 1.0 / -500;
middelTransform = CATransform3DRotate(middelTransform, DEGREES_TO_RADIANS(70), 1.0f, 0.0f, 0.0f);
middelTransform = CATransform3DRotate(middelTransform, DEGREES_TO_RADIANS(-100), 0.0f, 1.0f, 0.0f);
middelTransform = CATransform3DTranslate(middelTransform,116, 0,100);
finalTransform = CATransform3DIdentity;
finalTransform.m34 = 1.0 / -500;
finalTransform = CATransform3DRotate(finalTransform, DEGREES_TO_RADIANS(70), 1.0f, 0.0f, 0.0f);
finalTransform = CATransform3DRotate(finalTransform, DEGREES_TO_RADIANS(-170), 0.0f, 1.0f, 0.0f);
finalTransform = CATransform3DTranslate(finalTransform,198, 0,16);
}
-(void)unfoldGreeting:(UIButton *)iSender{
[UIView animateWithDuration:1.0f animations:^{
_myView2.layer.transform = middelTransform;
} completion:^ (BOOL iFinished) {
[UIView animateWithDuration:1.0f animations:^{
_myView2.layer.transform = finalTransform;
}completion:nil];
}];
}
Hope this will help you.