I set the border white and radius for my ImageView
. But in 4 corner of the ImageView
, some dark line appear.
Here is the code I set the color for my ImageView
self.image.layer.cornerRadius = 10;
self.image.layer.borderWidth = 3;
self.image.layer.borderColor = [[UIColor whiteColor] CGColor];
self.image.layer.masksToBounds = YES;
This is a small demo project. My storyboard only contains the ImageView
, and I don't set any constraint for my ImageView
.
I don't know why this happened, it have tested on simulator and real device but it give a same error
Here is demo project: (it's very simple) https://drive.google.com/file/d/0B_poNaia6t8kT0JLSFJleGxEcmc/view?usp=sharing
Update
Some people give a solution is change the color of border color from whiteColor
to clearColor
. Of course it will make 4 lines disappear. But if I using clearColor
, I don't need to add border to my ImageView
.
I need border white for some reason
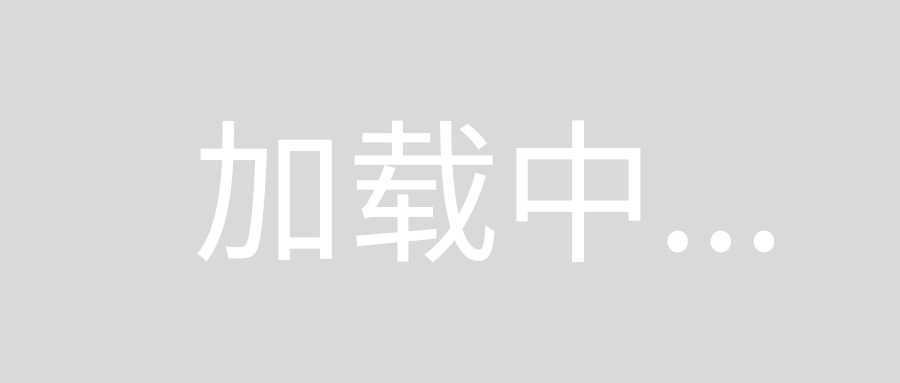
Updated code
I tried your code actually your image size is big initially I resized the image based on original Image size
UIImage *myIcon = [self imageWithImage:[UIImage imageNamed:@"abc.jpg"] scaledToSize:CGSizeMake(400, 400)];
self.image.image = myIcon;
sometimes corner radius does not work properly so I used UIBezierPath
for this concept
UIBezierPath *maskPath;
maskPath = [UIBezierPath bezierPathWithRoundedRect:self.image.bounds byRoundingCorners:(UIRectCornerTopLeft | UIRectCornerTopRight | UIRectCornerBottomLeft | UIRectCornerBottomRight) cornerRadii:CGSizeMake(10.0, 10.0)];
CAShapeLayer *maskLayer = [[CAShapeLayer alloc] init];
maskLayer.frame = self.view.bounds;
maskLayer.path = maskPath.CGPath;
self.image.layer.mask = maskLayer;
for border color and width use this
swift 3
let maskPath = UIBezierPath(roundedRect: imageView.bounds, byRoundingCorners: ([.topLeft, .topRight, .bottomLeft, .bottomRight]), cornerRadii: CGSize(width: 10.0, height: 10.0))
let borderShape = CAShapeLayer()
borderShape.frame = self.imageView.bounds
borderShape.path = maskPath.cgPath
borderShape.strokeColor = UIColor.white.cgColor
borderShape.fillColor = nil
borderShape.lineWidth = 3
self.imageView.layer.addSublayer(borderShape)
output
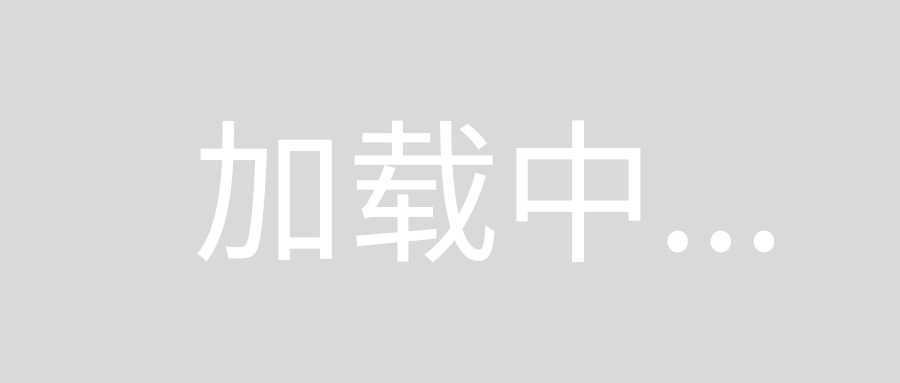
Update
CAShapeLayer* borderShape = [CAShapeLayer layer];
borderShape.frame = self.image.bounds;
