How we can setup ScrollView
vertically & horizontally? I tried the below code, but it didn't work.
<ScrollView
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:background="@color/red"
android:scrollbarFadeDuration="1000"
android:scrollbarSize="12dip" >
<HorizontalScrollView
android:id="@+id/horizontalScrollView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TableLayout
android:id="@+id/tableLayout1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:collapseColumns="2"
android:stretchColumns="1" >
</TableLayout>
</HorizontalScrollView>
<ScrollView >
</ScrollView>
Here is all my code : http://pastebin.com/ysRhLMyt
Current screen :
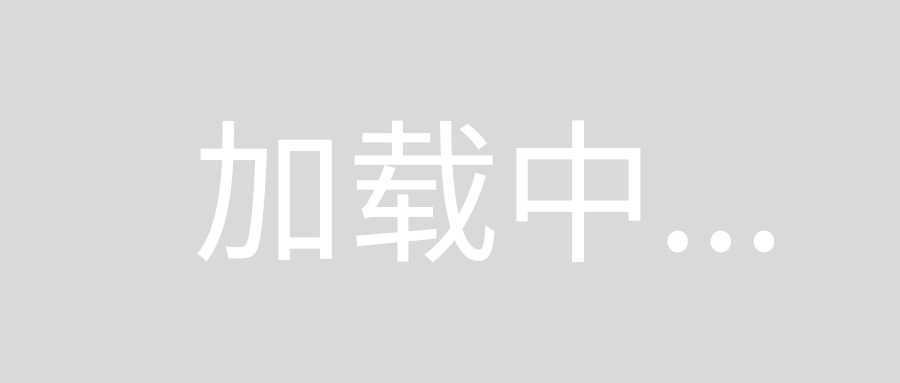
I want to display scroll bar always.
Try,
Set the android:scrollbarFadeDuration="0"
OR
ScrollView1.setScrollbarFadingEnabled(false);
OR
android:scrollbarFadeDuration="0" and
android:scrollbarAlwaysDrawVerticalTrack="true" for vertical
android:scrollbarAlwaysDrawHorizontalTrack="true" for horizontal
And one more thing,
Remember, the ScrollView can have only one child control, so we can make a container (Linear, relative, Table Layouts) the child of the ScrollView and put all the controls inside this child.
For reference: http://android-pro.blogspot.com/2010/02/android-scrollview.html
Nested scroll views doesn't work. It's related to scroll view touch handling: top level view always consume all touch events. You have to write you own custom scroll view.
Below code which makes horizontal and vertical scroll view,
To see its effect first define a area around 200x 200 dp and paste this code inside it.
The View will scrolling horizontally as well as vertically.
<ScrollView
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:scrollbarSize="10dp"
android:scrollbars="vertical" >
<HorizontalScrollView
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical" >
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal" >
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical" >
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
<Button
android:layout_width="300dp"
android:layout_height="100dp" />
</LinearLayout>
</LinearLayout>
</HorizontalScrollView>
Try using the android:orientation
attribute. This can be used for horizontal or vertical: android:orientation="horizontal"
or android:orientation="vertical"
.
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:scrollbarFadeDuration="1000"
android:scrollbarSize="12dip"
android:background="@color/red"
android:orientation="horizontal">
<TableLayout android:layout_width="match_parent"
android:layout_marginTop="10dp"
android:id="@+id/tableLayout1"
android:layout_height="wrap_content"
android:stretchColumns="1"
android:collapseColumns="2">
</TableLayout>
</ScrollView>