I need your help !
I work on a mobile development project, cross-platform. I use Visual Studio with Xamarin Forms. I have a SearchBar (Xamarin.Forms class), but, my problem is to include this into the NavigationBar.
Currently, I have a MasterDetailPage and I init this.Detail with new NavigationPage(new customPage()). I have a SearchBar renderer, too.
So, I need original NavigationBar (I want to keep the return button) but I would like to add my SearchBar inside.
I would be very grateful if you know of a way to do this especially to android.
Thank you in advance !
You need to create a custom NavigationRenderer
and insert your own UISearchBar
into the UIToolbar
or SearchView
into the ViewGroup
:
Xamarin Forms : Renderer Base Classes and Native Controls
As an example of inserting a iOS UISearchBar
into the nav bar via a custom NavigationRenderer
:
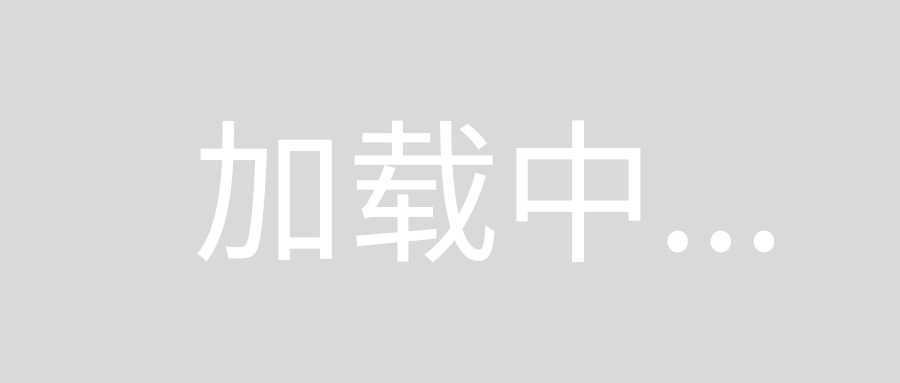
Note: In order to keep things decoupled, using the MessagingCenter
allows you to pump messages around without any hardcoding event dependancies within the custom renderer.
[assembly:ExportRenderer (typeof(NavigationPage), typeof(SeachBarNavigationRender))]
namespace Forms.Life.Cycle.iOS
{
public class SeachBarNavigationRender : NavigationRenderer
{
public SeachBarNavigationRender () : base()
{
}
public override void PushViewController (UIViewController viewController, bool animated)
{
base.PushViewController (viewController, animated);
List<UIBarButtonItem> toolbarItem = new List<UIBarButtonItem> ();
foreach (UIBarButtonItem barButtonItem in TopViewController.NavigationItem.RightBarButtonItems) {
if ( barButtonItem.Title.Contains( "search")) {
UISearchBar search = new UISearchBar (new CGRect (0, 0, 200, 25));
search.BackgroundColor = UIColor.LightGray;
search.SearchBarStyle = UISearchBarStyle.Prominent;
search.TextChanged += (object sender, UISearchBarTextChangedEventArgs e) => {
D.WriteLine(e.SearchText);
MessagingCenter.Send<object, string> (this, "SearchText", e.SearchText);
};
barButtonItem.CustomView = search;
}
toolbarItem.Add (barButtonItem);
}
TopViewController.NavigationItem.RightBarButtonItems = toolbarItem.ToArray ();
}
}
}