borderShape.path = maskPath.CGPath;
borderShape.strokeColor = [UIColor whiteColor].CGColor;
borderShape.fillColor = nil;
borderShape.lineWidth = 3;
[self.image.layer addSublayer:borderShape];
Swift
var borderShape: CAShapeLayer = CAShapeLayer.layer
borderShape.frame = self.image.bounds
borderShape.path = maskPath.CGPath
borderShape.strokeColor = UIColor.whiteColor().CGColor
borderShape.fillColor = nil
borderShape.lineWidth = 3
self.image.layer.addSublayer(borderShape)
Output
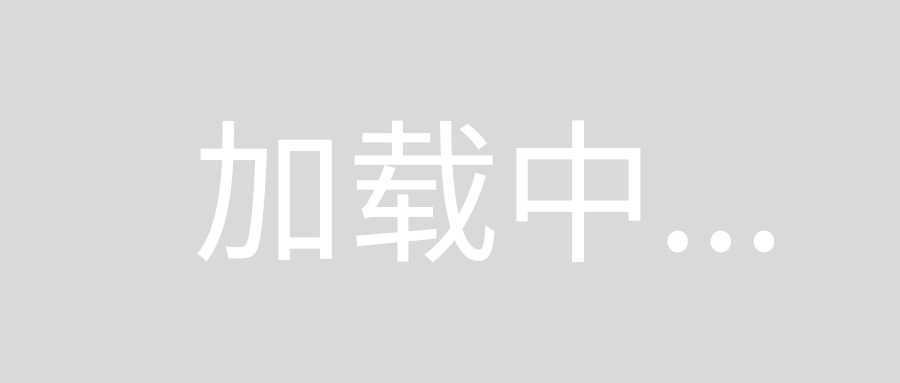
Code for whole project
Actually you have to use two layers.
self.image.clipsToBounds = YES;
UIBezierPath *maskPath = [UIBezierPath bezierPathWithRoundedRect:self.image.bounds byRoundingCorners:UIRectCornerAllCorners cornerRadii:CGSizeMake(48, 48)];
CAShapeLayer *maskLayer = [CAShapeLayer layer];
maskLayer.frame = self.image.bounds;
maskLayer.path = maskPath.CGPath;
maskLayer.strokeColor = [UIColor redColor].CGColor;
self.image.layer.mask = maskLayer;
CAShapeLayer* frameLayer = [CAShapeLayer layer];
frameLayer.frame = self.image.bounds;
frameLayer.path = maskPath.CGPath;
frameLayer.strokeColor = [UIColor whiteColor].CGColor;
frameLayer.fillColor = nil;
frameLayer.lineWidth = 20;
[self.image.layer addSublayer:frameLayer];
Try clip to bounds:
self.image.clipToBounds = YES
i tried this function written in imageview category:
- (void)setBorderWithRounCornersWithColor:(UIColor *)color{
self.layer.cornerRadius = 5.0f;
self.layer.masksToBounds = YES;
if(color){
self.layer.borderColor=color.CGColor;
self.layer.borderWidth=1.0f;
}
}
at time of using:
[self.imageView setBorderWithRounCornersWithColor:nil];
I check your code you can change this line its work for me
self.image.layer.borderColor = [[UIColor clearColor] CGColor];
when you add a border that add in inside the image border line and when you set rounded border that time your round corner has some transparent part so that part will display in corner side
just change the Color of border color
image1.layer.cornerRadius = 10;
image1.layer.borderWidth = 3;
image1.layer.borderColor = [[UIColor whiteColor] CGColor];
image1.layer.masksToBounds = YES;
image2.layer.cornerRadius = 10;
image2.layer.borderWidth = 3;
image2.layer.borderColor = [[UIColor clearColor] CGColor];
image2.layer.masksToBounds = YES;
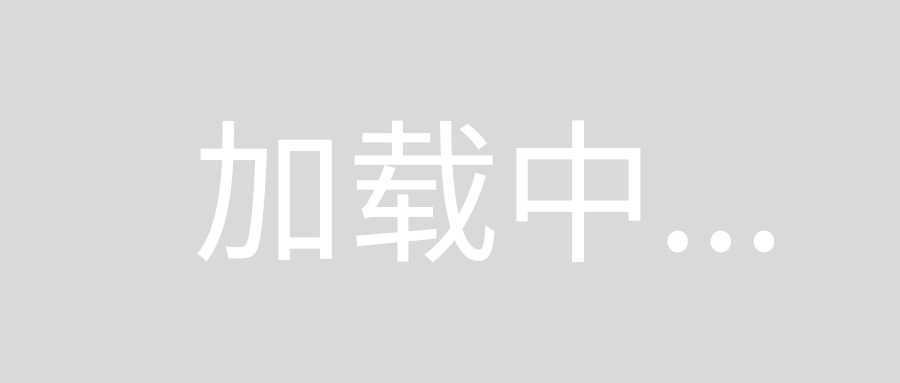